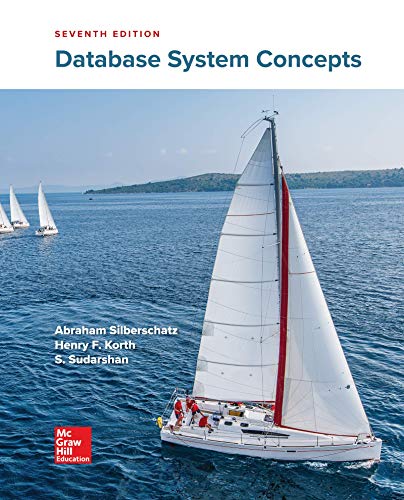
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write this program in Java using a custom method.
Implementation details
You will implement this program in a specific way in order to gain some experience with loops, arrays and array lists.
- Use an array of strings to store the 4 strings listed in the description.
- Use a do-while loop for your 'game engine'. This means the game starts once the user enters money. The decision to stop occurs at the bottom of the loop. The do-while loop keeps going until the user quits, or there is no money left. The pseudocode for this 'game engine' is shown below:
- determine the fruits to display (step 3 below) and print them
- determine if there are 3 or 4 of the same image
- display the results
- update the customer balance as necessary
- prompt to play or quit
- continue loop if customer wants to play and there's money for another game.
- Use the Random class to generate a random number between 0 and 3. This random number will be an index into the array of strings. Add the string at that index to an ArrayList. You'll have to do this four times. When done, your ArrayList will contain 4 strings that represent randomly selected fruit images.
- Write a custom method that is called within your do-while loop. This method will accept the array list as a parameter, and it will return an integer that represents the number of times an 'fruit' appeared in the array list.
- Inside this method, use the frequency method from the Collections class to determine how many times a particular value is in a ArrayList. You'll have to call Collections.frequency for each image to get the counts by image. You can see an example of using this method on page 794 in your text. Note that this is an exception to our rule about using just the techniques in our chapters because I want you to be aware of the Collections class and how it can be helpful in certain situations.
- If your custom method returns a three, then add $2 to the balance. If it returns a four, then add $10 to the customer's balance.
- Your code should display the contents of the arraylist on the same line with spaces between them.
- Your code should display the results of the play -- see sample output -- and the current balance.
- At the bottom of the do-while loop, prompt the user if they want to continue to play (P) or to quit (Q). Case should not matter. It's OK if the user enters a character other than those two characters. Treat anything other than a 'