ock-paper-scissors is a game for two players. Each player chooses an action without knowledge of the other’s choice. If the players choose the same action then there is a draw. Otherwise the winner is determined by the following rules: paper beats rock, rock beats scissors, and scissors beats paper. you are going to make a playable Rock-Paper-Scissors game and consider multiplayer tournaments. • Please make it a single Haskell file • Please put comments in your code to show which question you are answering with each piece of code. • You may create auxiliary functions if you like. You may use library functions from Haskell’s standard library. • Please limit your line lengths to 100 characters max. Please use the following two data types which you can copy-and-paste into your code. data Action = Rock | Paper | Scissors deriving (Eq, Show) data Outcome = Player1Win | Player2Win | Draw deriving Show Action represents a player’s chosen action and Outcome represents the outcome of playing a game
Rock-paper-scissors is a game for two players. Each player chooses an action without knowledge of
the other’s choice. If the players choose the same action then there is a draw. Otherwise the winner
is determined by the following rules: paper beats rock, rock beats scissors, and scissors beats paper. you are going to make a playable Rock-Paper-Scissors game and consider multiplayer tournaments.
• Please make it a single Haskell file
• Please put comments in your code to show which question you are answering with each piece of
code.
• You may create auxiliary functions if you like. You may use library functions from Haskell’s
standard library.
• Please limit your line lengths to 100 characters max.
Please use the following two data types which you can copy-and-paste into your code.
data Action = Rock | Paper | Scissors deriving (Eq, Show)
data Outcome = Player1Win | Player2Win | Draw deriving Show
Action represents a player’s chosen action and Outcome represents the outcome of playing a game

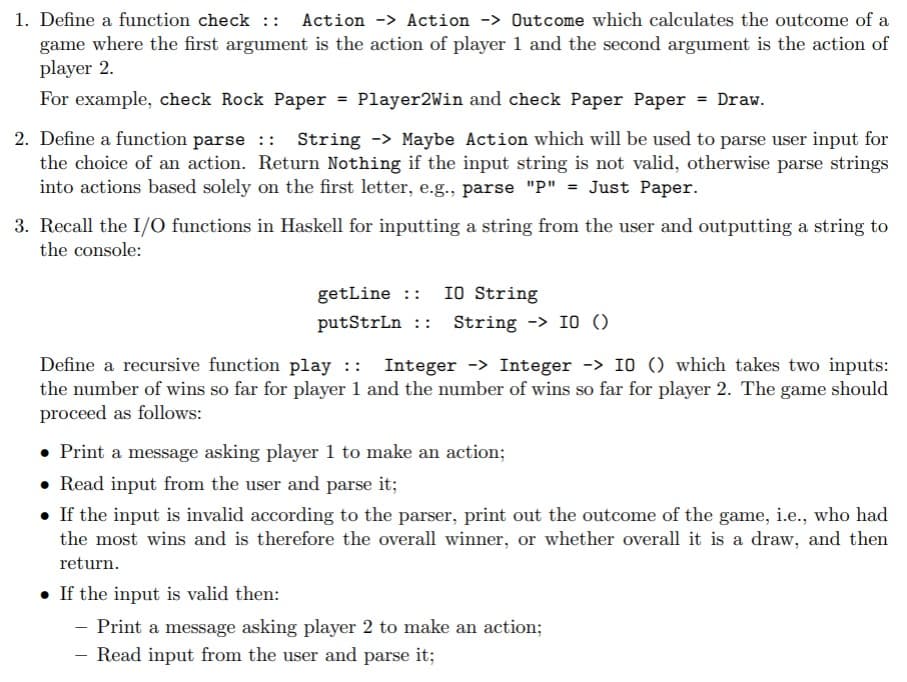

Step by step
Solved in 3 steps with 2 images

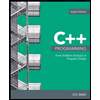
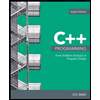