my.tccd.edu Home Page Tarrant County College Upload Assignment: Lab 13 - 2019SU-COSC-1436-11963 My Questions | bartleby Create a new file (in Dev C++) and save it as lab13_XYZ.cpp (replace XYZ with your initials) Write a program that simulates a Magic 8-Ball. Prompt the user for a question, and randomly select a response from a vector. The attached responses.txt file is available if you want to use it, OR you may create your own response file, OR you can add/modify/remove the responses in the attached file. Continue to prompt for questions until the user wants to exit the program (Pick your own exit condition, but the program must loop until the condition is met!) Header comments must be present Prototypes must be present if functions are used Hello and goodbye messages must be shown Responses must be loaded from file (not user input and not hard-coded into program) Use vector(s) for implementation Use comments and good style practices HINT: Read the contents of the response file into an a vector. When the user enters a question, generate a random index and display the response from the vector with that index. Make sure to check for the exit condition Make sure to #include " getRandomLib.h" to help get random indexes (integers). Download the attached header file and place in same location as your program If you use the provided response file, download the attached text file and place in same location as your program. If you create your own response file, make sure to place it in the same location as your program. SAMPLE RUN: Welcome to the Magic 8-Ball program! Ask yes/no questions and the program will give you clarity about the outcome... Enter EXIT to exit the program Please ask your question: Wili I get a good grade in this class? Without a doubt, yes. Please ask your question: Does the professor know what he's talking about? I'll tell you after my nap. Please ask your question: EXIT Exiting program. Goodbye!
- getRandomLib.h:
// This library provides a few helpful functions to return random values
// * getRandomInt() - returns a random integer between min and max values // * getRandomFraction() - returns a random float between 0 and 1
// * getRandomFloat() - returns a random float between min and max values // * getRandomDouble() - returns a random double between min and max values
// * getRandomBool() - returns a random bool value (true or false)
// * getRandomUpper() - returns a random char between 'A' and 'Z', or between min/max values
// * getRandomLower() - returns a random char between 'a' and 'z', or between min/max values
// * getRandomAlpha() - returns a random char between 'A' and 'Z' or 'a' and 'z'
// * getRandomDigit() - returns a random char between '0' and '9', or between min/max values
// * getRandomChar() - returns a random printable char //
// #define GET_RANDOM_LIB_H #include <cstdlib> #include <random> #include <ctime> using namespace std; const int MIN_UPPER = 65; const int MAX_UPPER = 90; const int MIN_LOWER = 97; const int MAX_LOWER = 122; const int MIN_DIGIT = 48; const int MAX_DIGIT = 57; const int MIN_CHAR = 33; const int MAX_CHAR = 126;
//unsigned seed = time(0); int getRandomInt(int min, int max) {
//seed random engine static default_random_engine gen((unsigned int)time(0));
// if max < min, then swap if (min > max) { int temp = min; min = max; max = temp; }
//set up random generator uniform_int_distribution<int> dis(min, max); //range
//need to throw away the first call, as it is always max... static int firstCall = dis(gen); return dis(gen); }
//end getRandomInt() float getRandomFraction() {
//seed random engine static unsigned seed = time(0); return rand()
/ static_cast<float>(RAND_MAX); }
//end getRandomFraction() float getRandomFloat(float min, float max) { if (min > max) { float temp = min; min = max; max = temp; } return (getRandomFraction() * (max - min)) + min; } //end getRandomFloat() double getRandomDouble(double min, double max) { if (min > max) { double temp = min; min = max; max = temp; } return (static_cast<double>(getRandomFraction()) * (max - min)) + min; } //end getRandomDouble() bool getRandomBool() { if (getRandomInt(0,1) == 0) return false; else return true; } //end getRandomBool char getRandomUpper() { return static_cast<char>(getRandomInt(MIN_UPPER, MAX_UPPER)); } //end getRandomUpper() char getRandomUpper(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); } //end getRandomUpper() char getRandomLower() { return static_cast<char>(getRandomInt(MIN_LOWER, MAX_LOWER)); } //end getRandomLower() char getRandomLower(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); } //end getRandomLower() char getRandomAlpha() { if (getRandomBool()) return getRandomUpper(); else return getRandomLower(); } //end getRandomAlpha() char getRandomDigit() { return static_cast<char>(getRandomInt(MIN_DIGIT, MAX_DIGIT)); } //end getRandomDigit() char getRandomDigit(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); } //end getRandomDigit() char getRandomChar() { return static_cast<char>(getRandomInt(MIN_CHAR, MAX_CHAR)); } //end getRandomChar()
//end getRandomFloat() double getRandomDouble(double min, double max) { if (min > max) { double temp = min; min = max; max = temp; } return (static_cast<double>(getRandomFraction()) * (max - min)) + min; }
//end getRandomDouble() bool getRandomBool() { if (getRandomInt(0,1) == 0) return false; else return true; }
//end getRandomBool char getRandomUpper() { return static_cast<char>(getRandomInt(MIN_UPPER, MAX_UPPER)); }
//end getRandomUpper() char getRandomUpper(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); }
//end getRandomUpper() char getRandomLower() { return static_cast<char>(getRandomInt(MIN_LOWER, MAX_LOWER)); }
//end getRandomLower() char getRandomLower(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); }
//end getRandomLower() char getRandomAlpha() { if (getRandomBool()) return getRandomUpper(); else return getRandomLower(); }
//end getRandomAlpha() char getRandomDigit() { return static_cast<char>(getRandomInt(MIN_DIGIT, MAX_DIGIT)); }
//end getRandomDigit() char getRandomDigit(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); }
//end getRandomDigit() char getRandomChar() { return static_cast<char>(getRandomInt(MIN_CHAR, MAX_CHAR)); }
//end getRandomChar()
#endif
- responses.txt
Yes, of course!
