Provided below is the C implementation of the quick sort sorting algorithm. Modify it, so that low, high and middle are pointers to array elements rather than integers. The split function will need to return a pointer, not an integer. #include #define N 10 void quicksort(int a[], int low, int high); int split(int a[], int low, int high); int main(void) { int a[N], i; printf("Enter %d numbers to be sorted: ", N); for (i=0; i= high) return; middle = split(a, low, high); quicksort(a, low, middle - 1); quicksort(a, middle + 1, high); } int split(int a[], int low, int high) { int part_element = a[low]; for (;;) { while (low < high && part_element <= a[high]) high--; if (low >= high) break; a[low++] = a[high]; while (low < high && a[low] <= part_element) low++; if (low >= high) break; a[high--] = a[low]; } a[high] = part_element; return(high); }
Provided below is the C implementation of the quick sort sorting
#include <stdio.h>
#define N 10
void quicksort(int a[], int low, int high);
int split(int a[], int low, int high);
int main(void) {
int a[N], i;
printf("Enter %d numbers to be sorted: ", N);
for (i=0; i<N; i++)
scanf("%d", &a[i]);
quicksort(a, 0, N-1);
printf("In sorted order: ");
for (i=0; i<N; i++)
printf("%d ", a[i]);
printf("\n");
return(0);
}
void quicksort(int a[], int low, int high) {
int middle;
if (low >= high) return;
middle = split(a, low, high);
quicksort(a, low, middle - 1);
quicksort(a, middle + 1, high);
}
int split(int a[], int low, int high) {
int part_element = a[low];
for (;;) {
while (low < high && part_element <= a[high])
high--;
if (low >= high) break;
a[low++] = a[high];
while (low < high && a[low] <= part_element)
low++;
if (low >= high) break;
a[high--] = a[low];
}
a[high] = part_element;
return(high);
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

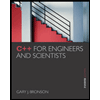
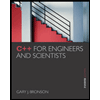