NEED HELP WITH PYTHON CODE AND PLEASE KEEP OUTPUT SAME. Implement the following: 1) Create a class BankAccount with the attribute balance. 2) Create a BankAccount print method that prints the balance. 3) Create a class CheckingAccount which inherits from BankAccount. 4) CheckingAccount has an attribute numChecks. 5) In CheckingAccount override the BankAccount print method to also print numChecks. 6) Instantiate two objects, one of type BankAccount and one of type CheckingAccount. 7) Call the print functions for each object. Example Output Balance: 100000 Balance: 200000 NumChecks: 10
NEED HELP WITH PYTHON CODE AND PLEASE KEEP OUTPUT SAME.
Implement the following:
1) Create a class BankAccount with the attribute balance.
2) Create a BankAccount print method that prints the balance.
3) Create a class CheckingAccount which inherits from BankAccount.
4) CheckingAccount has an attribute numChecks.
5) In CheckingAccount override the BankAccount print method to also print numChecks.
6) Instantiate two objects, one of type BankAccount and one of type CheckingAccount.
7) Call the print functions for each object.
Example Output
Balance: 100000
Balance: 200000 NumChecks: 10

Here we write a python program to perform simple bank operation like withdrawal and deposit of money with using oop concept.
> first of all we define a class BankAccount. Next our code is followed by defining a function using __init__. It is run as soon as an object of a class is instantiated. This __init__method is useful to do any initialization you want to do with object.
# BankAccount class
class Bankaccount:
def __init__(self):
# Function to withdraw the amount
def withdraw(self):
amount = float(input("Enter amount to be withdrawn: "))
if self.balance >= amount:
self.balance -= amount
print("\n You Withdrew:", amount)
else:
print("\n Insufficient balance ")
>Here, we use the display function to show the remaining amount.
# Function to display the amount
def display(self):
print("\n Net Available Balance =", self.balance)
Complete python code:-
# Python program to create Bankaccount class with a deposit() and a withdraw() function
class Bank_Account:
def __init__(self):
self.balance=0
print(" Welcome to the XYZ Bank")
def deposit(self):
amount=float(input("Enter amount to be Deposited: "))
self.balance += amount
print("\n Amount Deposited:",amount)
def withdraw(self):
amount = float(input("Enter amount to be Withdrawn: "))
if self.balance>=amount:
self.balance-=amount
print("\n You Withdrew:", amount)
else:
print("\n Insufficient balance ")
def display(self):
print("\n Net Available Balance=",self.balance)
# Driver code
# creating an object of class
s = Bank_Account()
# Calling functions with that class object
b.deposit()
b.withdraw()
b.display()
Step by step
Solved in 3 steps with 1 images

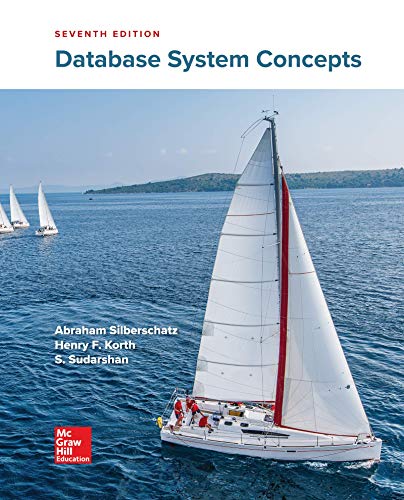
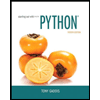
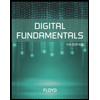
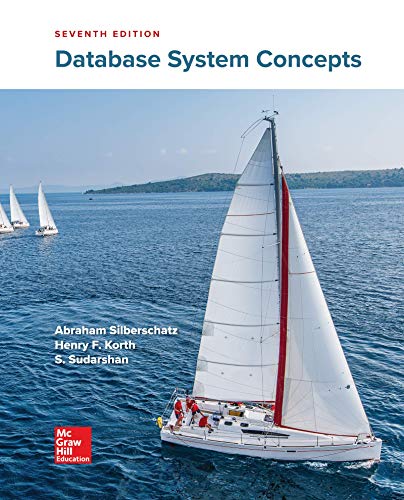
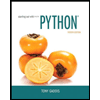
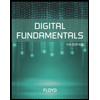
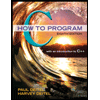
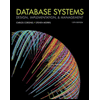
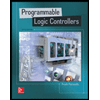