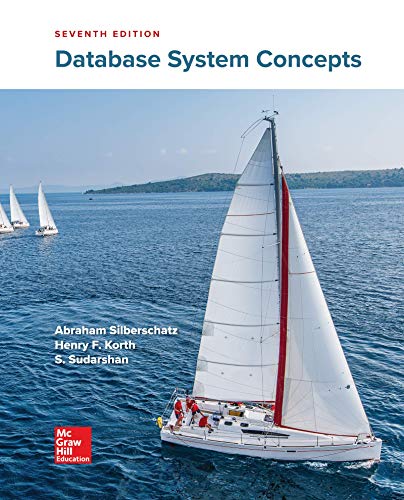
Concept explainers
Write a method that removes the value of a node with index i from a singly-linked list. Also, you must raise exceptions when necessary.
You must NOT use any other methods that **you implemented** in the assignment (everything must be done inline). (In python
Consider the following class definition:
class SLLException(Exception):
"""
Custom exception class to be used by Singly Linked List
DO NOT CHANGE THIS CLASS IN ANY WAY
"""
pass
class SLNode:
"""
Singly Linked List Node class
DO NOT CHANGE THIS CLASS IN ANY WAY
"""
def __init__(self, value: object) -> None:
self._next = None
self._value = value
class LinkedList:
def __init__(self, start_list=None):
"""
Initializes a new linked list with front and back sentinels
DO NOT CHANGE THIS METHOD IN ANY WAY
"""
self._head = SLNode(None)
self._tail = SLNode(None)
self._head._next = self._tail
# populate SLL with initial values (if provided)
# before using this feature, implement add_back() method
if start_list is not None:
for value in start_list:
self.add_back(value)
def __str__(self) -> str:
"""
Return content of singly linked list in human-readable form
DO NOT CHANGE THIS METHOD IN ANY WAY
"""
out = 'SLL ['
if self._head._next != self._tail:
cur = self._head._next._next
out = out + str(self._head._next._value)
while cur != self._tail:
out = out + ' -> ' + str(cur._value)
cur = cur._next
out = out + ']'
return out
def length(self) -> int:
"""
Return the length of the linked list
DO NOT CHANGE THIS METHOD IN ANY WAY
"""
length = 0
cur = self._head
while cur._next != self._tail:
cur = cur._next
length += 1
return length
def is_empty(self) -> bool:
"""
Return True is list is empty, False otherwise
DO NOT CHANGE THIS METHOD IN ANY WAY
"""
return self._head._next == self._tail
# ------------------------------------------------------------------ #
def remove_at_index(self, index: int) -> None :
"""
TODO: Write this implementation
"""
return

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Java: Code a generic class called Location that describes a point in the xy plane. The Location class requires instances variables to represent the x and y coordinates, a parameterized constructor, one method to change both coordinates of the point (both x and y), getX and getY methods and a toString. These are the only methods in this class. The class uses a generic type so that any numeric value can be used for the coordinates (2 integers, 2 floats, 2 doubles, etc) or 2 Strings (for example three and ten). Write a code segment in main( ) to thoroughly test the class Location. Your code segment should create two locations, one represented by a pair of real numbers and one represented by a pair of Strings.arrow_forwardWrite a C#program that uses a class called ClassRegistration as outlined below: The ClassRegistration class is responsible for keeping track of the student id numbers for students that register for a particular class. Each class has a maximum number of students that it can accommodate. Responsibilities of the ClassRegistration class: It is responsible for storing the student id numbers for a particular class (in an array of integers) It is responsible for adding new student id numbers to this list (returns boolean) It is responsible for checking if a student id is in the list (returns a boolean) It is responsible for getting a list of all students in the class (returns a string). It is responsible for returning the number of students registered for the class (returns an integer) It is responsible for returning the maximum number of students the class is allowed (returns an integer) It is responsible for returning the name of the class. (returns a string) ClassRegistration -…arrow_forwardPlease Solve in Java, Thank you!arrow_forward
- In Java please help with the following: Define a class Registration to implement course registration/withdrawal of students. The program should place the code into a try-catch block with multiple catches to check for the validity of various attributes based on the following criteria. a. Student ID must start with a letter and should be followed by four digits. b. The default number of courses must be at least 3. -Print suitable error matches within the catch block. If any of the criteria mentioned above is not fulfilled, the program should loop back and let the user enter new data. -Add methods for the number of courses registered and withdrawn. Create your own exception class which will check inside the method for the courses registered so that after the registration, the maximum number of registered courses should not be more than 6. Also, check inside the method for course withdrawal so that the number of courses after the withdrawal does not go less than 2. -Invoke the…arrow_forwardThis is a debugging question - The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. Code I fixed but seem to be missing a check, I will add a screenshot - // Catch exceptions for array index out of bounds // or dividing by 0 import java.util.*; public class DebugTwelve3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String inStr; int num, result; int[] array = {12, 4, 6, 8}; System.out.println("Enter a number "); inStr = input.nextLine(); try { num = Integer.parseInt(inStr); for (int x = 0; x < array.length; ++x) { result = array[x] / num; System.out.println("Result of division is " + result); } result = array[num];…arrow_forwardPlease explainarrow_forward
- Javaarrow_forwardI am writing a method to remove the first element in a doubly linked list. However I am supposed to throw an exception when the list is empty. When I test my class I keep getting "testRemoveFirst caused an ERROR: Uncompilable source code - exception Exceptions.EmptyCollectionException is never thrown in body of corresponding try statement" What does that mean and why is it not able to run?(I'm coding in Java)arrow_forwardA) Consider the generic print() method, write a method that takes an array of E, where E must be Comparable or extend a type that is Comparable, and returns a reference to the greatest object in the array. In this case, the greatest value is one for which compareTo() would return 0 or more for any other object in the array. If there is more than one possible largest value (these would have to be equal to each other but greater than any other objects in the array), it does not matter which one you choose. B) Write a generic Java queue class (a plain queue, not a priority queue). Call it GenericQueue, because the JDK already has an interface called Queue. The class must be able to create a queue of objects of any reference type. Consider the GenericStack class shown in the lecture notes for some hints. Like the Stack, the GenericQueue should use an underlying ArrayList<E>. Write these methods and any others you find useful: enqueue() adds an E to the queue peek() returns a…arrow_forward
- We may alter the throws clause by overriding a superclass function. Is such a thing even possible?arrow_forwardWrite a Program to define a Mobile class, with member variables String brand; double price String OS (make it Final). Float memory Make a default constructor, Make a parameterized constructor. Overload the above Constructor. Provide getters and setters for data members. Inside main, create an array of mobiles. Take data from user and set the values for the mobiles in array (check for array out of bound exception). At the end Print data of array using Loop.arrow_forwardJAVA Program(*MUST WORK IN HYPERGRADE*) Homework #1. Chapter 7. PC# 2. Payroll Class (page 488-489) Write a Payroll class that uses the following arrays as fields: * employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers: 5658845 4520125 7895122 8777541 8451277 1302850 7580489 * hours. An array of seven integers to hold the number of hours worked by each employee * payRate. An array of seven doubles to hold each employee’s hourly pay rate * wages. An array of seven doubles to hold each employee’s gross wages The class should relate the data in each array through the subscripts. For example, the number in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array. The class should have a method that accepts an…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
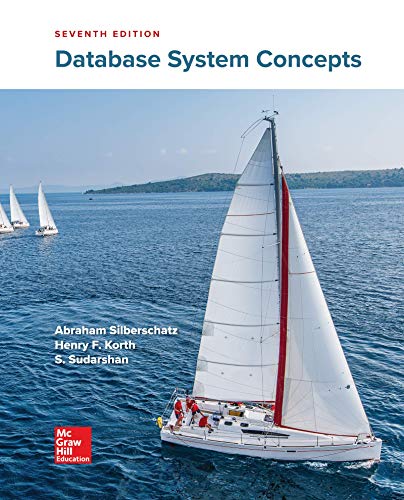
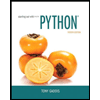
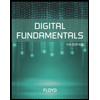
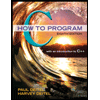
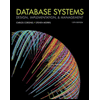
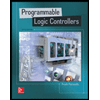