LinkedAssemble Editor 1 node* assemble(vectororange->banana->pear-NULL Test Submit
LinkedAssemble Editor 1 node* assemble(vectororange->banana->pear-NULL Test Submit
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Thank you!
C++ please
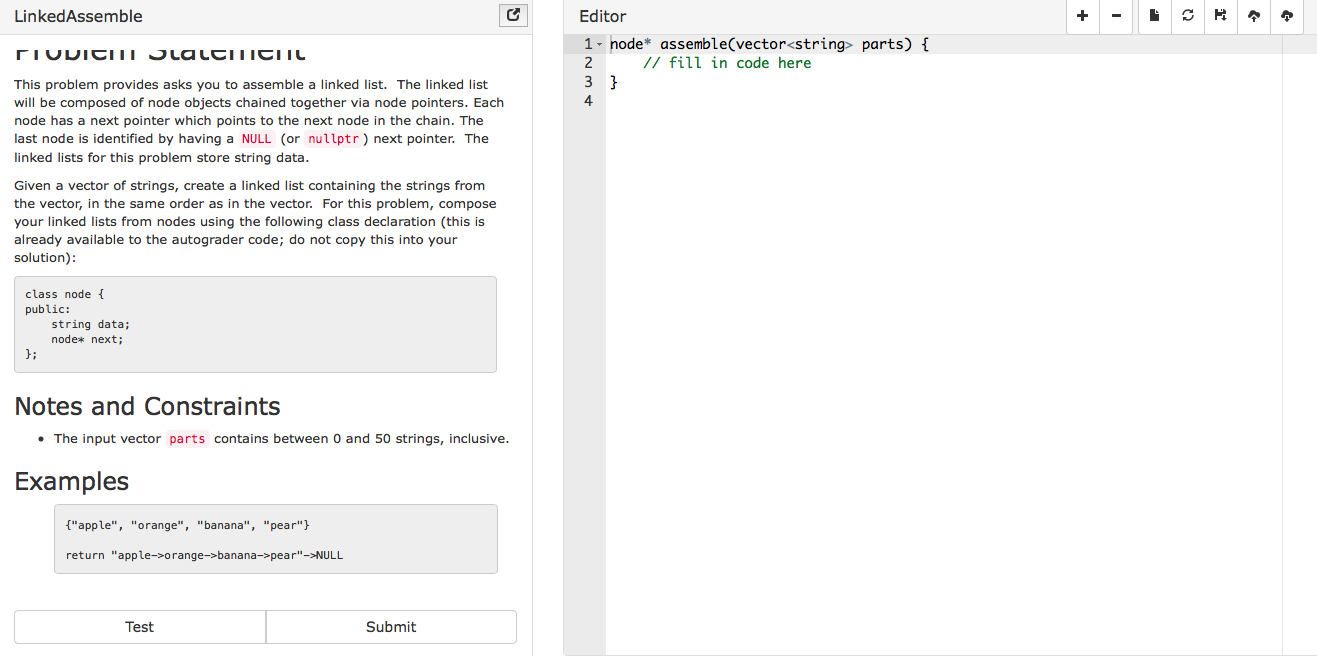
Transcribed Image Text:LinkedAssemble
Editor
1 node* assemble(vector<string parts)
//fill in code here
This problem provides asks you to assemble a linked list. The linked list
will be composed of node objects chained together via node pointers. Each
node has a next pointer which points to the next node in the chain. The
last node is identified by having a NULL (or nullptr) next pointer. The
linked lists for this problem store string data
4
Given a vector of strings, create a linked list containing the strings from
the vector, in the same order as in the vector. For this problem, compose
your linked lists from nodes using the following class declaration (this is
already available to the autograder code; do not copy this into your
solution)
class node
public:
string data;
node* next;
d:
Notes and Constraints
. The input vector parts contains between 0 and 50 strings, inclusive
Examples
["apple", "orange", "banana", "pear"
return "apple->orange->banana->pear-NULL
Test
Submit
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
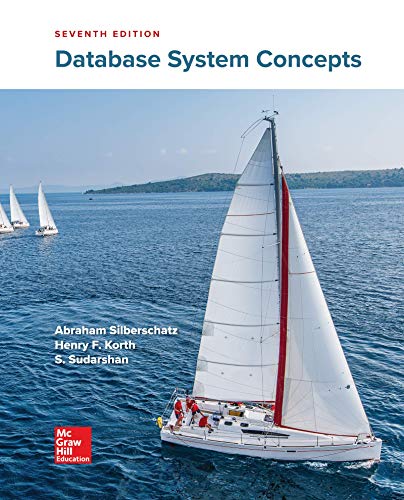
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
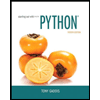
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
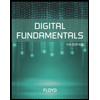
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
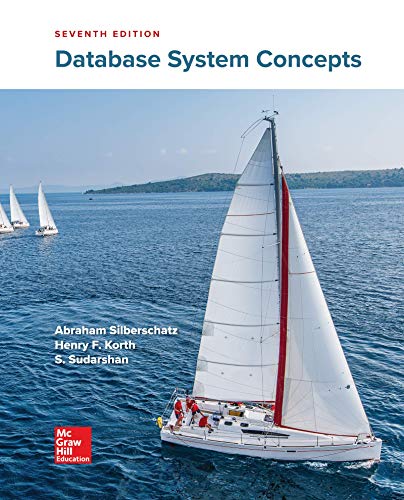
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
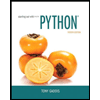
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
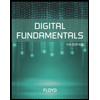
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
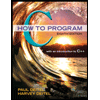
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
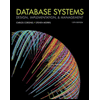
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
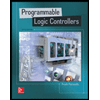
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education