Need help wit
Q: e censor technology? Provide details and examples
A: Reasons for censor technology is an given below :Political concern: one of the most widespread caus...
Q: Incident response guidelines explain the essential components of incident teams and how they are uti...
A: answer is
Q: Examine the advantages and disadvantages of various system models.
A: Introduction:- System modeling is constructing abstract models of a system, each of which gives a di...
Q: You need to show detail work on how you get the answer. Dont use answers from other websites please...
A: Solution: The answer for the above question is given below.
Q: (a) Design a PDA P that recognizes the language L = {x#y : x, y ∈{1}∗∧ |x| > |y|} over the alphabet ...
A: The answer is given below:
Q: Examine the advantages and disadvantages of various system models.
A: Introduction: System modeling is constructing abstract models of a system, each of which gives a dif...
Q: Is it easier for you to identify someone using their fingerprints, speech intonation, face features ...
A: Biometrics validation gadgets depend on actual qualities like a unique mark, facial examples, or iri...
Q: Write a Java program to perform the following task: First define an Employee class which includes em...
A: The answer is given below:-
Q: In the realm of cryptography, what are the three basic operations?
A: Introduction: Only the sender and the intended receiver of a message may see the message's contents ...
Q: Please type your answer not picture Complete the RTN for the MARIE instruction Load X.
A: Here X is stored in MAR Later MBR is stored in accumulator
Q: What are the disadvantages of using the SSTF disc scheduling algorithm?
A: Here in this question we have asked that what are the disadvantage of SSTF disk scheduling algorithm...
Q: A machine cycle is a four-part closed-loop process. Explain why the machine cycle is so important. I...
A: Here we have given answer regarding the importance of machine cycle and feasibility to swap.
Q: On the second access (the access of word 120), in which index will the block containing 120 be place...
A: On the second access, 120 will be placed in 1000 index
Q: If a system's instruction set consists of an 6-bit opcode, what is the maximum number of instruction...
A: 1) An opcode (abbreviated from operation code) is the portion of a machine language instruction that...
Q: A list of five common network topologies should be included. Which one do you think is the most trus...
A: 1) Mesh Topology 2) Star Topology 3) Bus Topology 4) Ring Topology 5) Hybrid Topology
Q: What is the time complexity of the following function- 5T(n/5+50)+n,T(1)=1 (a) θ(50logn) (b) θ(n5...
A: What is the time complexity of the following function- 5T(n/5+50)+n,T(1)=1 (a) θ(50logn) (b) θ(n...
Q: 15. Perform the following binary number Subtraction, using 2's complement : a. 011001, - 101110, b. ...
A: a. 0110012-1011102 Ans:- -0101012 b. 01110102-10011112 Ans:- -00101012
Q: Create Linux User Account: From the command line (terminal )using a Linux Cient. create a user accou...
A: Invoke the useradd command, followed by the user's name, to create a new user account. For example, ...
Q: CODE IN PYTHON In an alternate reality, chemistry Professors Hodge and Sibia have teamed up to de...
A: Given The answer is given below. 1.loop until string length becomes 0 a.create new empty string temp...
Q: What role does the CIA play in information security?
A: Defined the role does the CIA play in information security
Q: In terms of emphasis, what distinguishes the TPC from the SPEC?
A: Let's see the solution in the next steps
Q: Create ERD or EERD of a hospital with these requirements: write DB relational schema draw all foreig...
A: answer is
Q: In C++ Define a function named CoinFlip that returns "Heads" or "Tails" according to a random value...
A: I have provided C++ CODE along with CODE SCREENSHOT and also provided TWO OUTPUT SCREEN...
Q: A. Write an Assembly program to load the register E with the value 12 and the register H with the va...
A: Answer :-
Q: What are the ADO.NET framework's data providers?
A: Introduction: An ADO.NET data provider connects to a data source, such as SQL Server, Oracle, or an ...
Q: Define a function in CoffeeScript that takes a string of lowercase alphabets as a parameter and retu...
A: Define a function in CoffeeScript that takes a string of lowercase alphabets as a parameter and retu...
Q: Exercise 4: Write a program that determines the distance to a lightning strike based between the fla...
A: Answer is given below- Programming in python-
Q: Alice and Bob use the ElGamal scheme with a common prime q = 131 and a primitive root a = 6. Let Bob...
