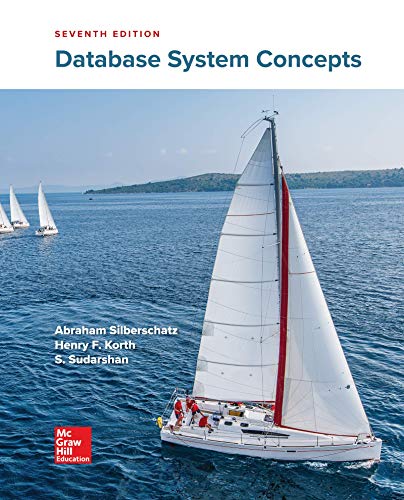
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Create a class Queue and a Main to test it. This Queue will be implemented using an array and will hold int values.
The array should start with a size of two. When needed, you should grow the array by doubling it in size. You will never shrink the array.
Use a basic array of type int (do not use ArrayList or anything like that).
Your Queue should have the following public methods:
- public void enqueue(int n)
- O(c) or O(n) when growing the array
- public int dequeue()
- O(n)
- public int peek()
- O(c)
- public int size()
- O(c)
- public boolean isEmpty()
- O(c)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Consider the IntArrayBag collection class that we discussed in class, Draw the data array after executing the following code and show the values that are stored in each cell of the array. Show the array four times at the points labelled A, B, C, and D IntArrayBag bag1 = new IntArrayBag(5); bag1.add(10); bag1.add(5); bag1.add(20); bag1.add(13); //Point A bag1.remove(5); //Point B bag1.add(8); bag1.remove(10); //Point C bag1.add(30); //Point D Exercise 4: search methodarrow_forwardDefine a class Car as follows:class Car { public String make; public String model; public int mpg;// Miles per gallon}a) Implement a comparator called CompareCarsByMakeThenModel that can be passed as an argument to the quicksort method from the lecture notes. CompareCarsByMakeThenModel should return a value that will cause quicksort to sort an array of cars in ascending order (from smallest to largest) by make and, when two cars have the same make, in ascending order by model.b) Implement a comparator called CompareCarsByDescendingMPG that can be passed as an argument to the quicksort method from the lecture notes. CompareCarsByDescendingMPG should return a value that will cause quicksort to sort an array of cars in descending order (from largest to smallest) by mpg.c) Implement a comparator called CompareCarsByMakeThenDescendingMPG that can be passed as an argument to the quicksort method from the lecture notes.…arrow_forwardjava Create a static method that: is called removeAll returns ArrayList<String> takes two parameters: an ArrayList of Strings called wordList, and a String called targetWord This method should go through every element of wordList and remove every instance of targetWord from the ArrayList. Example: ArrayList<String> wordList = new ArrayList<String>(Arrays.asList("hi","hey","hi","yo")); removeAll(wordList,"hi"); wordList: ["hey","yo"] public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); String target = in.next(); ArrayList<String> list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.next()); } System.out.println(removeAll(list, target)); } }arrow_forward
- using arrays or Arraylist in java language don't use Scanner class Write the method named examScore().* * The "key" list contains the correct answers * to an exam, like ["a", "a", "b", "b"]. The "answers" * list contains a student's answers, with "?" representing * a question left blank. The two lists are not empty and * are the same length. Return the score for this list * of answers, giving +4 for each correct answer, -1 for * each incorrect answer, and +0 for each blank answer.* * Examples:* examScore(["a", "a", "b", "b"], ["a", "c", "b", "c"]) returns 6* examScore(["a", "a", "b", "b"], ["a", "a", "b", "c"]) returns 11* examScore(["a", "a", "b", "b"], ["a", "a", "b", "b"]) returns 16* * @param key a list of correct answers to an exam.* @param answers the student's answers to the exam.* @return the score as described above.arrow_forwardImplement the following method where: 1. String s is a given string 2. myArray is an array list that contains strings 3. The method findExactString will take the string s and the arraylist myArray and return an arraylist that contains the indices of all strings in myArray that matches the string s. If the reference to the string is null and the reference to the string inside the array is also null, string s is considered to be a equal to the string in arraylist. public static ArrayList findExactString(String s, Arraylist myArray) { return null;arrow_forwardWrite a method that takes two arrays as parameters. It should return a new array where each position has the sum of the two corresponding positions in the two incoming arrays. The returned array should be as long as the longer of the two passed in arrays.arrow_forward
