void findMostAndLeastOccurrence(occurrences, size, highestCountIndex, lowestCountindex); Parameters: occurrences: array of integers size: array size highestCountindex: subscript of array element with the highest occurrence count, passed by reference lowestCountindex: subscript of array element with the lowest occurrence count, passed by reference This function find the number with the most and the number with the least occurrence in the array It does not print anything (no "cout" in here, main function and printCounts function does the printing) int findTotalOccurrenceCount(occurrences, size) Parameters: occurrences: array of integers size: array size Function adds up all the numbers in the occurrences array and returns the total value It does not print anything (no "cout" in here, main function and printCounts function does the printing) The main() function does: Ask user to enter a file name - Open the given file and check for error. If file does not exists, display error message "File XXXXXX not found" and exit program. - Ask user to enter input inputMax - Use an input validation loop to check that inputMax cannot be less than 1. - Define and initialize the array of size inputMax - Open the file as input file - Call the function readNumbersAndTallyOccurrences( ) to read all data and tally the occurrence counts. - Close the file Call the function printCounts() to display the occurrence counts of all numbers from 1 up to inputMax. Call the function findTotalOccurrenceCount() to get the total occurrence count. - Display the total occurrence count. - Call the function findMostAndLeastOccurrence() to find the number with highest and the number with the lowest occurrence count. - Display the number with the highest and the number with the lowest occurrence count Hint: 1. You should store the occurrence count of 1 in the first element (subscript 0) of the frequency array, occurrence count 2 in the second element of array (subscript 1),. 2. Use a simple arithmetic expression to calculate the array subscript from the number that is read from the file only if the number is <= maxlnput. Do not use a gigantic "if" statement to get the array subscript. 3. Remember the rules about array subscript range. 4. There should be only ONE array of size maxlnput (number values are from 1 to certain value > 1) to be defined in main(). The array is passed as a parameter to other functions. 5. You should use function prototypes before main() 6. If there are multiple numbers with the same highest occurrence count or the same lowest occurrence count, use only the first number.
void findMostAndLeastOccurrence(occurrences, size, highestCountIndex, lowestCountindex); Parameters: occurrences: array of integers size: array size highestCountindex: subscript of array element with the highest occurrence count, passed by reference lowestCountindex: subscript of array element with the lowest occurrence count, passed by reference This function find the number with the most and the number with the least occurrence in the array It does not print anything (no "cout" in here, main function and printCounts function does the printing) int findTotalOccurrenceCount(occurrences, size) Parameters: occurrences: array of integers size: array size Function adds up all the numbers in the occurrences array and returns the total value It does not print anything (no "cout" in here, main function and printCounts function does the printing) The main() function does: Ask user to enter a file name - Open the given file and check for error. If file does not exists, display error message "File XXXXXX not found" and exit program. - Ask user to enter input inputMax - Use an input validation loop to check that inputMax cannot be less than 1. - Define and initialize the array of size inputMax - Open the file as input file - Call the function readNumbersAndTallyOccurrences( ) to read all data and tally the occurrence counts. - Close the file Call the function printCounts() to display the occurrence counts of all numbers from 1 up to inputMax. Call the function findTotalOccurrenceCount() to get the total occurrence count. - Display the total occurrence count. - Call the function findMostAndLeastOccurrence() to find the number with highest and the number with the lowest occurrence count. - Display the number with the highest and the number with the lowest occurrence count Hint: 1. You should store the occurrence count of 1 in the first element (subscript 0) of the frequency array, occurrence count 2 in the second element of array (subscript 1),. 2. Use a simple arithmetic expression to calculate the array subscript from the number that is read from the file only if the number is <= maxlnput. Do not use a gigantic "if" statement to get the array subscript. 3. Remember the rules about array subscript range. 4. There should be only ONE array of size maxlnput (number values are from 1 to certain value > 1) to be defined in main(). The array is passed as a parameter to other functions. 5. You should use function prototypes before main() 6. If there are multiple numbers with the same highest occurrence count or the same lowest occurrence count, use only the first number.
Chapter13: File Input And Output
Section: Chapter Questions
Problem 6PE
Related questions
Question
input files are random numbers
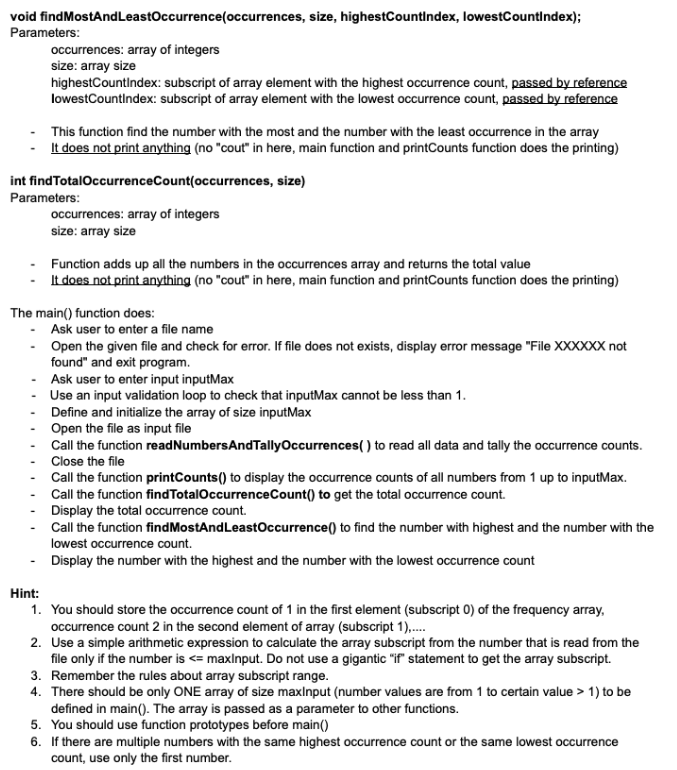
Transcribed Image Text:void findMostAndLeastOccurrence(occurrences, size, highestCountindex, lowestCountindex);
Parameters:
occurrences: array of integers
size: array size
highestCountindex: subscript of array element with the highest occurrence count, passed by reference
lowestCountindex: subscript of array element with the lowest occurrence count, passed by reference
- This function find the number with the most and the number with the least occurrence in the array
It does not print anything (no "cout" in here, main function and printCounts function does the printing)
int findTotalOccurrenceCount(occurrences, size)
Parameters:
occurrences: array of integers
size: array size
Function adds up all the numbers in the occurrences array and returns the total value
- It does not print anything (no "cout" in here, main function and printCounts function does the printing)
The main() function does:
- Ask user to enter a file name
- Open the given file and check for error. If file does not exists, display error message "File XXXXXX not
found" and exit program.