A: The answer is
Q: Use the register and memory values in the tables below for the next questions. Assume a 32-bit Littl...
A:
Q: It's exactly what it sounds like: a jump drive.
A: There are many devices which are used to store information. Jump drive is one of them. It is highly...
Q: Additionally, state briefly what you understand a file extension to be and what its purpose is. Go t...
A: The file extension, also known as a file suffix or filename extension, is the string of characters f...
Q: 9. Find the total amount of tax paid by resident of NJ Hint: The tax cost stored in tax_amount colum...
A: Inner join is used to join two tables. Tax_amount column is given , to find sum value of a column. S...
Q: What is the difference between symmetric and asymmetric key cryptography, and how do they differ?
A: symmetric key cryptography requires only a single key for both encryption and decryption. size of ...
Q: What does the term "reduced" imply in the context of a computer with a restricted instruction set?
A: 1) A Reduced Instruction Set Computer is a type of microprocessor architecture that utilizes a small...
Q: What does the term "abstraction" mean?
A: Introduction: Abstraction, in layman's terms, "shows" just the necessary properties of things while ...
Q: Write a program using JAVA that converts a floating point number to the simple model of the floating...
A: Answer :-
Q: Discuss input data checking, what are the best ways to do it, that is, where should you it? FIT YOUR...
A: Data Validation Or Input data checking is the process which helps in ensuring the accuracy and quali...
Q: When the shell creates a new process to perform a command, how does it determine whether a file is e...
A: The access permissions of a file assist in determining whether or not the file is possible. On the m...
Q: Are there any ideas of how to detect the piano notes played from a recording of piano in python? I a...
A: First we require the list of notes: Twinkle_List = ['c4','c4','g4','g4','a4','a4','g4',\ 'f4','f4...
Q: What is the output of the following segment of code if 4 is input by the us asked to enter a number?...
A: Switch case is branching statement. An expression is passed within switch and corresponding case is ...
Q: Write a java program that creates a 4 x 5 two-dimensional array and fills it with the following numb...
A: Step 1 : Start Step 2 : Declare and Initialize the 4 x 5 Array with the given elements. Step 3 : Usi...
Q: Solve this recurrence equation T(1) = 1 T(n) = T(n/2) + bnlogn
A: Defined the complexity of the given recurrence relation
Q: Problems 1. Modify SiftDown to have the following specification. proc SiftDown (L,U) pre Неap(L+1,U)...
A: Answer: I have given answered in the handwritten format in brief explanation.
Q: Three missionaries and three cannibals are standing at one side of a river and need to be transferre...
A: Draw the state space
Q: Convert the NFA to a DFA a, b а, b 2
A: We know that the power of NFA and DFA is same. So every NFA can be converted to an equivalent DFA. I...
Q: SPIM simulator (QtSpim). Simulation: Write a MIPS program that computes the expression; y = A * B ...
A: Answer :-
Q: What statements about the destructor of an object are accurate? a. The destructor of a class is a m...
A: What statements about the destructor of an object are accurate? a. The destructor of a class is a me...
Q: A list of five common network topologies should be included. Which one do you think is the most trus...
A: Introduction: Many topologies exist, such as the Ring topology. Diagram of a bus Topology based on s...
Q: Four function calculator ( addition, subtraction, multiplication, division) of a single expression i...
A: Lets see the solution in the next steps
Q: In terms of emphasis, what distinguishes the TPC from the SPEC?
A: TPC and SPEC: The Transaction Procession Council (TPC) is a non-profit organisation that creates be...
Need help with this C program please!
For this task, you will complete bit_ops.c by implementing the following three bit manipulation batch functions. You will want to use bitwise operations such as and (&), or (|), xor (^), not (~), left shifts (<<), and right shifts (>>).
#include <stdio.h>
#include <stdlib.h>
// Note, the bits are counted from right to left.
// Return the bit states of x within range of [start, end], in which both are inclusive.
// Assume 0 <= start & end <= 31
unsigned * get_bits(unsigned x,
unsigned start,
unsigned end) {
return NULL;
// YOUR CODE HERE
// Returning NULL is a placeholder
// get_bits dynamically allocates an array a and set a[i] = 1 when (i+start)-th bit
// of x is 1, otherwise siet a[i] = 0;
// At last, get_bits returns the address of the array.
