// The language is java Part I: Use of recursive method (4 points for students who need recursion/extracredit) Description: Code a class called RecursiveMultiply in which it will accept two integer arguments entered from user; pass these integers to a recursive method that returns the multiplication result of these two integers. The multiplication can be performed as repeated addition, for example, if two integers are 7 and 4: 8 * 4 = 4 + 4 + 4 + 4 + 4 + 4 + 4 + 4 You will write the application with GUI and recursion to display the result in a noneditable text field, based on the user’s entry from two text fields, while an OK button is pressed. You will utilize the exception handling code as you did in your Lab 4 and Lab 5 to verify if data entered in the text fields are valid (numerical and positive data only). You will make your own decision if there is any explanation that is not described in this specification. Finally, code a driver program that will test your class RecursiveMultiply. Part II: GUI and animation
// The language is java
Part I: Use of recursive method (4 points for students who need recursion/extracredit) Description: Code a class called RecursiveMultiply in which it will accept two integer arguments entered from user; pass these integers to a recursive method that returns the multiplication result of these two integers. The multiplication can be performed as repeated addition, for example, if two integers are 7 and 4: 8 * 4 = 4 + 4 + 4 + 4 + 4 + 4 + 4 + 4 You will write the application with GUI and recursion to display the result in a noneditable text field, based on the user’s entry from two text fields, while an OK button is pressed. You will utilize the exception handling code as you did in your Lab 4 and Lab 5 to verify if data entered in the text fields are valid (numerical and positive data only). You will make your own decision if there is any explanation that is not described in this specification. Finally, code a driver program that will test your class RecursiveMultiply. Part II: GUI and animation

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

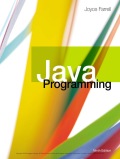
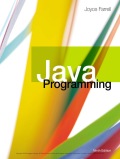