Please modify the following code using getline(cin,strObj) with cout< #include #include #include int main() { string fileOne = "info.txt"; // put the filename up front string fileTwo = "info.bak"; char ch; ifstream inFile; ofstream outfile; try //this block tries to open the input file { // open a basic input stream inFile.open(fileOne.c_str()); if (inFile.fail()) throw fileOne; } // end of outer try block catch (string in) // catch for outer try block { cout << "The input file " << in << " was not successfully opened." << endl << " No backup was made." << endl; exit(1); } try // this block tries to open the output file and { // perform all file processing outfile.open(fileTwo.c_str()); if (outfile.fail())throw fileTwo; while ((ch = inFile.get())!= EOF) outfile.put(ch); inFile.close(); outfile.close(); } catch (string out) // catch for inner try block { cout << "The backup file " << out << " was not successfully opened." << endl; exit(1); } cout << "A successful backup of " << fileOne << " named " << fileTwo << " was successfully made." << endl; return 0; } Chapter 9 501 Exceptions and File Checking thank you
Please modify the following code using getline(cin,strObj) with cout<<strObj<<endl; or getline(infile,strObj) with outfile<<strObj<<endl;
#include <iostream>
#include <fstream>
#include <cstdlib>
#include <string>
int main()
{
string fileOne = "info.txt"; // put the filename up front
string fileTwo = "info.bak";
char ch;
ifstream inFile;
ofstream outfile;
try //this block tries to open the input file
{
// open a basic input stream
inFile.open(fileOne.c_str());
if (inFile.fail()) throw fileOne;
} // end of outer try block
catch (string in) // catch for outer try block
{
cout << "The input file " << in
<< " was not successfully opened." << endl
<< " No backup was made." << endl;
exit(1);
}
try // this block tries to open the output file and
{ // perform all file processing
outfile.open(fileTwo.c_str());
if (outfile.fail())throw fileTwo;
while ((ch = inFile.get())!= EOF)
outfile.put(ch);
inFile.close();
outfile.close();
}
catch (string out) // catch for inner try block
{
cout << "The backup file " << out
<< " was not successfully opened." << endl;
exit(1);
}
cout << "A successful backup of " << fileOne
<< " named " << fileTwo << " was successfully made." << endl;
return 0;
}
Chapter 9 501
Exceptions and File Checking
thank you

Step by step
Solved in 2 steps with 4 images

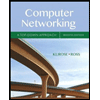
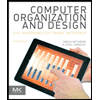
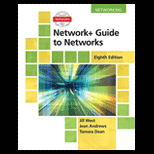
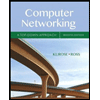
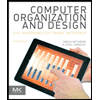
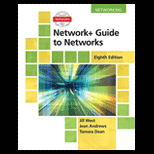
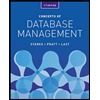
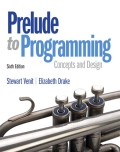
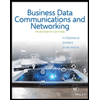