}
// Set the bits of x within range of [start, end], in which both are inclusive
// Assume 0 <= start & end <= 31
void set_bits(unsigned * x,
unsigned start,
unsigned end,
unsigned *v) {
// YOUR CODE HERE
// No return value
// v points to an array of at least (end-start+1) unsigned integers.
// if v[i] == 0, then set (i+start)-th bit of x zero, otherwise, set (i+start)-th bit of x one.
}
// Flip the bits of x within range [start, end], in which both are inclusive.
// Assume 0 <= start & end <= 31
void flip_bits(unsigned * x,
unsigned start,
unsigned end) {
// YOUR CODE HERE
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

- In the following C program, identify what is wrong with code and correct it. This program reads addresses from an input.txt file and sorts the adresses by order of zip codes into an output.txt file. Please also see the following error codes, thank you main.c: #include "contacts.h"#include <stdio.h>#include <stdlib.h> int main(int argc, char **argv) {size_t size;FILE *fp_in, *fp_out;errno_t err; if (argc != 3) {printf("Usage: %s infile outfile", argv[0]);return 0;} err = fopen_s(&fp_in, argv[1], "r");if (err != 0) {perror("Failed opening file for reading");}err = fopen_s(&fp_out, argv[2], "w+");if (err != 0) {perror("Failed opening file for writing");} contact **c = alloc_contacts(MAX_CONTACTS);size = get_contacts(c, fp_in);if (size == 0)perror("get_contacts failed");sort_contacts(c, size);print_contacts(c, size, fp_out);free_alloc(c, size); if (fp_in) {err = fclose(fp_in);if (err != 0) {perror("File could not be closed");}} if (fp_out) {err = fclose(fp_out);if…// Task 2 NEED HELP WITH C programming // For this function, you must build a string that when printed,// will output the entire binary representation of the integer x,// no matter how many bits an integer is. You may NOT use// division (/) or mod (%) anywhere in your code, and should// instead rely on bitwise operations to read the underlying binary// representation of x.stringbuilder get_bin_1(int x) {stringbuilder sb = new_sb();sb_append(sb, '$');return sb;}Write a C program that uses the following: a main() to read two integer values from the user, val1 and val2, and prints the returned value from swap().a swap() that uses call by reference (takes the addresses into pointers) to swap values, and prints their values after the swap "num1 = # and num2 = #". This function returns the largest of the two values. If these are equal, it returns their sum.
- In c++, please. Thank you! Given a main() that reads user IDs (until -1), complete the BubbleSort() functions to sort the IDs in ascending order using the Bubblesort algorithm, and output the sorted IDs one per line. You may assume there will be no more than 100 user IDs. Ex. If the input is: kaylasimms julia myron1994 kaylajones -1 the output is: julia kaylajones kaylasimms myron1994 The following code is given: #include <string>#include <iostream> using namespace std; // TODO: Write the Bubblesort algorithm that sorts the array of string, with k elementsvoid Bubblesort(string userIDs [ ], int k) { } int main() { string userIDList[100]; string userID; cin >> userID; while (userID != "-1") { //put userID in the array cin >> userID; } // Initial call to quicksort Bubblesort(userIDList, /* ?? */ ); //make this output only the userIDs that were entered, not garbage for (int i = 0; i < 100; ++i) { cout <<…This exercise is to write a Decimal to Hex function using BYTE operations. You can use a List, Dictionary or Tuple for your Hex_Map as shown below, but it's not necessary to use one. It is necessary to use Byte operations to convert the decimal to hex. The bitwise operations are also available as __dunders__. Write a function to convert a integer to hexidecimal. Do not use the Python hex function (i.e. hexnumber = hex(number)) to convert the number, instead write your own unique version (i.e. MYHEX: hexnumber = MYHEX(number)). Your function should not use any Python functions like hex, dec, oct, bin or any other Python functions or modules. Functions, like Python ORD, can be used to convert a string to a integer. The input number range is 0 to 1024. def MYHEX(number): hex_map = {10: 'A', 11: 'B', 12: 'C', 13: 'D', 14: 'E', 15: 'F'} result = '' while number: digit = number % 16 if digit > 9: result = hex_map[digit] + result else:…PROGRAM IN PLAIN C PLEASE! Write a void function that will merge the contents of two text files containing chemical elements sorted by atomic number and will produce a sorted file of binary records. The function’s parameters will be three file pointers. Each text file line will contain an integer atomic number followed by the element name, chemical symbol, and atomic weight. Here are two sample lines: 11 Sodium Na 22.99 20 Calcium Ca 40.08 The function can assume that one file does not have two copies of the same element and that the binary output file should have this same property. Hint: When one of the input files is exhausted, do not forget to copy the remaining elements of the other input file to the result file.
