package psa.naloga1; public class NodeBinarno { private static int counter; private int key; public int compare(NodeBinarno node) { counter++; return node.key - this.key; } public int getCounter() { return counter; } public void resetCounter() { counter=0; } }//don't rewrite this package psa.naloga1; public class Binarno { private NodeBinarno root; public boolean insert(int element) { if (root == null) { root = new NodeBinarno(element); return true; } else { return root.insert(element); } } public boolean delete(int element) { if (root == null) { return false; } if (root.getKey() == element) { NodeBinarno temporary = new NodeBinarno(0); temporary.left = root; boolean close = root.delete(temporary, element); root = temporary.left; return close; } else { return root.delete(null, element); } } public boolean search(int element) { if (root == null) { return false; } return root.search(root, element); } public int getCounter() { return root != null?root.getCounter():null; } public void resetCounter() { if(root!= null) root.resetCounter(); } } rewrite this code in another way
package psa.naloga1;
public class NodeBinarno {
private static int counter;
private int key;
public int compare(NodeBinarno node) {
counter++;
return node.key - this.key;
}
public int getCounter() {
return counter;
}
public void resetCounter() {
counter=0;
}
}//don't rewrite this
package psa.naloga1;
public class Binarno {
private NodeBinarno root;
public boolean insert(int element) {
if (root == null) {
root = new NodeBinarno(element);
return true;
} else {
return root.insert(element);
}
}
public boolean delete(int element) {
if (root == null) {
return false;
}
if (root.getKey() == element) {
NodeBinarno temporary = new NodeBinarno(0);
temporary.left = root;
boolean close = root.delete(temporary, element);
root = temporary.left;
return close;
}
else {
return root.delete(null, element);
}
}
public boolean search(int element) {
if (root == null) {
return false;
}
return root.search(root, element);
}
public int getCounter() {
return root != null?root.getCounter():null;
}
public void resetCounter() {
if(root!= null)
root.resetCounter();
}
}
rewrite this code in another way

Step by step
Solved in 6 steps with 4 images

package psa.naloga1;
public class NodeBinarno {
private static int counter;
private int key;
NodeBinarno left;
NodeBinarno right;
public NodeBinarno(int key) {
this.key = key;
this.left = null;
this.right = null;
}
public NodeBinarno() {
this.left = null;
this.right = null;
}
public NodeBinarno getLeft() {
return this.left;
}
public NodeBinarno setLeft(NodeBinarno left) {
return this.left = left;
}
public NodeBinarno getRight() {
return this.right;
}
public NodeBinarno setRight(NodeBinarno right) {
return this.right = right;
}
public int getKey() {
return this.key;
}
public boolean search(NodeBinarno root, int el) {
NodeBinarno c1 = new NodeBinarno(el);
if (root != null) {
int comp = root.compare(c1);
if (comp == 0) {
return true;
}
else if (comp < 0) {
return search(root.left, el);
}
else if (comp > 0) {
return search(root.right, el);
}
} return false;
}
public boolean insert(int el) {
NodeBinarno current = new NodeBinarno(el);
if ((compare(current)) == 0) {
return false;
}
else if ((compare(current)) > 0){
if (right == null) {
right = current;
return true;
}
else {
return right.insert(el);
}
}
else if ((compare(current)) < 0) {
if (left == null) {
left = current;
return true;
}
else {
return left.insert(el);
}
}
return false;
}
public boolean delete(NodeBinarno root, int element) {
NodeBinarno current = new NodeBinarno(element);
int cmp = compare(current);
if (cmp == 0) {
if (left != null && right != null) { // 2 children
int min = right.findMin();
this.key = min;
right.delete(this, this.key);
}
else if (root.right == this) {
root.right = (left != null) ? left : right;
}
else if (root.left == this) { // 1 child
root.left = (left != null) ? left : right;
}
return true;
}
if (cmp < 0) {
return (left != null) ? left.delete(this, element) : false;
}
return (right != null) ? right.delete(this, element) : false;
}
public int findMin() {
return left == null ? this.key : left.findMin();
}
public int compare(NodeBinarno node) {
counter++;
return node.key - this.key;
}
public int getCounter() {
return counter;
}
public void resetCounter() {
counter=0;
}
} i forgot this part of the code rewrite this part too
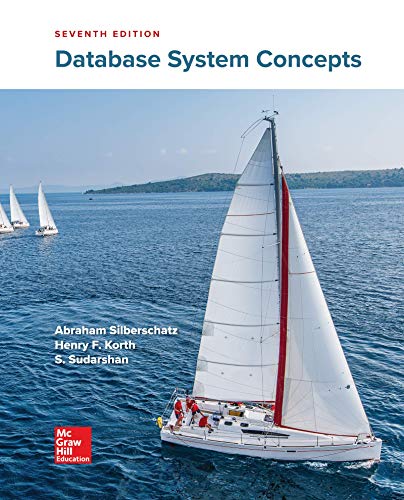
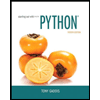
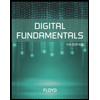
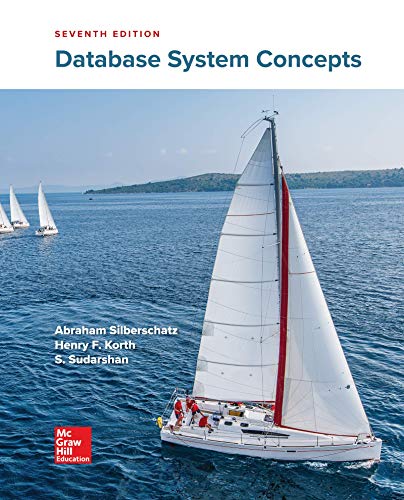
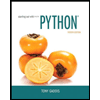
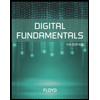
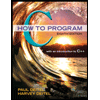
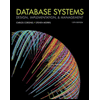
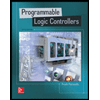