LAB RESTRICTIONS, PLEASE READ: - Do not add any imports, the ones that you need will be given to you. - Do not use recursion. - Do not use try-except statements, you should be able to anticipate or prevent any errors from happening at all! def longest_(s: str) -> str: """ Given a string , return the longest unique substring that occurs within . A unique substring is a substring within which DOES NOT have any repeating characters. As an example, "xd" is unique but "xxd" is not. If there are two equal length unique substrings within , return the one that starts first (i.e., begins at a smaller index). Note on grading for this question: You've already implemented a function that can generate all the substrings of a given string in lab 5. You can use that logic to help here, but it will be quite slow. For this question, PERFORMANCE WILL MATTER, and we will run long tests with up to 10,000,000 characters as an input. To get full credit, an input of 10,000,000 characters should return the correct answer within 60 seconds (the instructor solution takes about 2). Helpful tips: In order to get your function to run fast, consider using a dictionary to store the indexes of previously seen characters, from there, you can follow a set of rules based on each new character you see to determine the length of the longest unique substring seen so far. >>> longest_('aab') 'ab' """
LAB RESTRICTIONS, PLEASE READ:
- Do not add any imports, the ones that you need will be given to you.
- Do not use recursion.
- Do not use try-except statements, you should be able to anticipate
or prevent any errors from happening at all!
def longest_(s: str) -> str:
"""
Given a string <s>, return the longest unique substring that occurs within
<s>.
A unique substring is a substring within <s> which DOES NOT have any
repeating characters. As an example, "xd" is unique but "xxd" is not.
If there are two equal length unique substrings within <s>, return the one
that starts first (i.e., begins at a smaller index).
Note on grading for this question:
You've already implemented a function that can generate all the substrings
of a given string in lab 5. You can use that logic to help here, but it
will be quite slow.
For this question, PERFORMANCE WILL MATTER, and we will run long tests with
up to 10,000,000 characters as an input. To get full credit, an input of
10,000,000 characters should return the correct answer within 60 seconds
(the instructor solution takes about 2).
Helpful tips:
In order to get your function to run fast, consider using a dictionary to
store the indexes of previously seen characters, from there, you can
follow a set of rules based on each new character you see to determine
the length of the longest unique substring seen so far.
>>> longest_('aab')
'ab'
"""

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

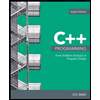
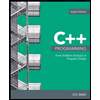