Modify the bracketsBalance function so that the caller can supply the brackets to match as arguments to this function. The second argument should be a list of beginning brackets, and the third argument should be a list of ending brackets. The pairs of brackets at each position in the two lists should match; that is, position 0 in the two lists might have [ and], respectively. You should be able to modify the code for the function so that it does not reference literal bracket symbols, but just uses the list arguments. (Hint: The method index returns the position of an item in a list.) the bracketsBalance function: def bracketsBalance(exp): """exp is a string that represents the expression""" stk = LinkedStack() for ch in exp: if ch in [’[’, ’(’]: stk.push(ch) elif ch in [’]’, ’)’]: if stk.isEmpty(): # Create a new stack # Scan across the expression # Push an opening bracket # Process a closing bracket # Not balanced return False chFromStack = stk.pop() # Brackets must be of same type and match up if ch == ’]’ and chFromStack != ’[’ or \ ch == ’)’ and chFromStack != ’(’: return False Use this Python template: from collections import deque class Stack: ''' TODO: Remove the "pass" statements and implement each method Add any methods if necesssary DON'T use any builtin stack class to store your items ''' def __init__(self): # Constructor function pass def isEmpty(self): # Returns True if the stack is empty or False otherwise pass def len(self): # Returns the number of items in the stack pass def peek(self): # Returns the item at the top of the stack pass def push(self, item): # Adds item to the top of the stack pass def pop(self): # Removes and returns the item at the top of the stack pass def bracketsBalance(input_str,opening_list,closing_list): isBalanced = True stk=Stack() #TODO: Your work here # Return if input_str is balanced or not return isBalanced if __name__ == "__main__": my_str = "([{))}" opening_list=['(', '[', '{'] closing_list=[')', ')', '}'] print(bracketsBalance(my_str,opening_list,closing_list)) # Correct Output: False #TODO (optional): Your testing code here
4. Modify the bracketsBalance function so that the caller can supply the brackets to match as arguments to this function. The second argument should be a list of beginning brackets, and the third argument should be a list of ending brackets. The pairs of brackets at each position in the two lists should match; that is, position 0 in the two lists might have [ and], respectively. You should be able to modify the code for the function so that it does not reference literal bracket symbols, but just uses the list arguments. (Hint: The method index returns the position of an item in a list.)
the bracketsBalance function:
def bracketsBalance(exp):
"""exp is a string that represents the expression"""
stk = LinkedStack() for ch in exp:
if ch in [’[’, ’(’]: stk.push(ch)
elif ch in [’]’, ’)’]: if stk.isEmpty():
# Create a new stack # Scan across the expression
# Push an opening bracket
# Process a closing bracket
# Not balanced
return False
chFromStack = stk.pop()
# Brackets must be of same type and match up if ch == ’]’ and chFromStack != ’[’ or \
ch == ’)’ and chFromStack != ’(’:
return False
Use this Python template:
from collections import deque
class Stack:
'''
TODO: Remove the "pass" statements and implement each method
Add any methods if necesssary
DON'T use any builtin stack class to store your items
'''
def __init__(self): # Constructor function
pass
def isEmpty(self): # Returns True if the stack is empty or False otherwise
pass
def len(self): # Returns the number of items in the stack
pass
def peek(self): # Returns the item at the top of the stack
pass
def push(self, item): # Adds item to the top of the stack
pass
def pop(self): # Removes and returns the item at the top of the stack
pass
def bracketsBalance(input_str,opening_list,closing_list):
isBalanced = True
stk=Stack()
#TODO: Your work here
# Return if input_str is balanced or not
return isBalanced
if __name__ == "__main__":
my_str = "([{))}"
opening_list=['(', '[', '{']
closing_list=[')', ')', '}']
print(bracketsBalance(my_str,opening_list,closing_list)) # Correct Output:
False
#TODO (optional): Your testing code here

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

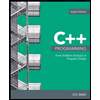
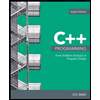