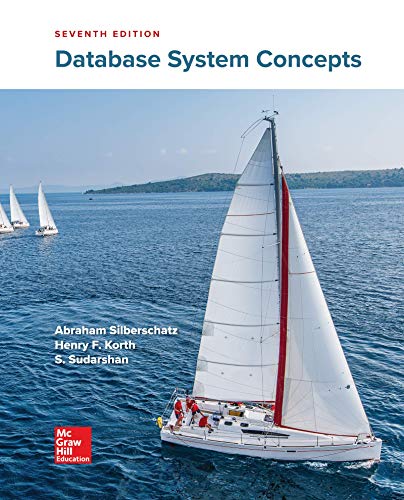
create a class in python with a constructor To create instances of the user defined class and practice applying them. Practice implementing loops.
Part 1 Instructions:
Create a class called BankAccount with the following variables:
balance
name
account_id
Create 1 constructor for the class BankAccount (with or without parameters, your choice, but only 1):
def __init_(self, name, account_id, balance)
def __init_(self)
Create the following methods in the BankAccount class:
def viewAccountInfo() //prints all account information def withdraw(self, amount) //withdraws from the balance def deposit(self, amount) //deposits to the balance
Your task after your class has been created:
- Create two objects, "personal" and "joint_account"
with initial balances of:
personal = $1000
joint_account = $3000
Use the constructor to create the objects. - Create a program that prompts the user which account they would like to access personal or joint
Which account would you like to access?
1. Personal
2. Joint Account
3. Exitonce they select an account, display all account information using the method ViewAccountInfo().
This menu should repeat, the user should be able to select either personal or joint and then be able to select again once they are done with that account. - Next show the following menu:
What would you like to do next:
1. Deposit
2. Withdraw
3. View Balance
4. Update account holder
5. Exit
Do not let them withdraw more than what is in the account, if they attempt, output "Error, Insufficient funds". Once the user has finished depositing or withdrawing to their account, output their old balance, and the new balance.
Part 2 Instructions accessors and mutators
Make all your class variables private.
Do not allow any of the class variables to be modified without a setter (mutator).
For the balance setter, do not allow any values of 0 or lower.
Do not allow account_id to be modified, you can do this by omitting the setter for account_id, or adding some type of error code if they do try.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Summary In this lab, you create a derived class from a base class, and then use the derived class in a Python program. The program should create two Motorcycle objects, and then set the Motorcycle’s speed, accelerate the Motorcycle object, and check its sidecar status. Instructions Open the file named Motorcycle.py. Create the Motorcycle class by deriving it from the Vehicle class. Call the parent class __init()__ method inside the Motorcycle class's __init()__ method. In theMotorcycle class, create an attribute named sidecar. Write a public set method to set the value for sidecar. Write a public get method to retrieve the value of sidecar. Write a public accelerate method. This method overrides the accelerate method inherited from the Vehicle class. Change the message in the accelerate method so the following is displayed when the Motorcycle tries to accelerate beyond its maximum speed: "This motorcycle cannot go that fast". Open the file named MyMotorcycleClassProgram.py. In the…arrow_forwardO Challenge task Write the code of the Constructors and Distructor for the following class 1-Write the code for the empty constructor, that sets X, Y to 0, and prints "Constructor 1 2-Write the code for the parameterized constructor, that takes two integers AB and assigns X A Y=B. and prints the Sentance "Constructor 2 3-Write the code for the Distructor that prints the Sentance "Distruct" followed by the value of X, Y Constraints 5 Input Format the input is two integers E. Output Format as shown in Test case Sample #1 Input 57 Output Constructor 1 Constructor 2 Constructor 1 Destruct 0,0 Destruct 5,7 Destruct 0,0arrow_forwardUsing C++: Write a program that creates a Bus class that includes a bus ID number, number of seats, driver name, and current speed. Create a default constructor without any parameters that initializes the value of ID number, number of seats, and driver name. Create another constructor with three parameters of bus id number, number of seats, and driver name. Car speed should always be initialized as 0. The program will also include the member functions to perform the various operations: modify, set, and display bus information (Bus id, number of seats, driver name, and current speed). For example:- Set the bus id number- Set the number of seats- Set of driver number----------------------------------------------------- Return the bus id number by using get method- Return the number of seats- Return of driver number- Return the current speed-----------------------Member function to display the bus information- busInformation() Also write two member functions, Accelerate() and Brake().…arrow_forward