Without a doubt, yes.
You can count on it.
For sure! Ask me later.
I'm not sure.
I can't tell you right now.
I'll tell you after my nap.
No way! I don't think so.
Without a doubt, no.
The answer is clearly NO.
Create a new file (in Dev C++) and save it as lab13_XYZ.cpp (replace XYZ with your initials).
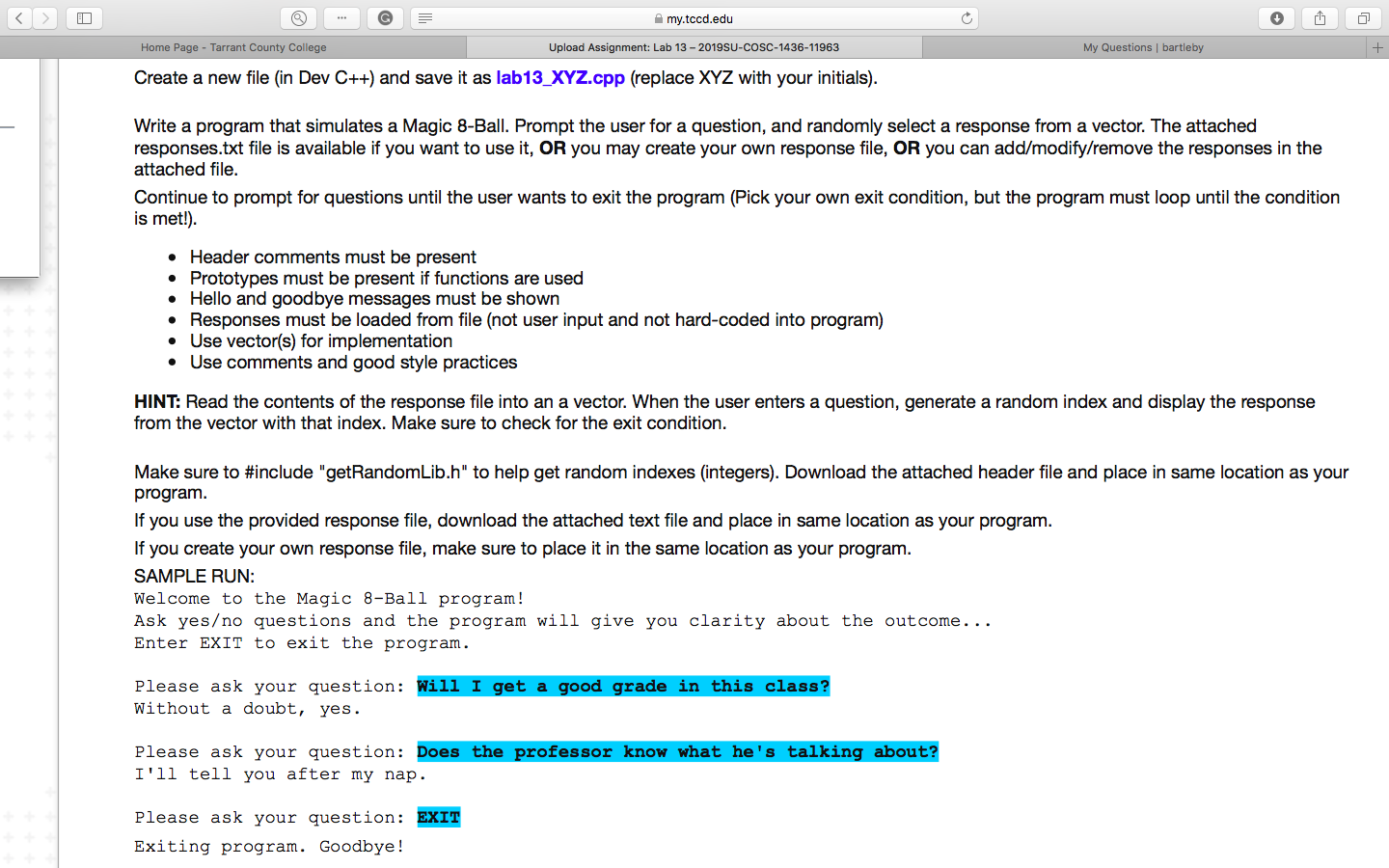

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

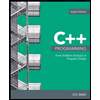
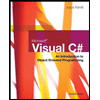
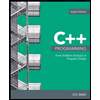
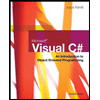