JAVA PROGRAMMING Please Help! Look over my code and explain to me what I'm doing wrong. Thank You! This is my code so far : package initials; import java.util.Random; public class Initials { public static void main(String[] args) { //Create a new array of Strings named strings with the following line of code: //String[] strings = {"Henry Lane Van", "Jakie Marie Johnson", "Oliver Ian Smith", //"Lucas James Whitaker","Billy Bob Washington"}; String[] strings = {"Henry Lane Van", "Jakie Marie Johnson", "Oliver Ian Smith", "Lucas James Whitaker","Billy Bob Washington"}; //Create a for loop to step through the array with the following form "for (int i=0;i
JAVA PROGRAMMING
Please Help! Look over my code and explain to me what I'm doing wrong. Thank You! This is my code so far :
package initials;
import java.util.Random;
public class Initials {
public static void main(String[] args) {
//Create a new array of Strings named strings with the following line of code:
//String[] strings = {"Henry Lane Van", "Jakie Marie Johnson", "Oliver Ian Smith",
//"Lucas James Whitaker","Billy Bob Washington"};
String[] strings = {"Henry Lane Van", "Jakie Marie Johnson", "Oliver Ian Smith", "Lucas James Whitaker","Billy Bob Washington"};
//Create a for loop to step through the array with the following form "for (int i=0;i<strings.length;i++)"
for (int i=0;i<strings.length;i++) {
//The following goes in the loop:
//Set a new String variable named currentName to "[Henry Lane Van]"
String currentName = new String("Henry Lane Van");
//Output "Your first name is [Henry] with a length of [5]"
String firstName = "";
System.out.println("Your first name is "+firstName+" with a length of "+firstName.length());
//Output "Your middle name is [Lane] with a length of [3]"
String middleName = "";
System.out.println("Your middle name is "+middleName+" with a length of "+middleName.length());
//Output "Your last name is [Van] with a length of [5]"
String lastName = "";
System.out.println("Your last name is "+lastName+" with a length of "+lastName.length());
//Output "Your initials are [HLV]. The length of your entire name is [14]"
String initials = ""; initials+=Character.toString(firstName.charAt(0))+Character.toString(middleName.charAt(0))+Character.toString(lastName.charAt(0));
System.out.println("Your initials are "+initials.toUpperCase()+". The length of your entire name is "+currentName.length());
//Output "A random sequence of 5 letters in your name is "[h Lan A]""
//Output two blank lines to separate the name being processed from the next name being processed
System.out.println();
System.out.println();
}
}
}
This is what I'm trying to accomplish:
New project named "Program1Initials" and in a package named "initials". In the sample, items in brackets are just examples for the first iteration of the loop (when the string is Henry Lane Van). These values will change with each iteration of the loop. For more information a sample of output when the program runs is below. Java program that will:
- Create a new array of Strings named strings with the following line of code:
- String[] strings = {"Henry Lane Van", "Jakie Marie Johnson", "Oliver Ian Smith",
"Lucas James Whitaker", "Billy Bob Washington"};
- String[] strings = {"Henry Lane Van", "Jakie Marie Johnson", "Oliver Ian Smith",
- Creates a for loop to step through the array with the following form "for (int i=0;i<strings.length;i++)"
- The following goes in the loop:
- Set a new String variable named currentName to "[Henry Lane Van]"
- Output "Your first name is [Henry] with a length of [5]"
- Output "Your middle name is [Lane] with a length of [4]"
- Output "Your last name is [Van] with a length of [3]"
- Output "Your initials are [HLV]. The length of your entire name is [14]"
- Output "A random sequence of 5 letters in your name is "[L Van]""
- Output two blank lines to separate the name being processed from the next name being processed
Items in brackets will change with each iteration of the loop. See the sample output below.
Values indicated in brackets will change at various times in the program.
Sample correct output:
Your first name is Henry with a length of 5
Your middle name is Lane with a length of 4
Your last name is Van with a length of 3
Your initials are HLV. The length of your entire name is 14
A random sequence of 5 letters in your name is "L Van"
Your first name is Jakie with a length of 5
Your middle name is Marie with a length of 5
Your last name is Johnson with a length of 7
Your initials are JMJ. The length of your entire name is 19
A random sequence of 5 letters in your name is "Jakie"
Your first name is Oliver with a length of 6
Your middle name is Ian with a length of 3
Your last name is Smith with a length of 5
Your initials are OIS. The length of your entire name is 16
A random sequence of 5 letters in your name is "Ian s"
Your first name is Lucas with a length of 5
Your middle name is James with a length of 5
Your last name is Whitaker with a length of 8
Your initials are LJW. The length of your entire name is 20
A random sequence of 5 letters in your name is " Whit"
Your first name is Billy with a length of 5
Your middle name is Bob with a length of 3
Your last name is Washington with a length of 10
Your initials are BBW. The length of your entire name is 20
A random sequence of 5 letters in your name is "ngton"

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

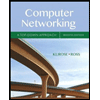
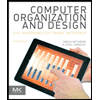
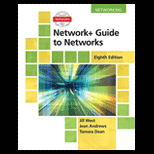
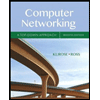
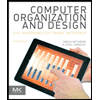
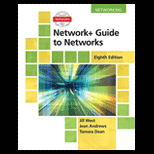
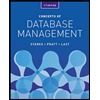
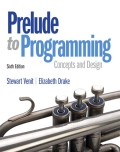
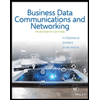