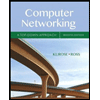
NEED HELP WITH JAVA PROGRAM ERROR
PLEASE HELP THANK YOU
CODE:
class quad{
public static void main(String args[] ){
Quadrilateral quadrilateral = new Quadrilateral( 1.1, 1.2, 6.6, 2.8, 6.2, 9.9, 2.2, 7.4 );
Trapezoid trapezoid = new Trapezoid (0.0, 0.0, 10.0, 0.0, 8.0, 5.0, 3.3, 5.0 );
Parallelogram parallelogram = new Parallelogram (5.0, 5.0, 11.0, 5.0, 12.0, 20.0, 6.0, 20.0 );
Rectagle rectagle = new Rectagle(17.0, 14.0, 30.0, 14.0, 30.0, 28.0, 17.0, 28.0 );
Square square = new Square( 4.0, 0.0, 8.0, 0.0, 8.0, 4.0, 4.0, 4.0 );
System.out.printf("%s %s %s %s %s\n", quadrilateral, trapezoid, parallelogram, rectagle, square );
}
}
class Point{
private double x;
private double y;
public Point(double xCoordinate, double yCoordinate){
x = xCoordinate;
y = yCoordinate;
}
public double getX(){
return x;
}
public double getY(){
return y;
}
public String toString(){
return String.format( "( %.1f, %.1f)", getX(), getY() );
}
}
class Quadrilateral {
private Point point1;
private Point point2;
private Point point3;
private Point point4;
public Quadrilateral( double x1, double y1, double x2, double y2, double x3, double y3, double x4, double y4){
point1 = new Point( x1, y1 );
point2 = new Point( x2, y2 );
point3 = new Point( x3, y3 );
point4 = new Point( x4, y4 );
}
public Point getPoint1(){
return point1;
}
public Point getPoint2(){
return point2;
}
public Point getPoint3(){
return point3;
}
public Point getPoint4(){
return point4;
}
public String toString(){
return String.format( "%s:\n%s","COORDINATES OF QUADRILATERAL ARE: ", getCoordinatesAsString() );
}
public String getCoordinatesAsString(){
return String.format("%s,%s,%s,%s,%s\n", point1, point2, point3,point4 );
}
}
class Trapezoid extends Quadrilateral {
private double height;
public Trapezoid(double x1, double y1, double x2, double y2, double x3, double y3, double x4, double y4){
super(x1, y1, x2, y2, x3, y3, x4, y4);
}
public double getHeight(){
if (getPoint1().getY() == getPoint2().getY() )
return Math.abs( getPoint2().getY() - getPoint3().getY() );
else
return Math.abs( getPoint1().getY() - getPoint2().getY() );
}
public double getArea(){
return getSumOfTwoSides() * getHeight() / 2.0;
}
public double getSumOfTwoSides(){
if (getPoint1().getY() == getPoint2().getY() )
return Math.abs( getPoint1().getX() - getPoint2().getX() ) +
Math.abs( getPoint3().getX() - getPoint4().getX() );
else
return Math.abs( getPoint2().getX() - getPoint3().getX() )+
Math.abs( getPoint4().getX() - getPoint1().getX() );
}
public String toString() {
return String.format("\n%s:\n%s: %s\n%s: %s\n", "COORDINATES OF TRAPEZOID ARE", getCoordinatesAsString(), "Height is:", getHeight(), "Area is:", getArea() );
}
}
class Parallelogram extends Trapezoid{
public Parallelogram( double x1, double y1, double x2, double y2, double x3, double y3, double x4, double y4 ){
super ( x1, y1, x2, y2, x3, y3, x4, y4);
}
public double getWidth(){
if (getPoint1().getY() == getPoint2().getY() )
return Math.abs( getPoint1().getX() - getPoint2().getX() );
else
return Math.abs( getPoint2().getX() - getPoint3().getX() );
}
public String toString(){
return String.format( "\n%s:\n%s%s: %s\n%s: %s\n%s: %s\n","COORDINATES OF PARALELLOGRAM ARE:",getCoordinatesAsString(), "Width is", getWidth(),"Height is", getHeight(),"Area is:", getArea() );
}
}
class Rectagle extends Parallelogram{
public Rectagle( double x1, double y1, double x2, double y2, double x3, double y3, double x4, double y4 ){
super ( x1, y1, x2, y2, x3, y3, x4, y4);
}
public String toString(){
return String.format( "\n%s:\n%s%s: %s\n%s: %s\n%s: %s\n","COORDINATES OF RECTAGLE ARE:",getCoordinatesAsString(), "Width is", getWidth(),"Height is", getHeight(),"Area is:", getArea());
}
}
class Square extends Parallelogram{
public Square( double x1, double y1, double x2, double y2, double x3, double y3, double x4, double y4 ){
super ( x1, y1, x2, y2, x3, y3, x4, y4);
}
public String toString(){
return String.format( "\n%s:\n%s%s: %s\n%s: %s\n%s: %s\n","COORDINATES OF SQUARE ARE:",getCoordinatesAsString(), "Width is", getWidth(),"Height is", getHeight(),"Area is:", getArea() );
}
}
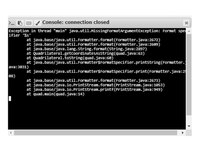

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Javaarrow_forwardC programming #include <stdio.h>int main() {int i, j, n ;printf("height? ") ;scanf("%2d", &n) ;for (i = 1 ; i <= n ; i++) {// printf("%d: ", i) ;for (j = 1 ; j <= i ; j++) {// Pick *one* of the following// printf("%d", j % 10) ;printf("*") ;}printf("\n") ;}return 0 ;} How can I get output like this by editing the given programming -- height? 5************************* and then how can I get the following?- height? 7 1 123 12345 1234567 123456789 12345678901 1234567890123arrow_forwardQ1. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { balance = balance + amount ; } // modified toString() method public String toString() { return "Account: " + actNum + "\tName: " + nameOnAct + "\tBalance: " + amount ; } } Q2. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { // scope of amount starts here balance = balance + amount ; // scope of amount ends here } public void processCheck( int amount ) { // scope of amount starts here int charge; incrementUse(); if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // scope of amount ends here }…arrow_forward
