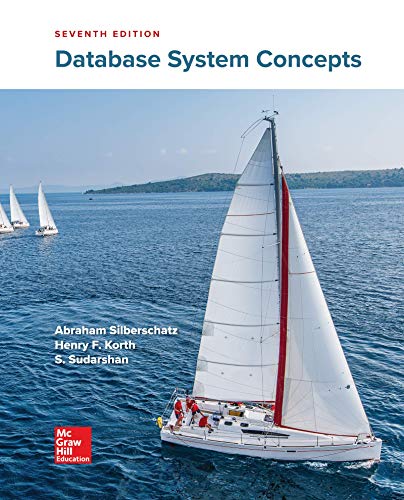
Write a C program that reads integer values from stdin, separated by one or more spaces or newlines, until reaching EOF.
Once we have entered all our integers, we may have to press Enter before pressing Ctrl+d.
This signifies that we do not have to create a function, we can implement this vertical histogram
program within our main(...) function.
Save your program as verticalHistogram.c
-
The input contains no more than 80 values.
Why? Because a standard terminal window is 80 character-columns across. -
The input is guaranteed to be well-formed.
-
On standard output (stdout), it renders a simple vertical column graph representation of the input values (i.e., a vertical histogram), in order left to right, using hash '#' characters as shown in the examples on the next slides. The number of hashes '#' printed in each column
should be equal to the corresponding input value.
The area above a completed column should be filled with space characters.
Ignore empty lines in the input. Do not output a column for an empty line in the input.
The entire graph must end with a newline character. Hint:
In order to plot a histogram vertically, we need to have finished reading all the input before we start drawing the histogram on stdout. An array is suitable for this.
If we limit the number of columns we can graph to a maximum of 80, we know how large an array we need to allocate and this program is simple to write.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Write a c++ program that includes two functions for sorting integers. your program must acts as following: Ask the user to enter 5 numbers and then by calling Asc() and Dsc() functions sort these 5 numbers in ascending and descending orders. Sample output: How many integers you wants to sort? 5 Please enter 5 integers: 5 8 -1 0 2 the ascending order of your list is: -1 0 2 5 8 The Descending order of your list is: 8 5 2 0 -1 Note: to do the above question do not use the array , vector or class. Use swaparrow_forwardWrite a function to determine the resultant force vector R of the two forces F₁ and F₂ applied to the bracket, where 0₁ (positive, unit in degree) and ₂ (negative, unit in degree). Write R in terms of unit vector along the and y axis. R must be a vector, for example R = [R₂, R₂]. Note that is expressed in degrees. The coordinate system is shown in the figure below: F2 021 return R # import numpy as np. import math from math import * # Import all the math functions from the math library from numpy import array # Complete the function given the variables F1, F2, thetal (positive, degree), theta2 (negative, degree) and return the value as array #Hints: Use numpy.array to form the force vector and then add the force vectors # Remember to convert the degree to radian in the calculations and be careful of the sign def force_vec (F1, F2, thetal, theta2): R=np.array([0,0]) # Resultant force vector, Initiation of the value # YOUR CODE HERE # Check your answer F1=300 F2=200 0₁ Theta1=60…arrow_forwardCreate a function that determines whether each seat can "see" the front-stage. A number can "see" the front-stage if it is strictly greater than the number before it. Everyone can see the front-stage in the example below: # FRONT STAGE [[1, 2, 3, 2, 1, 1], [2, 4, 4, 3, 2, 2], [5, 5, 5, 5, 4, 4], [6, 6, 7, 6, 5, 5]] # Starting from the left, the 6 > 5 > 2 > 1, so all numbe # 6 > 5 > 4 > 2 - so all numbers can see, etc. Not everyone can see the front-stage in the example below: # FRONT STAGE [[1, 2, 3, 2, 1, 1], [2, 4, 4, 3, 2, 2], [5, 5, 5, 10, 4, 4], [6, 6, 7, 6, 5, 5]] # The 10 is directly in front of the 6 and blocking its varrow_forward
- Write a c++ program that includes two functions for sorting integers: Ask user to enter 5 numbers and then by calling Asc() and Dsc() functions sort these 5 numbers in ascending and descending orders. Sample output: How many integers you wants to sort? 5 Please enter 5 integers: 5 8 -1 0 2 the ascending order of your list is: -1 0 2 5 8 The Descending order of your list is: 8 5 2 0 -1 Note: Do not use the array.arrow_forwardComplete the rotate_text() function that takes 2 parameters, a string data and an integer n. If n is positive, then the function will shift all the characters in data forward by n positions, with characters at the end of the string being moved to the start of the string. If n is 0 then the text remains the same. For example: rotate_text('abcde', rotate_text('abcde', rotate_text('abcde', 1) would return the string 'eabcd' 3) would return the string 'cdeab' 5) would return the string 'abcde' rotate_text('abcde', 6) would return the string 'eabcd' ... and so on. If n is negative, then the function will shift the characters in data backward by n positions, with characters at the start of the string being moved to the end of the string. For example: rotate text('abcde', -1) would return the string 'bcdea'arrow_forwardPython Write a function called count_dashes that takes a line of text as inputand outputs the number of dashes in it, as shown below. In addition to printing the requested information, return the number of dashes. The function should output (print) its findings as follows:There are DDD dashes in 'XXX'.where XXX and DDD are replaced by the appropriate values that are based on the given argument.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
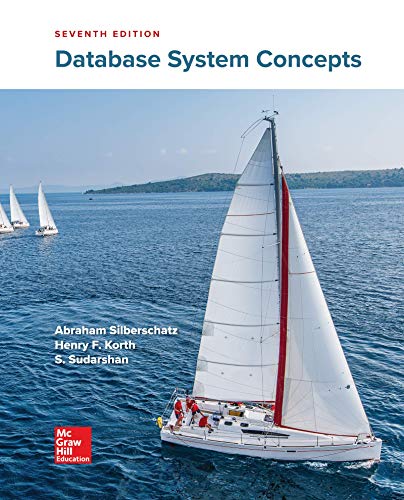
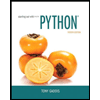
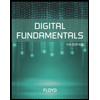
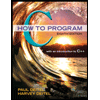
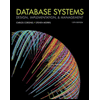
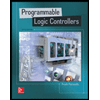