My data structure doesn't seem to work, please help me Data.h: #pragma once class List { private: typedef struct node{ int data; node* next; }* nodePtr; nodePtr head; nodePtr curr; nodePtr temp; public: List(); void AddNode(int addData); void DeleteNode(int delData); void PrintList(); }; Implementation.cpp: #include #include "Data.h" using namespace std; List::List(){ head = NULL; curr = NULL; temp = NULL; } void List::AddNode(int addData){ nodePtr n = new node; n->next = NULL; n->next = addData; if(head !=NULL){ curr = head; while(curr->next !=NULL){ curr = curr->next; } curr->next = n; } else { head = n; } } void List::DeleteNode (int delData){ nodePtr delPtr = NULL; temp = head; curr = head; while (curr != NULL && curr->data != delData){ temp = curr; curr = curr->next; } if (curr == NULL){ cout << delData << "is not on the list\n"; delete delPtr; } else{ delPtr = curr; curr = curr->next; temp->next = curr; if (delPtr == head){ head = head->next; temp = NULL; } delete delPtr; cout << "The value" <data << endl; curr = curr->next; } } Main.cpp: #include #include "Data.h" using namespace std; int main() { List Paul; Paul.AddNode(3); Paul.AddNode(5); Paul.AddNode(7); Paul.PrintList(); Paul.DeleteNode(5); Paul.PrintList(); }
My data structure doesn't seem to work, please help me
Data.h:
#pragma once
class List
{
private:
typedef struct node{
int data;
node* next;
}* nodePtr;
nodePtr head;
nodePtr curr;
nodePtr temp;
public:
List();
void AddNode(int addData);
void DeleteNode(int delData);
void PrintList();
};
Implementation.cpp:
#include <iostream>
#include "Data.h"
using namespace std;
List::List(){
head = NULL;
curr = NULL;
temp = NULL;
}
void List::AddNode(int addData){
nodePtr n = new node;
n->next = NULL;
n->next = addData;
if(head !=NULL){
curr = head;
while(curr->next !=NULL){
curr = curr->next;
}
curr->next = n;
}
else
{
head = n;
}
}
void List::DeleteNode (int delData){
nodePtr delPtr = NULL;
temp = head;
curr = head;
while (curr != NULL && curr->data != delData){
temp = curr;
curr = curr->next;
}
if (curr == NULL){
cout << delData << "is not on the list\n";
delete delPtr;
}
else{
delPtr = curr;
curr = curr->next;
temp->next = curr;
if (delPtr == head){
head = head->next;
temp = NULL;
}
delete delPtr;
cout << "The value" <<delData <<"was deleted\n";
}
}
void List::PrintList(){
curr = head;
while (curr !=NULL){
cout << curr->data << endl;
curr = curr->next;
}
}
Main.cpp:
#include <iostream>
#include "Data.h"
using namespace std;
int main()
{
List Paul;
Paul.AddNode(3);
Paul.AddNode(5);
Paul.AddNode(7);
Paul.PrintList();
Paul.DeleteNode(5);
Paul.PrintList();
}

Step by step
Solved in 3 steps with 2 images

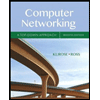
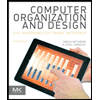
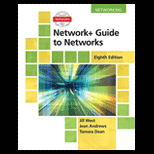
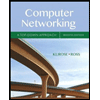
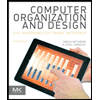
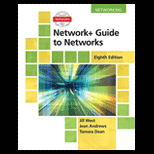
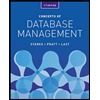
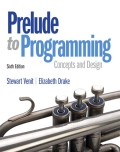
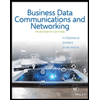