- Written in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwarda- Write a class definition for Student containing the following instance variables and methods: b- Create an object of this class c- Call the method to calculate and set average_test d- Print average test self. name self. test1 self. test2 self. average test A constructor to accept name, test1 and test2 as parameters and assign them to instant variables. A method to calculate and set averave test (each test worth 50%) A method to get average_testarrow_forwardCSC 1302: PRINCIPLES OF COMPUTER SCIENCE II Lab 6 How to Submit Please submit your answers to the lab instructor once you have completed. Failure to submit will result in a ZERO FOR THIS LAB. NO EXCEPTIONS. The class diagram with four classes Student, Graduate, Undergraduate, and Exchange is given. Write the classes as described in the class diagram. The fields and methods for each class is given below. Student Exchange Graduate Undergraduate Student: Fields: major (String) gpa (double) creditHours (int) Methods: getGpa: returns gpa getYear: returns “freshman”, “sophomore”, “junior” or “senior” as determined by earned credit hours: Freshman: Less than 32 credit hours Sophomore: At least 32 credit hours but less than 64 credit hours Junior: At least credit hours 64 but less than 96 credit hours Senior: At least 96 credit hours Graduate:…arrow_forward
- Write a C++ program that does the following: Create a class called Automobile This class has the following private attributes: model year mileage This class has the following methods: Default constructor Non-default constructor that will initialize all the above attributes Set/Get method for each of the above attributes displayAuto that will print the information for the Automobile all three attributes on the same line with proper spacing Destructor method that will print a message that the object has been deleted Create a class called Inventory This class has the following private attributes: count: This represents how many current automobiles in the inventory maxCount: This represents the maximum number of automobiles that can be stored autoPtr: A pointer to Automobile objects. This class has the following methods: Default constructor (count is 0, maxCount will be 10, autoPtr is NULL) Non-default constructor that will have one integer parameter count will be initialized…arrow_forwardHello can you help me do this Java homework (it's a program)arrow_forwardMake C# (Sharp): console project named MyPlayList. Create an Album class with 4 fields to keep track of title, artist, genre, and copies sold. Each field should have a get/set property. Your Album class should have a constructor to initialize the title (other 3 variables should default to null or 0). In addition, create a public method in the Album class to calculate amount sold for the album (copies sold * cost_of_album). You determine the cost of each album. Also create a method in the Album class to display the information. Print out of information should look something like this: The album Tattoo You by the Rolling Stones, a Rock group, made $25,000,000. In the main method, create 3 instances of the Album class with different albums, use setters to set values of the artist, genre, and copies sold. And then use getters to display information about each on the console.arrow_forward
- 1. Create the main method using pythone to test the classes. #Create the class personTypefrom matplotlib.pyplot import phase_spectrum class personType: #create the class constructor def __init__(self,fName,lName): #Initialize the data members self.fName = fName self.lName = lName #Method to access def getFName(self): return self.fName def getLName(self): return self.lName #Method to manipulate the data members def setFName(self,fName): self.fName = fName def setLName(self,lName): self.lName = lName #Create the class Doctor Type inherit from personTypeclass doctorType(personType): #Create the constructor for the doctorType class def __init__(self, fName, lName,speciality="unknown"): super().__init__(fName, lName) self.speciality = speciality #Methods to access def getSpeciality(self): return self.speciality #Methods to manipulate def setSpeciality(self,spc):…arrow_forwardPythonarrow_forwardC++ Assignment---This is an unfinished code please complete it. Make changes if necessary. Upvote for finished working code. Objectives • To apply knowledge of classes and objects Instructions Implement all of the following, each in a separate Visual Studio project (and/or solution). 1. Define a class called CounterType to implement a simple counter. The class must have a private field (data member) counter of type int. You must also provide methods (member functions), including the following: a. A constructor to set the counter to the value specified by the user b. A constructor to set the counter to 0 c. A method to allow the user to set the counter to a value they specify d. A method to increment the counter by 1 e. A method to decrement the counter by 1 Your code must ensure the value of counter is never negative. If the user calls a method to attempt to make the counter negative, set the counter to 0 and print out an error to the user (e.g., “The counter must not be negative.”) Do…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
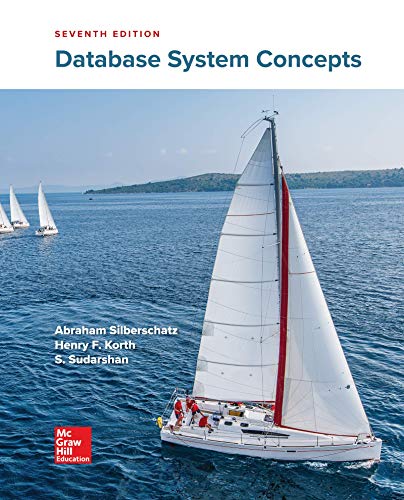
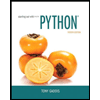
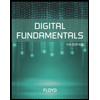
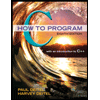
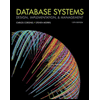
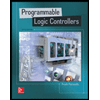