- Develop a class ResizingArrayQueueOfStrings that implements the queueabstraction with a fixed-size array, and then extend your implementation to use arrayresizing to remove the size restriction.Develop a class ResizingArrayQueueOfStrings that implements the queueabstraction with a fixed-size array, and then extend your implementation to use arrayresizing to remove the size restriction.arrow_forwardIn java Write a method public static ArrayList<Integer> append(ArrayList<Integer> a, ArrayList<Integer> b) that appends one array list after another. Ex: a = 1 2 3 4 5, b = 6 7 8 9 10 then append returns 1 2 3 4 5 6 7 8 9 10arrow_forwardin Java: this {ArrayList<T>} is a type of class called ....... class that offers some useful methods for working with arrays these methods include (mention four methods):arrow_forward
- 1. Write a program that calls a method that takes an integer array as a parameter (you can initialize an array in the main method) and find the minimum value from the list and returns it. Partial program is given below. public class Min{ public static void main(String[] args){ int arr= System.out.print(minimum(arr)); }arrow_forwardWrite a java program to create prices of Shopping Cart items that provides a menu: 1: Create a new price for each item in the Cart list 2: Print the price of a particular item 0: Exit Enter your choice: Define an array: ShoppingCart which stores prices of items. Define another array: ItemsNames of the same size that stores names of such items. Assume that there are 10 items. When the user presses 1, the program call a method that creates a new price for each item in the array using random numbers between 1 and 1000 AED [not inclusive]. If the user presses 2, the program call a method that prints the name of the requested item and its price. Use switch case statement for choosing among the menu options. Make a method to display the menu to give the user the possibility to use the menu as much as he/she wants.arrow_forwardPlease use java only and public int method. Show the steps with the pictures also. In this assignment, you will implement a class calledArrayAndArrayList. This class includes some interesting methods for working with Arrays and ArrayLists. For example, the ArrayAndArrayList class has a “findMax” method which finds and returns the max number in a given array. For a defined array: int[] array = {1, 3, 5, 7, 9}, calling findMax(array) will return 9. There are 4 methods that need to be implemented in the A rrayAndArrayList class: ● howMany(int[] array, int element) - Counts the number of occurrences of the given element in the given array. ● findMax(int[] array) - Finds the max number in the given array. ● maxArray(int[] array) - Keeps track of every occurrence of the max number in the given array. ● swapZero(int[] array) - Puts all of the zeros in the given array, at the end of the given array. Each method has been defined for you, but without the code. See the javadoc for each…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
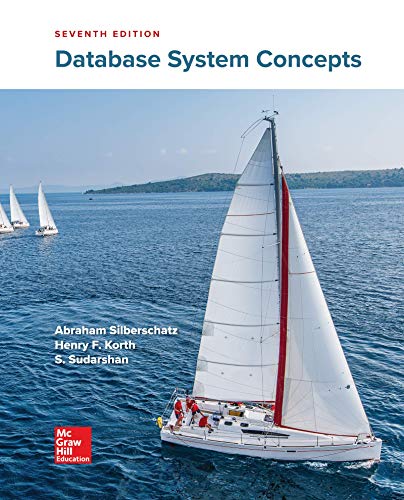
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
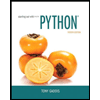
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
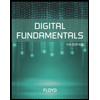
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
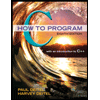
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
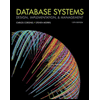
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
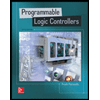
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education