Over the first few Long Weekly Homework assignments for this quarter, we have been building a Sudoku (Links to an external site.) solver program in pieces. This is Part 3 of the Sudoku solv
Overview
Over the first few Long Weekly Homework assignments for this quarter, we have been building a Sudoku (Links to an external site.) solver program in pieces. This is Part 3 of the Sudoku solver series - the last in the series!
In this assignment, we will add a new method to our board class called solve() which will allow us to use recursive backtracking to solve a Sudoku puzzle.
Topics
recursive backtracking
Solving a Sudoku Puzzle
What does it look like to recursively try and solve a Sudoku puzzle? Basically you try to place the values 1-9 in the empty spots of a Sudoku puzzle (move forward with a brute force attempt) until you either reach an invalid state (which causes you to backtrack) or you solve the puzzle or find out it's unsolvable (which ends the recursion).
Instructions
Part 0: Make a copy of your Sudoku #2 Solution
We will be building off your Sudoku #2 solution. I recommend beginning this assignment by making a new folder with a copy of your Sudoku #2 solution just so that you are working in a new space and can easily start over if needed.
- If you did not complete Part 2, you can use this solution to Part 2 of Sudoku Download this solution to Part 2 of Sudokuas starter code for this assignment. Note that I have intentionally provided a solution to Part 2 that is not stylistically beautiful according to my standards; however it does work.
Part 1: solve() method header and initial checks
Add a method to your board class that will allow the puzzle to be solved.
This method is going to take no parameters, as it will operate on the 2D array that is already stored in the Board.
This method will return true or false, depending on whether or not the puzzle can be solved.
- If your board state is invalid - you already have a method that can check this - then you should immediately return false because the board cannot be solved.
- If your board is already solved - you also already have a method that can check this - then you should return true because the board is already solved.
- In all other cases you have an incomplete, but valid, board so you will use recursive backtracking to attempt to solve the puzzle.
- If in your exhaustive searching you find a solution, you'll end up returning true; but
- if you never find a solution, you'll end up returning false.
Part 2: solve() with recursive backtracking
Tackling the recursive backtracking of this solution is not going to require a lot of code. For reference, my solution is around 20 lines of code in total. Yours does not need to be the same length, but I will be surprised if it is much longer. The hard part with recursive backtracking is *thinking* in the appropriate way. I highly recommend reviewing 8 Queens problem from chapter 12 of our text book a the solution is similar to this problem.
The general idea is that you need to try the values 1-9 in each of the empty spaces on your board in an intentional and brute force / exhaustive way. Every time you try a number you check if this creates a valid state on the board and then recurse hoping this leads you to a solution. If it does, you end up returning true up and up out of the recursive calls. However before that is likely to happen, you will hit several dead end solutions where you need to recurse out once and undo whatever change you tried on your board. Again, recursive backtracking is essentially made up of three parts: 1) try something 2) recurse and see if this leads to a solution 3) undo what you tried if the recursive try didn't work out.
Part 3: Test your Sudoku Solver functionality
To test that everything works, run my SudokuSolverEngine.java Download SudokuSolverEngine.javausing these provided board states: very-fast-solve.sdk Download very-fast-solve.sdkand fast-solve.sdk Download fast-solve.sdkI am giving you these test boards because it turns out that recursive backtracking is both memory in-efficient and slow. This means that if you try to solve() some of the boards from Sudoku #2, the solve() method might run for a very long time.
You need to add two things to the SolverEngine.
- Before trying to solve the board, check if the board is in an invalid state. If it is, print a message to the screen that says that the board cannot be solved.
- You can test with the any of the rules violating boards from Sudoku #2
- Before trying to solve the board, check if the board is already solved. If it is, print a message to the screen that says that the board is already solved.
- You can test with the valid-complete.sdk board from Sudoku #2
What to Submit
- Your modified board class
- The SudokuSolverEngine with your modifications
Submission Requirements
You should do the following for _all_ assignments submitted for this course.


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

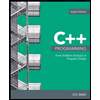
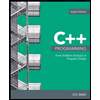