- T/F 1. Java methods can only return primitive typesarrow_forwardDecrease-by-Constant-Factor Fake-Coin puzzle method in Java or C++ to find the fake coin out of n coins. Assume the false coin is lighter. Randomly place the false coin among the n coins. Submit results images and code files.arrow_forwardC++ language pleasearrow_forward
- Charge Account Validation Using Java programming Create a class with a method that accepts a charge account number as its argument. The method should determine whether the number is valid by comparing it to the following list of valid charge account numbers:5658845 4520125 7895122 8777541 8451277 13028508080152 4562555 5552012 5050552 7825877 12502551005231 6545231 3852085 7576651 7881200 4581002These numbers should be stored in an array. Use a sequential search to locate the number passed as an argument. If the number is in the array, the method should return true, indicating the number is valid. If the number is not in the array, the method should return false, indicating the number is invalid.Write a program that tests the class by asking the user to enter a charge account number. The program should display a message indicating whether the number is valid or invalid.arrow_forwardcomplete the missing code. public class Exercise09_04Extra { public static void main(String[] args) { SimpleTime time = new SimpleTime(); time.hour = 2; time.minute = 3; time.second = 4; System.out.println("Hour: " + time.hour + " Minute: " + time.minute + " Second: " + time.second); } } class SimpleTime { // Complete the code to declare the data fields hour, // minute, and second of the int type }arrow_forwardArrays of objects. Java programming: How to create and work with an array of objects in Java I wanted to know that supppose that I have 100 employees who are paid on an hourly basis and I need to keep track of their arrival and departure times, and I have also created the class Clock to implement the time of day in a program. I also know that I will use two arraays; one called arrivalTime and the other array called departure time. Each of these arrays will have 100 elements and each element will be a reference variable of Clock type.. For example Clock[] arrival = new Clock[100]. My qeustion is how do I create the objects arrival and departure. Then how could I use the methods f the class clokc. Thank youarrow_forward
- public class Test { } public static void main(String[] args) { int a = 5; a += 5; } switch(a) { case 5: } System.out.print("5"); break; case 10: System.out.print("10"); System.out.print("0"); default:arrow_forwardFIX THE CODE PROVIDED BELOW Part 2 - Syntax Errors and Troubleshooting The code below has many syntax (and other) errors. Tasks:Compile the code and fix the errors one at a time. // Lab1a.java // This short class has several bugs for practice. // Authors: Carol Zander, Rob Nash, Clark Olson, you public class Lab1a { public static void main(String[] args) { compareNumbers(); calculateDist(); } publicstatic void compareNumbers() { int firstNum = 5; int secondNum; System.out.println( "Sum is: + firstNum + secondNum ); System.out.println( "Difference is: " + (firstNum - secondNum ); System.out.println( "Product is: " + firstNun * secondNum ); } public static void calculateDistance() { int velocity = 10; //miles-per-hour int time = 2, //hoursint distance = velocity * timeSystem.out.println( "Total distance is: " distanace); } } Part 3 - Print Statements and Simple Methods Use "print" and "println" statements, but use method calls to reduce the number of repeated "print"and "println"…arrow_forwardpublic static class Lab2{public static void Main(){int idNum;double payRate, hours, grossPay;string firstName, lastName;// prompt the user to enter employee's first nameConsole.Write("Enter employee's first name => ");firstName = Console.ReadLine();// prompt the user to enter employee's last nameConsole.Write("Enter employee's last name => ");lastName = Console.ReadLine()// prompt the user to enter a six digit employee numberConsole.Write("Enter a six digit employee's ID => ");idNum = Convert.ToInt32(Console.ReadLine());// prompt the user to enter the number of hours employeeworkedConsole.Write("Enter the number of hours employee worked =>");// prompt the user to enter the employee's hourly pay rateconsole.Write("Enter employee's hourly pay rate: ");payRate = Convert.ToDouble(Console.ReadLine());// calculate gross paygrossPay = hours * payRate;// output resultsConsole.WriteLine("Employee {0} {1}, (ID: {2}) earned {3}",firstName, lastName, idNum,…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
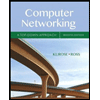
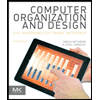
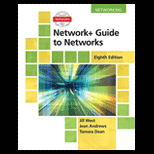
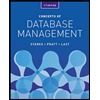
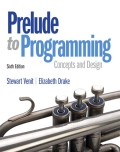
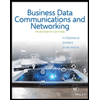