Now, modify it to do the following: Modify the while loop to utilize tolower() or toupper(). Add default values to the to_celsius() and to_fahrenheit() functions.
Now, modify it to do the following: Modify the while loop to utilize tolower() or toupper(). Add default values to the to_celsius() and to_fahrenheit() functions.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section: Chapter Questions
Problem 9PP
Related questions
Question
#include <iostream>
#include <cmath>
using namespace std;
// declare functions
void display_menu();
void convert_temp();
double to_celsius(double fahrenheit);
double to_fahrenheit(double celsius);
int main() {
cout << "Convert Temperatures\n\n";
display_menu();
char again = 'y';
while (again == 'y')
{
convert_temp();
cout << "Convert another temperature? (y/n): ";
cin >> again;
cout << endl;
}
cout << "Bye!\n";
}
// define functions
void display_menu()
{
cout << "MENU\n"
<< "1. Fahrenheit to Celsius\n"
<< "2. Celsius to Fahrenheit\n\n";
}
void convert_temp()
{
int option;
cout << "Enter a menu option: ";
cin >> option;
double f = 0.0;
double c = 0.0;
switch (option)
{
case 1:
cout << "Enter degrees Fahrenheit: ";
cin >> f;
c = to_celsius(f);
c = round(c * 10) / 10; // round to one decimal place
cout << "Degrees Celsius: " << c << endl;
break;
case 2:
cout << "Enter degrees Celsius: ";
cin >> c;
f = to_fahrenheit(c);
f = round(f * 10) / 10; // round to one decimal place
cout << "Degrees Fahrenheit: " << f << endl;
break;
default:
cout << "You must enter a valid menu number.\n";
break;
}
}
double to_celsius(double fahrenheit)
{
double celsius = (fahrenheit - 32.0) * 5.0 / 9.0;
return celsius;
}
double to_fahrenheit(double celsius)
{
double fahrenheit = celsius * 9.0 / 5.0 + 32.0;
return fahrenheit;
}
- Now, modify it to do the following:
- Modify the while loop to utilize tolower() or toupper().
-
- Add default values to the to_celsius() and to_fahrenheit() functions.
- Add default values to the to_celsius() and to_fahrenheit() functions.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
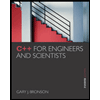
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
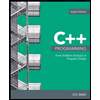
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
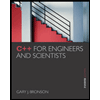
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
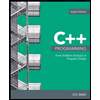
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage