package DataStructures; import Exceptions.ElementNotFoundException; import Exceptions.EmptyCollectionException; import Exceptions.InvalidArgumentException; import org.junit.Test; import static org.junit.Assert.*; /** * * @author ITSC 2214 Q * @version 1.0 */ public
package DataStructures;
import Exceptions.ElementNotFoundException;
import Exceptions.EmptyCollectionException;
import Exceptions.InvalidArgumentException;
import org.junit.Test;
import static org.junit.Assert.*;
/**
*
* @author ITSC 2214 Q
* @version 1.0
*/
public class CircularDoublyLinkedListTest {
public CircularDoublyLinkedListTest() {
}
/**
* Test of first method, of class CircularDoublyLinkedList.
*/
@Test(expected=EmptyCollectionException.class)
public void testFirst1() throws Exception {
CircularDoublyLinkedList<Integer> circle = new CircularDoublyLinkedList<Integer>();
circle.first();
}
/**
* Test of first method, of class CircularDoublyLinkedList.
*/
@Test
public void testFirst2() throws Exception {
CircularDoublyLinkedList<Integer> circle = new CircularDoublyLinkedList<Integer>();
circle.addFirst(10);
assertEquals(circle.first().intValue(), 10);
}
/**
* Test of current method, of class CircularDoublyLinkedList.
*/
@Test
public void testCurrent() {
CircularDoublyLinkedList<Integer> circle = new CircularDoublyLinkedList<Integer>();
assertNull(circle.current());
circle.addFirst(10);
assertEquals(circle.current().intValue(), 10);
}
/**
* Test of last method, of class CircularDoublyLinkedList.
*/
@Test(expected=EmptyCollectionException.class)
public void testLast() throws Exception {
CircularDoublyLinkedList<Integer> circle = new CircularDoublyLinkedList<Integer>();
circle.addLast(10);
assertEquals(circle.last().intValue(), 10);
circle.removeLast();
circle.last();
}
/**
* Test of next method, of class CircularDoublyLinkedList.
*/
@Test
public void testNext() {
CircularDoublyLinkedList<Integer> circle = new CircularDoublyLinkedList<Integer>();
circle.next();
circle.addFirst(10);
circle.addLast(17);
circle.next();
assertEquals(circle.current().intValue(), 17);
assertEquals(circle.size(), 2);
try{
assertEquals(circle.getNode(1).getElement().intValue(), 10);
}
catch (Exception ex){
fail("testNext");
}
}
/**
* Test of isEmpty method, of class CircularDoublyLinkedList.
*/
@Test
public void testIsEmpty() {
CircularDoublyLinkedList<Integer> circle = new CircularDoublyLinkedList<Integer>();
assertTrue(circle.isEmpty());
circle.addFirst(10);
assertFalse(circle.isEmpty());
}
/**
* Test of printList method, of class CircularDoublyLinkedList.
*/
@Test
public void testPrintList() {
CircularDoublyLinkedList<Integer> circle = new CircularDoublyLinkedList<Integer>();
circle.printList();
circle.addFirst(10);
circle.printList();
}
/**
* TODO Test of addLast method, of class CircularDoublyLinkedList.
*/
@Test
public void testAddLast() {
}
/**
* Test of addFirst method, of class CircularDoublyLinkedList.
*/
@Test
public void testAddFirst() {
CircularDoublyLinkedList<Integer> circle = new CircularDoublyLinkedList<Integer>();
circle.addFirst(10);
assertEquals(circle.size(), 1);
assertEquals(circle.current().intValue(), 10);
circle.addFirst(17);
assertEquals(circle.size(), 2);
try {
assertEquals(circle.getNode(0).getElement().intValue(), 17);
assertEquals(circle.getNode(1).getElement().intValue(), 10);
}
catch (Exception ex){
ex.printStackTrace();
}
}
/**
* Test of addAfter method, of class CircularDoublyLinkedList.
