Please do not change any of the method signatures in either class. Implement the methods described below. RadixSort.java RadixSort.java contains two different RadixSort implementations each using a different version of Counting Sort that you will implement. As a reminder the pseudocode for CountingSort discussed in class is as follows. countingSort(arr, n, k) 1. let B[1 : n] and C[0 : k] be new arrays 2. for i = 0 to k 3. C[i] = 0 4. for j = 1 to n 5 . C[arr[j]] = C [arr[j]] + 1 6. for i = 1 to k 7. C[i] = C[i] + C[i – 1] 8. for j = n downto 1 9. B[C[arr[j]]] = arr[j] 10 C[arr[j]] = C[arr[j]] – 1 11. return B private static void countingSort1(int[] arr, int place) For this method you will implement the countingSort algorithm. Note that this implementation is specific to RadixSort and assumes that k=10 and the int place parameter represents which column is currently being sorted (1s, 10s, 100s, etc.). Note that you can use the pseudocode above as a guide, but you will need to think about the correct math to ensure that you are sorting on the right significant digit in the array. You can test your method independently and using the RadixSort1 function. this is the method signature class: package sorting; import java.util.Arrays; public class RadixSort { //returns the max value in the array //used to provide the k to counting sort private static int getMax(int[] arr) { int max = Integer.MIN_VALUE; for(int i: arr) { if(i>max) max = i; } return max; } private static void countingSort1(int[] arr, int place) { int[] output = new int[arr.length]; int[] count = new int[10]; Arrays.fill(count, 0); /**********YOUR CODE GOES HERE***********/ //Reorder the original array using the output array for (int i = 0; i < arr.length; i++) arr[i] = output[i]; } public static void radixSort1(int[] arr) { //Get the maximum to know how many digits I have int max = getMax(arr); //Applies counting sort to each place starting with the 1s place for (int place = 1; max / place > 0; place *= 10) countingSort1(arr, place); }
Please do not change any of the method signatures in either class. Implement the methods described below.
RadixSort.java
RadixSort.java contains two different RadixSort implementations each using a different version of Counting Sort that you will implement. As a reminder the pseudocode for CountingSort discussed in class is as follows.
countingSort(arr, n, k)
1. let B[1 : n] and C[0 : k] be new arrays
2. for i = 0 to k
3. C[i] = 0
4. for j = 1 to n
5 . C[arr[j]] = C [arr[j]] + 1
6. for i = 1 to k
7. C[i] = C[i] + C[i – 1]
8. for j = n downto 1
9. B[C[arr[j]]] = arr[j]
10 C[arr[j]] = C[arr[j]] – 1
11. return B
private static void countingSort1(int[] arr, int place)
For this method you will implement the countingSort
You can test your method independently and using the RadixSort1 function.
this is the method signature class:
package sorting;
import java.util.Arrays;
public class RadixSort {
//returns the max value in the array
//used to provide the k to counting sort
private static int getMax(int[] arr) {
int max = Integer.MIN_VALUE;
for(int i: arr) {
if(i>max)
max = i;
}
return max;
}
private static void countingSort1(int[] arr, int place) {
int[] output = new int[arr.length];
int[] count = new int[10];
Arrays.fill(count, 0);
/**********YOUR CODE GOES HERE***********/
//Reorder the original array using the output array
for (int i = 0; i < arr.length; i++)
arr[i] = output[i];
}
public static void radixSort1(int[] arr) {
//Get the maximum to know how many digits I have
int max = getMax(arr);
//Applies counting sort to each place starting with the 1s place
for (int place = 1; max / place > 0; place *= 10)
countingSort1(arr, place);
}

Step by step
Solved in 3 steps with 1 images

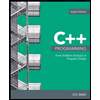
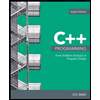