- Ask user to enter input inputMax
Use an input validation loop to check that inputMax cannot be less than 1.
Define and initialize the array of size inputMax
Open the file as input file
Call the function readNumbersAndTallyOccurrences( ) to read all data and tally the occurrence counts.
- Close the file
Call the function printCounts() to display the occurrence counts of all numbers from 1 up to inputMax.
- Call the function findTotalOccurrenceCount() to get the total occurrence count.
- Display the total occurrence count.
- Call the function findMostAndLeastOccurrence() to find the number with highest and the number with the
lowest occurrence count.
- Display the number with the highest and the number with the lowest occurrence count
Hint:
1. You should store the occurrence count of 1 in the first element (subscript 0) of the frequency array,
occurrence count 2 in the second element of array (subscript 1).,.
2. Use a simple arithmetic expression to calculate the array subscript from the number that is read from the
file only if the number is <= maxlnput. Do not use a gigantic "if" statement to get the array subscript.
3. Remember the rules about array subscript range.
4. There should be only ONE array of size maxlnput (number values are from 1 to certain value > 1) to be
defined in main(). The array is passed as a parameter to other functions.
5. You should use function prototypes before main()
6. If there are multiple numbers with the same highest occurrence count or the same lowest occurrence
count, use only the first number.
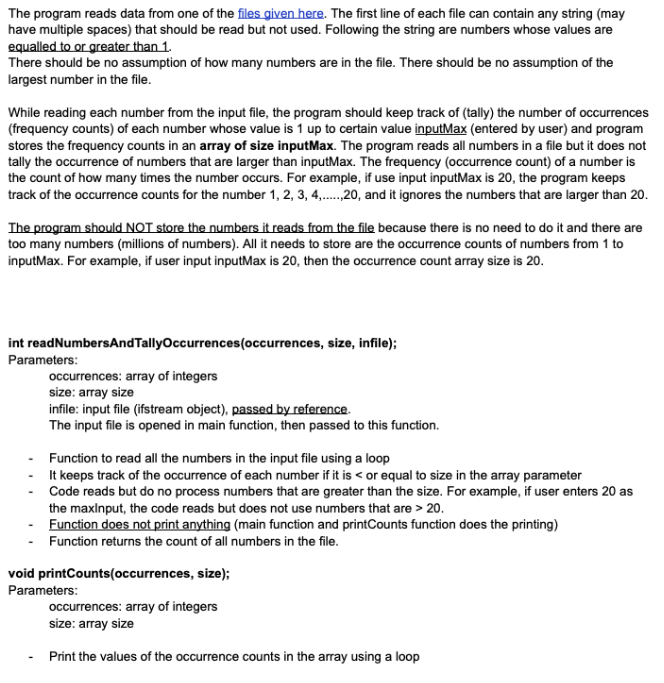
Transcribed Image Text:The program reads data from one of the files given here. The first line of each file can contain any string (may
have multiple spaces) that should be read but not used. Following the string are numbers whose values are
equalled to or greater than 1.
There should be no assumption of how many numbers are in the file. There should be no assumption of the
largest number in the file.
While reading each number from the input file, the program should keep track of (tally) the number of occurrences
(frequency counts) of each number whose value is 1 up to certain value inputMax (entered by user) and program
stores the frequency counts in an array of size inputMax. The program reads all numbers in a file but it does not
tally the occurrence of numbers that are larger than inputMax. The frequency (occurrence count) of a number is
the count of how many times the number occurs. For example, if use input inputMax is 20, the program keeps
track of the occurrence counts for the number 1, 2, 3, 4,.20, and it ignores the numbers that are larger than 20.
The program should NOT store the numbers it reads from the file because there is no need to do it and there are
too many numbers (millions of numbers). All it needs to store are the occurrence counts of numbers from 1 to
inputMax. For example, if user input inputMax is 20, then the occurrence count array size is 20.
int readNumbersAndTallyOccurrences(occurrences, size, infile);
Parameters:
occurrences: array of integers
size: array size
infile: input file (ifstream object), passed by reference.
The input file is opened in main function, then passed to this function.
Function to read all the numbers in the input file using a loop
It keeps track of the occurrence of each number if it is < or equal to size in the array parameter
Code reads but do no process numbers that are greater than the size. For example, if user enters 20 as
the maxlnput, the code reads but does not use numbers that are > 20.
Function does not print anything (main function and printCounts function does the printing)
Function returns the count of all numbers in the file.
void printCounts(occurrences, size);
Parameters:
occurrences: array of integers
size: array size
Print the values of the occurrence counts in the array using a loop
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
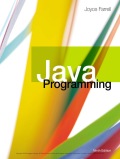
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
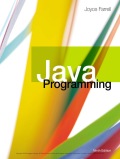
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT