Please answer in C++ and have at least two string functions. Your country is at war and your enemies are using a secret code to communicate with one another. You have managed to intercept a message that reads as follows: :mmZ\dxZmx]Zpgy The message is obviously encrypted using the enemy's secret code. You have just learned that their encryption method is based upon the ASCII code. Appendix 3 shows the character set. Individual characters in string are encoded using this system. For example, the character "A" is encoded using the number 65, and "B" is encoded using the number 66. Your enemy's secret code takes each letter of the message and encrypts it as follows : if (orginalChar + key > 126) then encryptedChar = 32 + ((orginalChar + key) -127) else encryptedChar = (orginalChar +key) For example, if the enemy uses key = 10 then the message "Hey" would be encrypted as: Character ASCII code H 72 e 101 y 121 Encrypted H = (72 +10) = 82 = R in ASCII Encrypted e = (101 +10) = 111 = o in ASCII Encrypted y = 32 + ((121 + 10) - 127) = 36 = $ in ASCII Consequently, "Hey" would be transmitted as "Ro$." Write a program that decrypts the intercepted message. The ASCII codes for the unencrypted message are limited to the visible ASCII characters. You only know that the key used is a number between 1 and 100. Your program should try to decode the message using all possible keys between 100. When you try the valid key, the message will make sense. For all other keys, the message will appear as gibberish.
Please answer in C++ and have at least two string functions. Your country is at war and your enemies are using a secret code to communicate with one another. You have managed to intercept a message that reads as follows: :mmZ\dxZmx]Zpgy The message is obviously encrypted using the enemy's secret code. You have just learned that their encryption method is based upon the ASCII code. Appendix 3 shows the character set. Individual characters in string are encoded using this system. For example, the character "A" is encoded using the number 65, and "B" is encoded using the number 66. Your enemy's secret code takes each letter of the message and encrypts it as follows : if (orginalChar + key > 126) then encryptedChar = 32 + ((orginalChar + key) -127) else encryptedChar = (orginalChar +key) For example, if the enemy uses key = 10 then the message "Hey" would be encrypted as: Character ASCII code H 72 e 101 y 121 Encrypted H = (72 +10) = 82 = R in ASCII Encrypted e = (101 +10) = 111 = o in ASCII Encrypted y = 32 + ((121 + 10) - 127) = 36 = $ in ASCII Consequently, "Hey" would be transmitted as "Ro$." Write a program that decrypts the intercepted message. The ASCII codes for the unencrypted message are limited to the visible ASCII characters. You only know that the key used is a number between 1 and 100. Your program should try to decode the message using all possible keys between 100. When you try the valid key, the message will make sense. For all other keys, the message will appear as gibberish.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter4: Selection Structures
Section4.3: Nested If Statements
Problem 7E
Related questions
Topic Video
Question
Please answer in C++ and have at least two string functions.
Your country is at war and your enemies are using a secret code to communicate with one another. You have managed to intercept a message that reads as follows:
:mmZ\dxZmx]Zpgy
The message is obviously encrypted using the enemy's secret code. You have just learned that their encryption method is based upon the ASCII code. Appendix 3 shows the character set. Individual characters in string are encoded using this system. For example, the character "A" is encoded using the number 65, and "B" is encoded using the number 66.
Your enemy's secret code takes each letter of the message and encrypts it as follows :
if (orginalChar + key > 126) then
encryptedChar = 32 + ((orginalChar + key) -127)
else
encryptedChar = (orginalChar +key)
For example, if the enemy uses key = 10 then the message "Hey" would be encrypted as:
Character ASCII code
H 72
e 101
y 121
Encrypted H = (72 +10) = 82 = R in ASCII
Encrypted e = (101 +10) = 111 = o in ASCII
Encrypted y = 32 + ((121 + 10) - 127) = 36 = $ in ASCII
Consequently, "Hey" would be transmitted as "Ro$."
Write a program that decrypts the intercepted message. The ASCII codes for the unencrypted message are limited to the visible ASCII characters. You only know that the key used is a number between 1 and 100. Your program should try to decode the message using all possible keys between 100. When you try the valid key, the message will make sense. For all other keys, the message will appear as gibberish.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
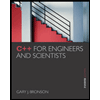
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
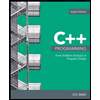
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
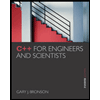
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
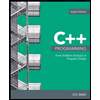
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning