This question was rejected because lack of a text file, which I do not see a way to upload. I saved the first part of the file as an image because that is all I can upload. If that doesn't suffice, please advise how to include a text file. In C++, I would like some help improving or making a better password lookup function for a Hash Table class/program I made, The function: it is supposed to lookup a user password using their ID and name which I read in from a text file. The one I have only works for the first 10 records, which I highlighted below in the program.
This question was rejected because lack of a text file, which I do not see a way to upload. I saved the first part of the file as an image because that is all I can upload. If that doesn't suffice, please advise how to include a text file.
In C++, I would like some help improving or making a better password lookup function for a Hash Table class/program I made,
The function: it is supposed to lookup a user password using their ID and name which I read in from a text file. The one I have only works for the first 10 records, which I highlighted below in the program.
string lookupPassword (string inUserID, string inUserName){
string thePassword;
...
return thePassword;
}
Here is part of the program thus far:
// Hash.cpp
#include <iostream>
#include<string>
#include<iomanip>
#include<fstream>
using namespace std;
//node class
class nodeBST {
private: //user data
string userID;
string userName;
string userPW;
//BST left and right children
nodeBST* ptrLeftNode;
nodeBST* ptrRightNode;
public:
//constructor
nodeBST() { userID = " "; userName = " "; userPW = " "; ptrLeftNode = NULL; ptrRightNode = NULL; }
//get and set methods for ID, Name, PW
string getUserID() { return userID; }
void setUserID(string inUserID) { userID = inUserID; }
string getUserName() { return userName; }
void setUserName(string inUserName) { userName = inUserName; }
string getUserPW() { return userPW; }
void setUserPW(string inUserPW) { userPW = inUserPW; }
//get and set left and right children pointers
void setPtrLeftNode(nodeBST* inPtrLeftNode) { ptrLeftNode = inPtrLeftNode; }
nodeBST* getPtrLeftNode() { return ptrLeftNode; }
void setPtrRightNode(nodeBST* inPtrRightNode) { ptrRightNode = inPtrRightNode; }
nodeBST* getPtrRightNode() { return ptrRightNode; }
};
class myHash { //hash class
private:
//hash table with chaining - using BST's
nodeBST* myHashTable[10]; //10 BST root node headers
int recordCount = 0; //count initialization
public:
myHash() { //constructor
recordCount = 0;
for (int i = 0; i < 10; i++) { myHashTable[i] = NULL; }
} //loads 100 record text file into hash table
void loadHashTableFromFile(string inFileName) {
string inUserID;
string inUserName;
string inUserPW;
string aWord;
ifstream fin;
cout << "Reading in File for Hash Table" << endl;
fin.open(inFileName); //opens file
for (int i = 0; i < 100; i++) {
if (fin >> aWord) {
if (i % 3 == 0) {
inUserID = aWord;
}
else if (i % 3 == 1) {
inUserName = aWord;
}
else if (i % 3 == 2) {
inUserPW = aWord;
addRecord(inUserID, inUserName, inUserPW);
}
}
}
fin.close(); //closes file
}
string lookupPassword(string inUserId, string inUserName) { //******This is where I need help****
string thePassWord = "Goofy"; //initialize PW temp value
//gather user index
int index = myHashFunction(inUserId);
//root to hold index for user ID
nodeBST* root = myHashTable[index];
//set PW by looking up userPW through root
thePassWord = root->getUserPW();
return thePassWord;
}
int myHashFunction(string inUserID) {
//calculate array index and return index value 0 -9
int index = 0;
char aChar = inUserID[inUserID.length() - 1];
index = aChar - '0';
return index;
}
void addRecord(string inUserID, string inUserName, string inUserPW) {
int index = 0;
//calculate index = ID's last digit
index = myHashFunction(inUserID);
//declare new node and add to BST
nodeBST* newNode = new nodeBST;
//assign values to new node
newNode->setUserID(inUserID);
newNode->setUserName(inUserName);
newNode->setUserPW(inUserPW);
//check if root node occupied
if (myHashTable[index] == NULL) { //add to root
myHashTable[index] = newNode;
}
else {
//add to BST
//find location to add new node
recuriseInsertRecord(myHashTable[index], newNode);
}
}
I have print and traversal functions but I cut them out. Thank You for your time!
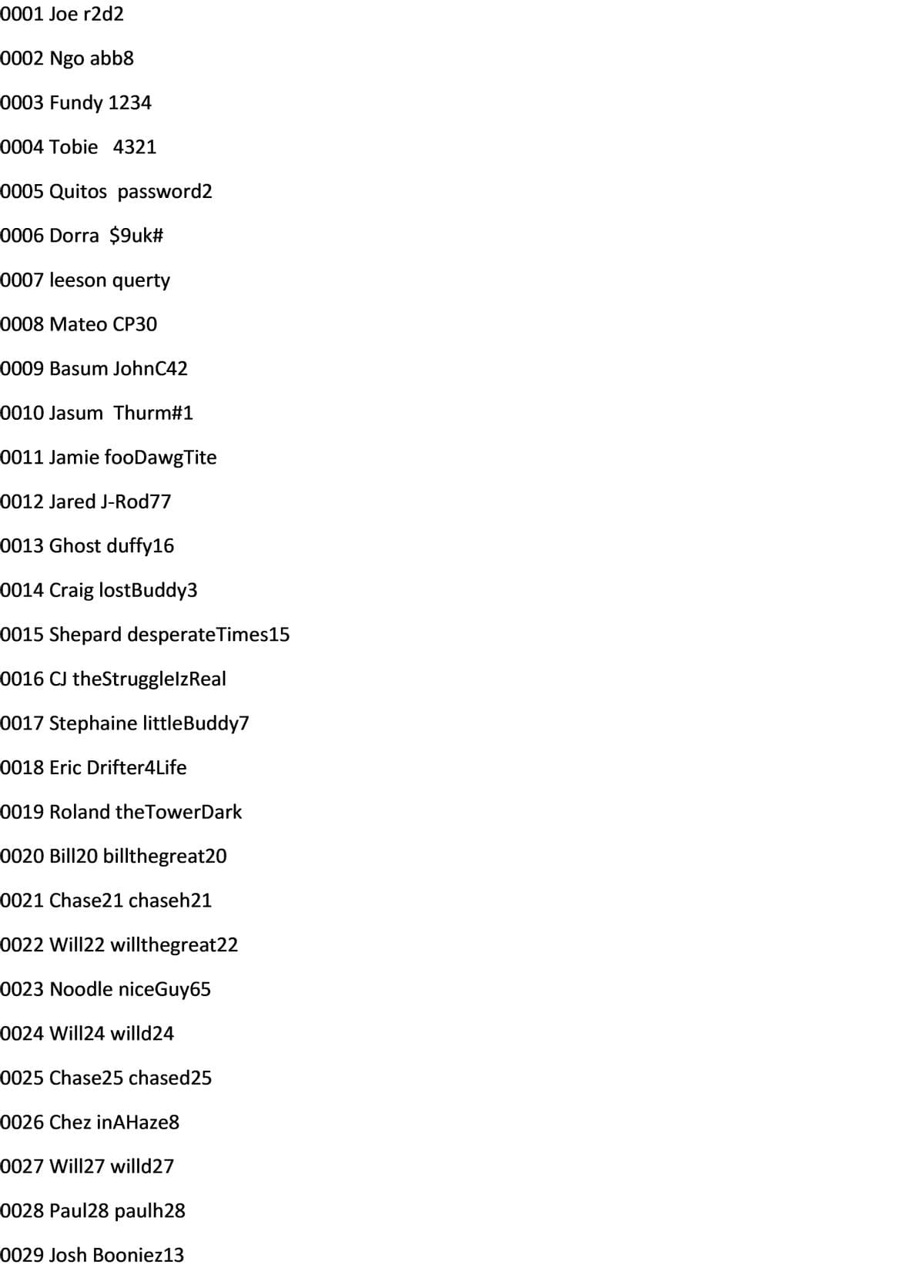

Step by step
Solved in 2 steps

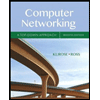
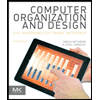
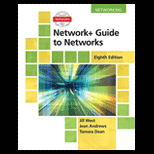
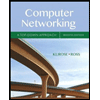
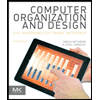
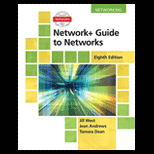
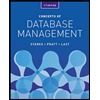
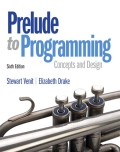
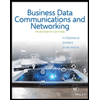