Objectives: At the end of this activity, you should be able to: define classes implement polymorphism and inheritance instantiate an object array apply Exception to all input validations create a Java application for multiple users Procedure: Create a NetBeans project for this activity. The project name should be as follows: o Client (the main class that contains the main method and the implementation of the main menu) o Credit (the class where attributes and methods are defined) The class names to be created are the following: o Client Ex. ClientBlanco o Credit Ex. CreditBlanco All class names must be suffixed with your last name. Note that the object to be instantiated in the main method is an object array. For example: CreditBlanco [ ] cb = new CreditBlanco [100]; Compress the NetBeans project into .rar or .zip format and then upload to the link provided in the LMS. Only NetBeans project compressed in .rar or .zip format will be accepted. Java Credit Main Menu [1] New Credit Account [2] Credit Inquiry [3] Purchases [4] Payment [5] Close Credit Account [7] Exit Program Specifications: 1. New Credit Account Input the name of the client Input the annual income and assign the credit limit as follows: Annual Income Credit Limit 200,000 and above but not greater than 300,000 30,000 300,000 and above but not greater than 500,000 60,000 500,000 and above 100,000 Generate and display a random four-digit credit account number Display also the credit limit. The credit limit is the maximum amount of credit An annual income below 200,000 is not eligible for the credit 2. Credit Inquiry Input the credit account number and validate If the credit account number is valid, display the credit account number, credit account name, credit limit and credit balance. The credit balance is the outstanding balance or the total amount due. 3. Purchases Input the credit account number and validate If the credit account number is valid, input the amount of purchases of not less than Php 1 and not greater than the credit limit and the allowable purchase amount. Compute and update the credit balance and allowable purchase amount as follows: Credit balance+=amount of purchases Interest=3% of credit balance Credit balance+=interest Allowable purchase amount = credit limit – credit balance The allowable purchase amount is the difference between the credit limit and the credit balance. The credit balance should not exceed the credit limit. 4. Payment Input the credit account number and validate If the credit account number is valid, input the amount of payment of not less than Php 1 and not greater than the credit balance Compute and update the credit balance as follows: Credit balance – = amount of payment 5. Close Credit Account Input the credit account number and validate If the credit account number is valid, confirm if the user wants to close the account. If confirmed by the user, he should be required to pay all the credit balance before closing the account. Otherwise, go back to the main menu. If the account has been closed, the credit account should not exist anymore. 6. Exit Terminate the Program 7. All input values must be validated and must be required for re-entry of data when invalid. 8. The use of Exception for all input validations is required. Class Diagrams: Client Credit +main(args:String[]):void -creditAcctNo: long -name: String -creditLimit: double -creditBalance: double +Credit() //setters //getters +inquireCreditBalance() +purchase(double amount, double fixedCreditLimit): double +payCredit(double amount, double fixedCreditLimit) +closeAccount() +validateAcctNo(int acctNo):boolean
Objectives:
At the end of this activity, you should be able to:
- define classes
- implement polymorphism and inheritance
- instantiate an object array
- apply Exception to all input validations
- create a Java application for multiple users
Procedure:
- Create a NetBeans project for this activity. The project name should be as follows:
o Client (the main class that contains the main method and the implementation of the main menu)
o Credit (the class where attributes and methods are defined)
- The class names to be created are the following:
o Client<your_last_name> Ex. ClientBlanco
o Credit<your_last_name> Ex. CreditBlanco
- All class names must be suffixed with your last name.
- Note that the object to be instantiated in the main method is an object array.
For example: CreditBlanco [ ] cb = new CreditBlanco [100]; - Compress the NetBeans project into .rar or .zip format and then upload to the link provided in the LMS.
- Only NetBeans project compressed in .rar or .zip format will be accepted.
Java Credit Main Menu
[1] New Credit Account
[2] Credit Inquiry
[3] Purchases
[4] Payment
[5] Close Credit Account
[7] Exit
Program Specifications:
1. New Credit Account
- Input the name of the client
- Input the annual income and assign the credit limit as follows:
Annual Income | Credit Limit |
200,000 and above but not greater than 300,000 | 30,000 |
300,000 and above but not greater than 500,000 | 60,000 |
500,000 and above | 100,000 |
- Generate and display a random four-digit credit account number
- Display also the credit limit. The credit limit is the maximum amount of credit
- An annual income below 200,000 is not eligible for the credit
2. Credit Inquiry
- Input the credit account number and validate
- If the credit account number is valid, display the credit account number, credit account
name, credit limit and credit balance. - The credit balance is the outstanding balance or the total amount due.
3. Purchases
- Input the credit account number and validate
- If the credit account number is valid, input the amount of purchases of not less than Php 1 and not greater than the credit limit and the allowable purchase amount.
- Compute and update the credit balance and allowable purchase amount as follows:
Credit balance+=amount of purchases
Interest=3% of credit balance
Credit balance+=interest
Allowable purchase amount = credit limit – credit balance
- The allowable purchase amount is the difference between the credit limit and the
credit balance. The credit balance should not exceed the credit limit.
4. Payment
- Input the credit account number and validate
- If the credit account number is valid, input the amount of payment of not less than Php 1 and not greater than the credit balance
- Compute and update the credit balance as follows:
Credit balance – = amount of payment
5. Close Credit Account
- Input the credit account number and validate
- If the credit account number is valid, confirm if the user wants to close the account.
- If confirmed by the user, he should be required to pay all the credit balance before closing the account.
- Otherwise, go back to the main menu.
- If the account has been closed, the credit account should not exist anymore.
6. Exit
- Terminate the Program
7. All input values must be validated and must be required for re-entry of data when invalid.
8. The use of Exception for all input validations is required.
Class Diagrams:
Client | Credit |
+main(args:String[]):void |
-creditAcctNo: long -name: String -creditLimit: double -creditBalance: double |
+Credit() //setters //getters +inquireCreditBalance() +purchase(double amount, double fixedCreditLimit): double +payCredit(double amount, double fixedCreditLimit) +closeAccount() +validateAcctNo(int acctNo):boolean |

Step by step
Solved in 4 steps with 1 images

Hello, I am using this code. However it is showing me an error on my netbeans compiler. Could someone tell what should be done on this errors? Thank you.
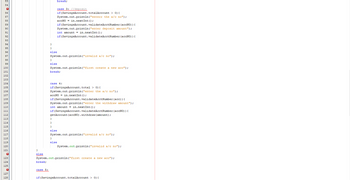
Hello, is it possible if you could explain each
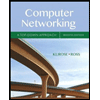
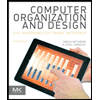
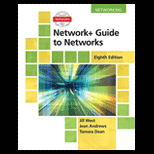
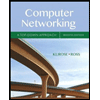
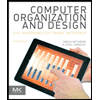
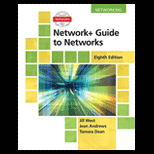
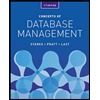
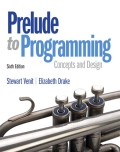
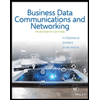