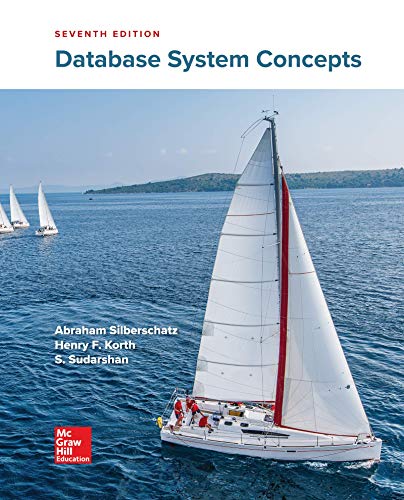
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
![You are to implement an interactive dynamic single Linked List structure. This List will be based on
the node definition given on the next page. Since you already know how to create classes, this
should be a logical extension. Your goal is to create a Linked List class which contains "node"
elements. Your program should contain the ability to dynamically (meaning the user can select what
each choice at anytime):
1. Insert at head (new node at head)
2. Insert at tail (new node placed at the end of the list)
3. Print (prints out the contents of the list in order)
4. Remove from head (remove first node) [watch special case]]
5. Remove from tail (remove last node) [watch special cases]
6. Find a target value (an isThere routine) (tells you if the target is in the list)
7. Give total # of occurrences of a specified value (how many items of target are in the list)
8. Give a total # of nodes (total items in list)
This program will track integers from the user. Be aware that some of these methods above will
force you to create other routines/methods. You are coding dynamic structures - code accordingly.
See page 2 for moro specificity on the methods.
Deliverables:
1) Clearly documented, professionally written source code (in a running form)
2) 3 runs showing all the user menu items. (output) (screenshots are fine)
3) Grading is based on:
a) Meets all requirements (80%)
b) Quality of sourco code (comments, logic, pre/post conditions, etc.) (20%)
For extra credit, you may do the following: (will require research on your own)
[DO NOT DO THIS UNLESS YOUR PROGRAM ALREADY FULLY WORKS]
1) Upgrade the "insert" method to allow for a "variable location insert". (this isn't easy) (5%)
2) Attempt to make 1 or more of the methods recursive. (5%)](https://content.bartleby.com/qna-images/question/341d06cc-fc5c-4ccd-b5b0-8d9552e9adb4/7f588e54-a19c-409b-a338-df5fdc702fcd/p0sdjw_thumbnail.jpeg)
Transcribed Image Text:You are to implement an interactive dynamic single Linked List structure. This List will be based on
the node definition given on the next page. Since you already know how to create classes, this
should be a logical extension. Your goal is to create a Linked List class which contains "node"
elements. Your program should contain the ability to dynamically (meaning the user can select what
each choice at anytime):
1. Insert at head (new node at head)
2. Insert at tail (new node placed at the end of the list)
3. Print (prints out the contents of the list in order)
4. Remove from head (remove first node) [watch special case]]
5. Remove from tail (remove last node) [watch special cases]
6. Find a target value (an isThere routine) (tells you if the target is in the list)
7. Give total # of occurrences of a specified value (how many items of target are in the list)
8. Give a total # of nodes (total items in list)
This program will track integers from the user. Be aware that some of these methods above will
force you to create other routines/methods. You are coding dynamic structures - code accordingly.
See page 2 for moro specificity on the methods.
Deliverables:
1) Clearly documented, professionally written source code (in a running form)
2) 3 runs showing all the user menu items. (output) (screenshots are fine)
3) Grading is based on:
a) Meets all requirements (80%)
b) Quality of sourco code (comments, logic, pre/post conditions, etc.) (20%)
For extra credit, you may do the following: (will require research on your own)
[DO NOT DO THIS UNLESS YOUR PROGRAM ALREADY FULLY WORKS]
1) Upgrade the "insert" method to allow for a "variable location insert". (this isn't easy) (5%)
2) Attempt to make 1 or more of the methods recursive. (5%)
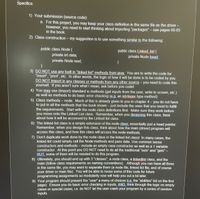
Transcribed Image Text:Specifics:
1) Your submission (source code):
a. For this project, you may keep your class definition in the same file as the driver -
however, you need to start thinking about importing "packages" – see pages 60-65
in the book.
2) Class construction – my suggestion is to use something similar to the following:
public class Node (
public class Linked_ list {
private int data;
private Node head;
private Node next;
3) DO NOT use any built in "linked list" methods from java. You are to write the code for
"insert", "print", etc. In other words, the logic of how it will be done is to be coded by you.
DO NOT import in any classes or methods from any other source - you need to code this
yourself. If you aren't sure what I mean, ask before you codel
4) You may use (import) standard io methods (get inputs from the user, write to screen, etc.)
as well as methods to do basic error checking (e.g, an islnteger type method)
5) Class methods - node. Much of this is already given to you in chapter 4- you do not have
to put in all the methods that the book shows - just include the ones that you need to fulfill
the requirements. Start with the node class definitions first. Make sure they work before
you move onto the Linked List class. Remember, when you designing this class, think
about how it will be accessed by the Linked list class.
6) The linked list class is a simple extension of the node class; essentially just a head pointer.
Remember, when you design this class, think about how the main (driver) program will
access this class, and how this class will access the node methods.
7) Don't duplicate work done by the node class in the linked list classl In many cases, the
linked list could simply call the Node methods and pass data. Use common sense
constructors and methods - include an empty case constructor as well as a 1 variable
constructor. At this point you DO NOT need to do all the traditional "sets" and "gets" -
BUT, some of them will be needed to do this program.
8) Ultimately, you should end up with 3 "classes"; a node class, a linkedlist class, and the
main (follow class requirements on naming conventions). Although you can have all three
in the same file, you may want to separate them (a node file, linked list file, and of course
your driver or main file). You will be able to reuse some of this code for future
programming assignments so modularity now will help you out a lot later.
9) Your program should present the "user" a menu of choices (i.e. the "abilities" list on the first
page). Ensure you do basic error checking in inputs, AND, think through the logic on empty
cases or special cases; ie. do NOT let the user crash your program by a series of random
inputs.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- write a program for a library automation which gets the isbn number, name, author and publication year of the books in the library. the status will be filled by the program as follows: if publication year before 1985 the status is reference else status is available, the information about the books should be stored inside a linked list. the program should have a menu and the user inserts, displays, and deletes the elements from the menu by write a selecting options. the following data structure should be used. struct list char isbn[ 20 ]: char namei 20 ]. char authori 20 ]: int year; char status[200j: struct list "next, jinfo, wedoad n un posn ag pinogs nuu sulnogoj l press 1. to insert a book press 2. to display the book list press 3. to delete a book from listarrow_forwardJava Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Second image is ItemNodearrow_forwardJava language Write a method to insert an array of elements at index in a single linked list and then display this list. The method receives this array by parametersarrow_forward
- In Java please help with the following: Sees whether this list is empty.@return True if the list is empty, or false if not. */ public boolean isEmpty(); } // end ListInterface Hint:Node class definition should look something like: public class Node<T> { T element; Node next; Node prev; public Node(T element, Node next, Node prev) { this.element = element;this.next = next;this.prev = prev; } }arrow_forwardJAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forwardGiven the interface of the Linked-List struct Node{ int data; Node* next = nullptr; }; class LinkedList{ private: Node* head; Node* tail; public: display_at(int pos) const; ... }; Write a definition for a method display_at. The method takes as a parameter integer that indicates the node's position which data you need to display. Example: list: 5 -> 8 -> 3 -> 10 display_at(1); // will display 5 display_at(4); // will display 10 void LinkedList::display_at(int pos) const{ // your code will go here }arrow_forward
- PYTHON LAB: Inserting an integer in descending order (doubly-linked list) Given main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 _____________________________________________________________________________________ Main.py: from IntNode import IntNode from IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py class IntNode: def __init__(self, initial_data, next = None,…arrow_forwardThis is using Data Structures in Javaarrow_forwardYou will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forward
- Having trouble with creating the InsertAtEnd function in the ItemNode.h file below. " // TODO: Define InsertAtEnd() function that inserts a node // to the end of the linked list" Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts ------------------------------------------------------- main.cpp -------------------------------------------------------- #include "ItemNode.h" int main() { ItemNode *headNode; // Create intNode objects ItemNode *currNode; ItemNode *lastNode; string item; int i; int input; // Front of nodes list headNode = new ItemNode(); lastNode = headNode; cin >> input; for (i = 0; i < input; i++) { cin >> item;…arrow_forwardTrue or False For each statement below, indicate whether you think it is True or False. If you like, you can provide a description of your answer for partial credit in case you are incorrect. Use the standard linked list below to answer True/False statements 9-12: 8 7 null 4 10 The “head” pointer of this list is pointing to Node 4 If we called “insert(5)”, the new node’s “next” pointer will point to Node 8 If we called “delete(10)”, Node 7’s “next” pointer will point to Node 8 If we called “search(20)”, the “head” pointer will be at Node 4 after the search function endsarrow_forwardWhich best describes this axiom: aList.getLength ( ) = ( aList.remove ( i ) ) . getLength ( ) + 1 You cannot remove an item from from existing list that is empty Removing an item from an existing list decreases its length by one Removing an item from an existing list increases the list size by one None of thesearrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
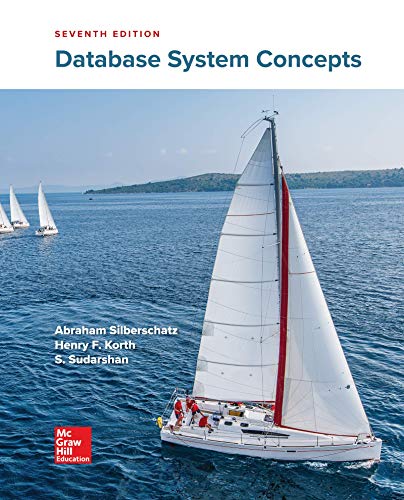
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
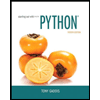
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
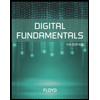
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
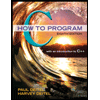
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
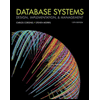
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
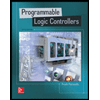
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education