*/
@Test
public void testAddAfter() throws Exception {
CircularDoublyLinkedList<Integer> circle = new CircularDoublyLinkedList<Integer>();
circle.addFirst(10);
circle.addAfter(10, 17);
assertEquals(circle.size(), 2);
try {
assertEquals(circle.getNode(0).getElement().intValue(), 10);
assertEquals(circle.getNode(1).getElement().intValue(), 17);
}
catch (Exception ex){
ex.printStackTrace();
}
}
/**
* Test of remove method, of class CircularDoublyLinkedList.
*/
@Test(expected=EmptyCollectionException.class)
public void testRemove1() throws Exception {
//TODO complete the Unit testing
//of the EmptyCollectionException in Remove() method
}
/**
* Test of remove method, of class CircularDoublyLinkedList.
*/
@Test(expected=ElementNotFoundException.class)
public void testRemove2() throws Exception {
//TODO complete the Unit testing
//of the ElementNotFoundException in Remove() method
}
/**
* Test of remove method, of class CircularDoublyLinkedList.
*/
@Test
public void testRemove3() throws Exception {
//TODO complete the Unit testing of
//logic correctness of the remove() method
}
/**
* Test of removeFirst method, of class CircularDoublyLinkedList.
*/
@Test(expected=EmptyCollectionException.class)
public void testRemoveFirst1() throws Exception {
CircularDoublyLinkedList<Integer> circle = new CircularDoublyLinkedList<Integer>();
circle.removeFirst();
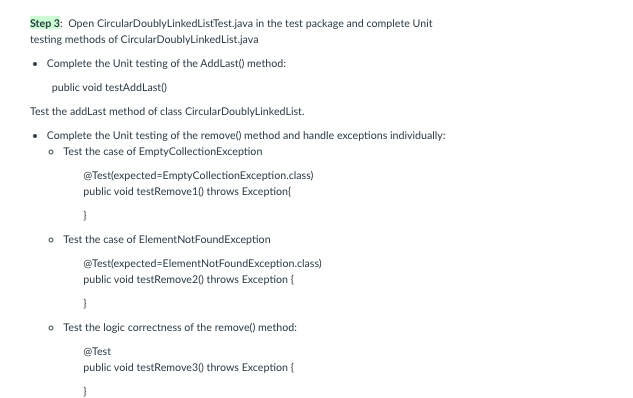

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

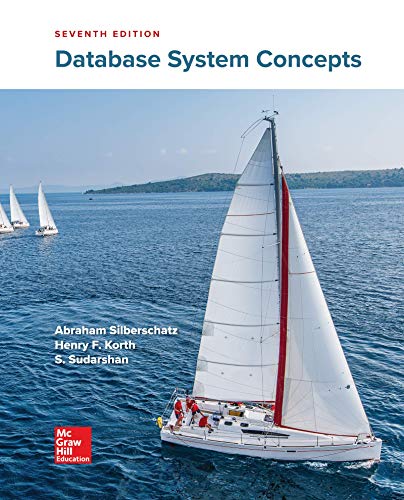
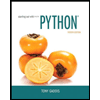
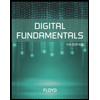
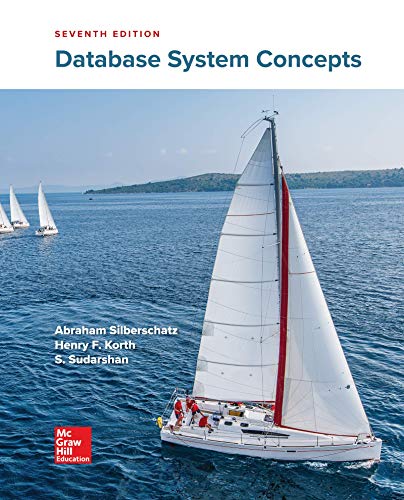
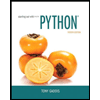
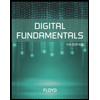
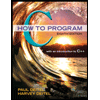
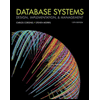
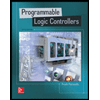