- When a variable is stored in memory, it is associated with an address. To obtain the address of avariable, the & operator can be used. For example, &a gets the memory address of variable a.Let's try some examples.Write a C program addressOfScalar.c by inserting the code below in the main function. 1 // intialize a char variable, print its address and the next address2 char charvar = 'a';3 printf("address of charvar = %p\n", (void *)(&charvar));4 printf("address of charvar - 1 = %p\n", (void *)(&charvar - 1));5 printf("address of charvar + 1 = %p\n", (void *)(&charvar + 1));67 // intialize an int variable, print its address and the next address8 double doublevar = 1.0;9 printf("address of doublevar = %p\n", (void *)(&doublevar));10 printf("address of doublevar - 1 = %p\n", (void *)(&doublevar - 1));11 printf("address of doublevar + 1 = %p\n", (void *)(&doublevar + 1)); Questions:1) Run the C program, attach a screenshot of the output in the answer…Computer Science Write a C++ language program that reads the file numbers.txt having 10,000 random integers ranging from 1-1,000,000. It takes an input argument num_jobs with a value between 1 and 8. E.g. if num_jobs is 3, it splits into 3 parallel processes using fork() function and each process then calculates prime numbers from a subset of the input data. Together these 3 jobs find prime numbers from all the 10,000 input integers. The overall parent process then combines the results from its children into a single file prime.txt. NOTE: The key part is to solve it with fork()Computer Science Write a C program that calls malloc() 1001 times allocating blocks of size 4 each. Use type char * to store the pointers returned by malloc(). When done, print the difference in the addresses of the first and last block allocated. This difference is the amount of memory malloc actually used to create 1000 blocks of size 4. It is a lot more than you would expect. Repeat this for other block sizes and make a table of your results. Do you see any pattern? Any guesses why?
- * Assignment: Word operations * * Description: * This assignment asks you to implement common word operations that are * not available in the Scala programming language. The intent is to practice * your skills at working with bits. * */ package wordOps /* * Task 1: Population count (number of 1-bits in a word) * * Complete the following function that takes as parameter * a 32-bit word, w, and returns __the number of 1-bits__ in w. * */ def popCount(w: Int): Int = ??? /* * Task 2: Reverse bit positions * * Complete the following function that takes as parameter * a 16-bit word, w, and returns a 16-bit word, r, such that * for every j=0,1,...,15, * the value of the bit at position j in r is equal to * the value of the bit at position 15-j in w. * */ def reverse(w: Short): Short = ??? /* * Task 3: Left rotation * * Complete the following function that takes two parameters * * 1) a 64-bit word, w, and *…Write a c function that swaps the first and the last elements of chunks in an array. For an array consisting of 9 elements and assuming a chunk of size 3: Array elements: 1 2 3 4 5 6 7 8 9 The output would be, 3 2 1 6 5 4 9 8 7 Inverting here means swapping the last and the first element of a chunk. Note: Size of array should always be multiple of chunk size. User will enter the size of array and chunkI need the code in C programing i am begging you please. There are several test cases. Each test case begins with a line containing a single integer n (1≤n≤1000). Each of the next n lines is either a type-1 command, or an integer 2 followed by an integer x. This means that executing the type-2 command returned the element x. The value of x is always a positive integer not larger than 100. Given a sequence of operations with return values, you’re going to guess the data structure. It is a stack (Last-In, First-Out), a queue (First-In, First-Out), a priority-queue (Always take out larger elements first), not sure(It can be more than one of the three data structures mentioned above) or impossible(It can’t be a stack, a queue or a priority queue). Sample Input Sample output 6 1 1 1 2 1 3 2 1 2 2 2 3 6 1 1 1 2 1 3 2 3 2 2 2 1 2 1 1 2 2 4 1 2 1 1 2 1 2 2 7 1 2 1 5 1 1 1 3 2 5 1 4 2 4 1 2 1 queue not sure impossible stack priority queue impossible
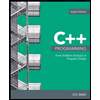
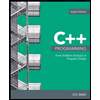