Part 1: Encryption A. Using your FileReader, read the file "PlentyOfRoomAtTheBottom.txt" in the Labs Module. There are non-printable characters in the file. Sometimes FileReaders abort when encountering a non-printable or extended ASCII characters. Handle this with exception handling if it happens. Your program should continue processing instead of aborting. Assign a specific character, of your choice, to the aborted character. B. Using the rawdata from reading the "PlentyOfRoomAtTheBottom.txt", develop a frequency counter algorithm for each character the lower ASCII table. For example: Character[ a ] = Frequency[ 2475 ] Character[ b ] = Frequency[ 420 ] Character[ c ] = Frequency[ 985 ] C. Sort the Lower ASCII frequency characters (i.e. 1 to 128) in decending order and process the data by using your QTree algorithm. Your QTree algorithm should be based on a PRIORITY_QUEUE. D. Create bit-trails, as defined in the Exercise Bit-Trail, for each character your QTree. E. For each character in the raw data file from part A, "lookup" each character in your QTree tuple list using your Tuple Search algorithm from Exercise Tuple and write the character's bit-trail in a file. This encrypts the file in binary. Part 2: Decryption A. For each encrypted character in the encrypted binary file, for example "01010010", walk the QTree by looping through the branches. B. Start at the root of the QTree and "walk" the bit-trail until a Leaf Node is found. C. A zero goes down the left branch and a one goes down the right branch. The found Leaf Node will contain the Node.symbol at the end of the bit-trail. D. Write the Node.symbol to a decrypted file. When complete, your original file, the PlentyOfRoomAtTheBottom.txt, will be decrypted and readable as plain text. E. In cases where the decrypted text is not readable, this implies your QTree is not constructed properly OR the bit-trail path is incorrect. You will need to debug your QTree and Bit-Trail algorithms. PlentyOfRoomAtTheBottom.txt "There's Plenty of Room at the Bottom by Richard P. Feynman I imagine experimental physicists must often look with envy at men like Kamerlingh Onnes, who discovered a field like low temperature, which seems to be bottomless and in which one can go down and down. Such a man is then a leader and has some temporary monopoly in a scientific adventure. Percy Bridgman, in designing a way to obtain higher pressures, opened up another new field and was able to move into it and to lead us all along. The development of ever higher vacuum was a continuing development of the same kind. I would like to describe a field, in which little has been done, but in which an enormous amount can be done in principle. This field is not quite the same as the others in that it will not tell us much of fundamental physics (in the sense of, "What are the strange particles?") but it is more like solid-state physics in the sense that it might tell us much of great interest about the strange phenomena that occur in complex situations. Furthermore, a point that is most important is that it would have an enormous number of technical applications. What I want to talk about is the problem of manipulating and controlling things on a small scale. As soon as I mention this, people tell me about miniaturization, and how far it has progressed today. They tell me about electric motors that are the size of the nail on your small finger. And there is a device on the market, they tell me, by which you can write the Lord's Prayer on the head of a pin. But that's nothing; that's the most primitive, halting step in the direction I intend to discuss. It is a staggeringly small world that is below. In the year 2000, when they look back at this age, they will wonder why it was not until the year 1960 that anybody began seriously to move in this direction. Why cannot we write the entire 24 volumes of the Encyclopaedia Brittanica on the head of a pin? Let's see what would be involved. The head of a pin is a sixteenth of an inch across. If you magnify it by 25,000 diameters, the area of the head of the pin is then equal to the area of all the pages of the Encyclopaedia Brittanica. Therefore, all it is necessary to do is to reduce in size all the writing in the Encyclopaedia by 25,000 times. Is that possible? " Please write in C++, with your code. Just write the code that encrypt the file into binary, then decrypt it back into letters (it means the expected output would be the same with file .txt above)
C. Sort the Lower ASCII frequency characters (i.e. 1 to 128) in decending order and process the data by using your QTree algorithm. Your QTree algorithm should be based on a PRIORITY_QUEUE.
D. Create bit-trails, as defined in the Exercise Bit-Trail, for each character your QTree.
E. For each character in the raw data file from part A, "lookup" each character in your QTree tuple list using your Tuple Search algorithm from Exercise Tuple and write the character's bit-trail in a file. This encrypts the file in binary.
Part 2: Decryption
A. For each encrypted character in the encrypted binary file, for example "01010010", walk the QTree by looping through the branches.
B. Start at the root of the QTree and "walk" the bit-trail until a Leaf Node is found.
C. A zero goes down the left branch and a one goes down the right branch. The found Leaf Node will contain the Node.symbol at the end of the bit-trail.
D. Write the Node.symbol to a decrypted file. When complete, your original file, the PlentyOfRoomAtTheBottom.txt, will be decrypted and readable as plain text.
E. In cases where the decrypted text is not readable, this implies your QTree is not constructed properly OR the bit-trail path is incorrect. You will need to debug your QTree and Bit-Trail algorithms.
PlentyOfRoomAtTheBottom.txt
"There's Plenty of Room at the Bottom
by Richard P. Feynman
I imagine experimental physicists must often look with envy at men like Kamerlingh Onnes, who discovered a field like low temperature, which seems to be bottomless and in which one can go down and down. Such a man is then a leader and has some temporary monopoly in a scientific adventure. Percy Bridgman, in designing a way to obtain higher pressures, opened up another new field and was able to move into it and to lead us all along. The development of ever higher vacuum was a continuing development of the same kind.
I would like to describe a field, in which little has been done, but in which an enormous amount can be done in principle. This field is not quite the same as the others in that it will not tell us much of fundamental physics (in the sense of, "What are the strange particles?") but it is more like solid-state physics in the sense that it might tell us much of great interest about the strange phenomena that occur in complex situations. Furthermore, a point that is most important is that it would have an enormous number of technical applications.
What I want to talk about is the problem of manipulating and controlling things on a small scale.
As soon as I mention this, people tell me about miniaturization, and how far it has progressed today. They tell me about electric motors that are the size of the nail on your small finger. And there is a device on the market, they tell me, by which you can write the Lord's Prayer on the head of a pin. But that's nothing; that's the most primitive, halting step in the direction I intend to discuss. It is a staggeringly small world that is below. In the year 2000, when they look back at this age, they will wonder why it was not until the year 1960 that anybody began seriously to move in this direction.
Why cannot we write the entire 24 volumes of the Encyclopaedia Brittanica on the head of a pin?
Let's see what would be involved. The head of a pin is a sixteenth of an inch across. If you magnify it by 25,000 diameters, the area of the head of the pin is then equal to the area of all the pages of the Encyclopaedia Brittanica. Therefore, all it is necessary to do is to reduce in size all the writing in the Encyclopaedia by 25,000 times. Is that possible? "
Please write in C++, with your code. Just write the code that encrypt the file into binary, then decrypt it back into letters (it means the expected output would be the same with file .txt above)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

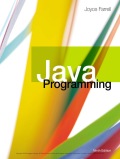
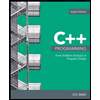
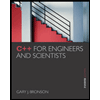
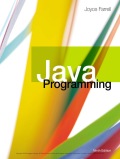
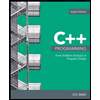
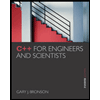
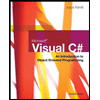