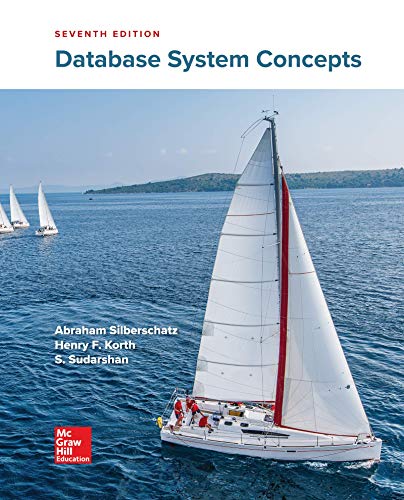
The Binary Search Tree(BST) provided below:
{# Class to represent Tree node
class Node:
# A function to create a new node
def __init__(self, key):
self.data = key
self.left = None
self.right = None
root = Node(4)
root.left = Node(2)
root.right = Node(6)
root.left.left = Node(1)
root.left.right = Node(3)
root.right.left = Node(5)
root.right.right = Node(7)
}
Write a complete Python program to only return the third smallest element in the Binary Search Tree. The value of the third smallest element
is ”3”. You may choose either a recursive or an iterative solution. You
are NOT allowed to actively maintain an extra data-structure for storage
of values of the tree elements to perform a linear scan later to return the
required value.
HINT: A certain BST Traversal is ordered.

Step by stepSolved in 5 steps with 3 images

- class BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forwardJava code that eliminates the binary search's node with the lowest value. returns a reference to its element from a tree. If this tree is empty, it raises an EmptyCollectionException. If the tree is empty, returning a reference to the node with the fewest values produces an EmptyCollectionException.arrow_forwardGiven Class:- import java.util.*; // Iterator, Comparatorpublic class BinarySearchTree<T> implements BSTInterface<T>{protected BSTNode<T> root; // reference to the root of this BSTprotected Comparator<T> comp; // used for all comparisonsprotected boolean found; // used by removepublic BinarySearchTree() // Precondition: T implements Comparable// Creates an empty BST object - uses the natural order of elements.{root = null;comp = new Comparator<T>(){public int compare(T element1, T element2){return ((Comparable)element1).compareTo(element2);}};}public BinarySearchTree(Comparator<T> comp) // Creates an empty BST object - uses Comparator comp for order// of elements.{root = null;this.comp = comp;}public boolean isFull()// Returns false; this link-based BST is never full.{return false;}public boolean isEmpty()// Returns true if this BST is empty; otherwise, returns false.{return (root == null);}public T min()// If this BST is empty, returns null;//…arrow_forward
- How to announce a Linked List Node with three characters in C language, same as the example shown in teaching materials For example is the following figure. EAT O a. struct Node { char *EAT[3]; struct Node next; } Node; O b. struct { Char Three; Link *Next; }; O c. typedef Linked Node { Character EAT; Arrow Link; O d. struct Node { char Three; Arrow Link; }; O e. typedef structure Node { char Name[3]; structure Node *Next; }; O f. structure List Node { Three Character; Link; } O g. typedef struct Node { char name[3]; struct Node *next; } node_C; Oh. typedef struct Node { char EAT; struct *Next; };arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forwardA Dictionary implementation using Binary Search Trees Program requirements and structure You should be able to do the following: Add dictionary entries Search for an entry Print the whole dictionary You will be using the .compareTo method from the String class in order to move through your tree. Recursive method to print the tree in inorder traversal (you need little mods below code) public void printTree(Node root){ if(root != null){ printTree(root.getLeftChild()); System.out.println(root.toSting( )); printTree(root.getRightChild()); } } Instructions: Please use CODE TEMPLATE!arrow_forward
- class BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forwardTree.java: This is a simple binary tree class that should be used to represent the data in your Huffman tree. You only need to implement two of the methods (_printTree and compareTo). Huffman.java: This file contains all of the logic required to implement a Huffman tree. The code in this file relies on the Tree.java file. You must provide the implementation for all of the methods in this class. public class Main { /* * tree.txt should produce this tree: * 16 * / \ * 6 Z * / \ * A D * * A (1), D (5), Z (10) * * Codes: * A: 00 * D: 01 * Z: 1 * * Preorder Traversal: * 16, 6, 1 (A), 5 (D), 10 (Z) */ public static void main(String[] args) throws Exception { Huffman huff = new Huffman(); huff.buildTreeFromFile("tree.txt"); // Print the tree: System.out.println("printTree tests:"); huff.printTree(); // Expected output: [16: , 6: , 1:A, 5:D, 10:Z] // Get some codes:…arrow_forward12:34 A cs61a.org Implement add_d_leaves, a function that takes in a Tree instance t and a number v. We define the depth of a node in t to be the number of edges from the root to that node. The depth of root is therefore 0. For each node in the tree, you should add d leaves to it, where d is the depth of the node. Every added leaf should have a label of v. If the node at this depth has existing branches, you should add these leaves to the end of that list of branches. For example, you should be adding 1 leaf with label v to each node at depth 1, 2 leaves to each node at depth 2, and so on. Here is an example of a tree t (shown on the left) and the result after add_d_leaves is applied with v as 5. 3 2 3 2 4 4 5 5 5 Hint: Use a helper function to keep track of the depth! def add_d_leaves(t, v): """Add d leaves containing v to each ngarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
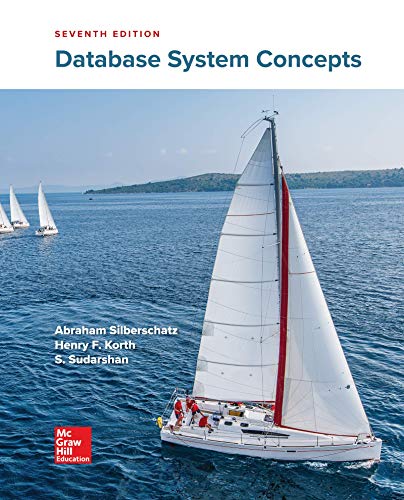
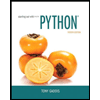
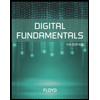
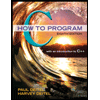
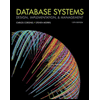
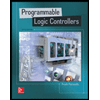