please check my JAVA code. One of my test cases is wrong. The question is as stated in the image My answer: import java.util.*; public class BalanceSymbolChecker { public static void main(String[] args) { Scanner scn=new Scanner(System.in); Stack stack=new Stack(); String str=scn.nextLine(); // take input boolean ans=false; int flag=0; while(str.length()!=0) { char ch=str.charAt(0); str=str.substring(1); if(stack.isEmpty()&&(ch==')'||ch==']'||ch=='}'||ch=='>')) { flag=1; break; } else if(ch=='('||ch=='['||ch=='{'||ch=='<') { stack.push(ch); } else if(ch==')') { char top=(char) stack.peek(); if(top=='(') { stack.pop(); } } else if(ch==']') { char top=(char) stack.peek(); if(top=='[') { stack.pop(); } } else if(ch=='}') { char top=(char) stack.peek(); if(top=='{') { stack.pop(); } } else if(ch=='>') { char top=(char) stack.peek(); if(top=='<') { stack.pop(); } } } if(flag==1) { ans=false; } else { if(!stack.isEmpty()) { ans= false; //unbalanced } else { ans= true; //balanced } } if(ans==true) { System.out.println("Balanced"); } else { System.out.println("Unbalanced"); } } }
please check my JAVA code. One of my test cases is wrong.
The question is as stated in the image
My answer:
import java.util.*;
public class BalanceSymbolChecker {
public static void main(String[] args) {
Scanner scn=new Scanner(System.in);
Stack<Character> stack=new Stack<Character>();
String str=scn.nextLine(); // take input
boolean ans=false;
int flag=0;
while(str.length()!=0) {
char ch=str.charAt(0);
str=str.substring(1);
if(stack.isEmpty()&&(ch==')'||ch==']'||ch=='}'||ch=='>')) {
flag=1;
break;
}
else if(ch=='('||ch=='['||ch=='{'||ch=='<') {
stack.push(ch);
}
else if(ch==')') {
char top=(char) stack.peek();
if(top=='(') {
stack.pop();
}
}
else if(ch==']') {
char top=(char) stack.peek();
if(top=='[') {
stack.pop();
}
}
else if(ch=='}') {
char top=(char) stack.peek();
if(top=='{') {
stack.pop();
}
}
else if(ch=='>') {
char top=(char) stack.peek();
if(top=='<') {
stack.pop();
}
}
}
if(flag==1) {
ans=false;
}
else {
if(!stack.isEmpty()) {
ans= false; //unbalanced
}
else {
ans= true; //balanced
}
}
if(ans==true) {
System.out.println("Balanced");
}
else {
System.out.println("Unbalanced");
}
}
}
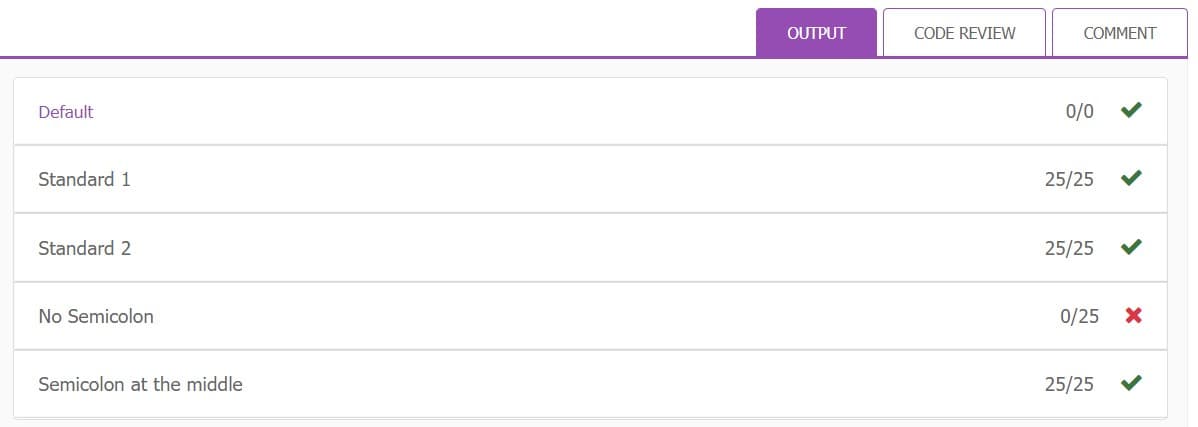
![Problem Description
Compilers check your programs for syntax errors. Frequently, however, a lack of one symbol, such as a missing */ comment-ender, causes the compiler to produce numerous lines of diagnostics without identifying the real error. A useful tool
to help debug compiler error messages is a program that checks whether symbols are balanced.
In other words, every { must correspond to a }, every [ to a ], every (to a) and so on. However, simply counting the numbers of each symbol is insufficient. For example, the sequence [()] is legal, but the sequence [(]) is wrong. A
stack is useful here because we know that when a closing symbol such as ) is seen, it matches the most recently seen unclosed (.
Therefore, by placing an opening symbol on a stack, we can easily determine whether a closing symbol makes sense. The black box shown below represents the Balance Symbol Checker. If we give the input (4+ (2*3), the program will
display the error message. How this is done?
(4+ (23)
Error: Symbol ')' expected.
Challenge yourself to write a program to perform Balance Symbol Checker with implementation Stack.
Input
The input contains any symbols including alphabets or numbers. Each of them is separated into a single space. The input ends with the symbol semicolon. Input example:
(4+ ( 2 * 3 ) ;
(4+ (2* 3 )
Output
The output will be a word either 'Balanced' or 'Unbalanced'. The above input example will give the following output:
Unbalanced
ERROR: no END OF STRING in the expression](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F379f37b2-8125-4ac0-b806-322af81c8467%2Feca909b3-c35d-47d4-8458-c6fb679b583b%2Feut8kcc7_processed.jpeg&w=3840&q=75)

Step by step
Solved in 3 steps with 3 images

Now the last test case is wrong. Can u help me
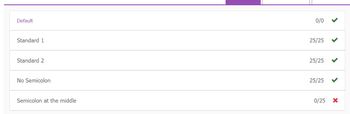
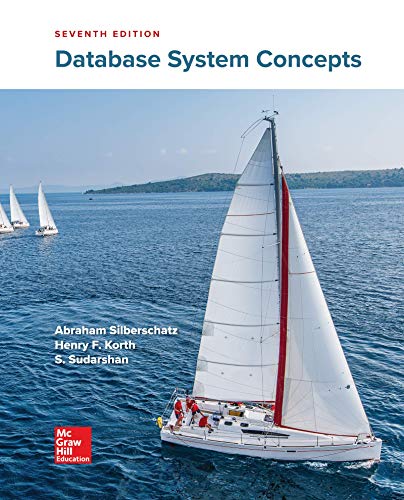
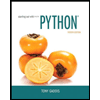
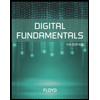
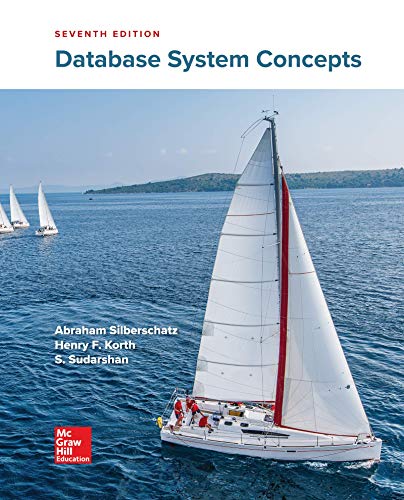
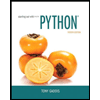
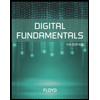
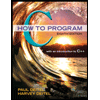
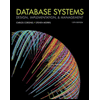
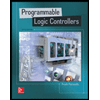