Need help converting this C++ code to java. 1. Bubble Sort #include using namespace std; void swap(int * xp, int * yp) { int temp = * xp; * xp = * yp * yp - temp; } void bubbleSort(int arr[], int n) { int i, j; for (i = 0; i < n - 1; i++) for (j - 0; j < n - i - 1; j++) if (arr[j] > arr[j + 1]) swap( & arr[j], & arr[j + 1]); } void printArray(int arr[], int size) { int i; for (i = 0; i < size; i++) cout << arr[i] << " "; cout << end1; } //Driver ClassLoader int main() { int arr[] = {1, 2, 3, 7, 5, 6, 4}; int n = sizeof(arr)/sizeof(arr[0]); bubbleSort(arr, n); cout <<"Sorted Array: \n"; printArray(arr, n); return 0; } 2. Inserted Sort #include using namespace std; void insertionSort(int arr[], int n) { int i, key, j; { key = arr[i]; j = i-1; while (j >= 0 && arr[j]>key) { arr[j+1]=arr[j]; j=j-1; } arr[j+1]=key; } } void printArray(int arr[], int n) { int i; for (i=0; i
Need help converting this C++ code to java.
1. Bubble Sort
#include <bits/stdc++.h>
using namespace std;
void swap(int * xp, int * yp) {
int temp = * xp;
* xp = * yp *
yp - temp;
}
void bubbleSort(int arr[], int n) {
int i, j;
for (i = 0; i < n - 1; i++)
for (j - 0; j < n - i - 1; j++)
if (arr[j] > arr[j + 1])
swap( & arr[j], & arr[j + 1]);
}
void printArray(int arr[], int size) {
int i;
for (i = 0; i < size; i++)
cout << arr[i] << " ";
cout << end1;
}
//Driver ClassLoader
int main()
{
int arr[] = {1, 2, 3, 7, 5, 6, 4};
int n = sizeof(arr)/sizeof(arr[0]);
bubbleSort(arr, n);
cout <<"Sorted Array: \n";
printArray(arr, n);
return 0;
}
2. Inserted Sort
#include <bits/stdc++.h>
using namespace std;
void insertionSort(int arr[], int n)
{
int i, key, j;
{
key = arr[i];
j = i-1;
while (j >= 0 && arr[j]>key)
{
arr[j+1]=arr[j];
j=j-1;
}
arr[j+1]=key;
}
}
void printArray(int arr[], int n)
{
int i;
for (i=0; i<n; i++)
cout << arr[i] <<" ";
cout << end1;
}
//Driver code//
int main()
{
int arr[] = {7, 2, 4, 3, 1};
int n = sizeof(arr)/sizeof(arr[0]);
insertionSort(arr, n);
printArray(arr, n);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

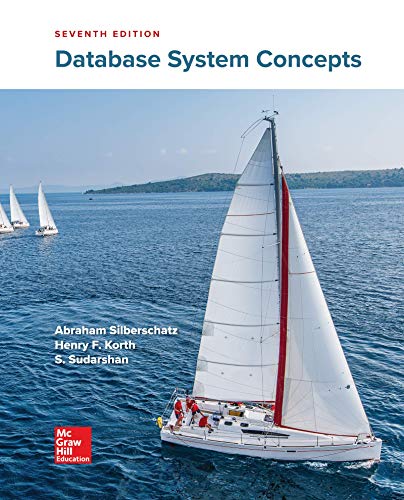
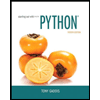
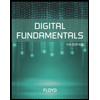
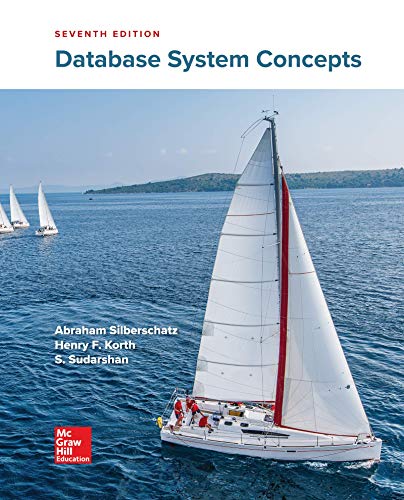
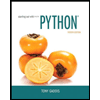
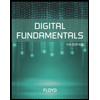
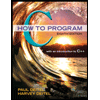
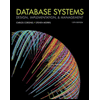
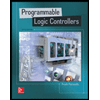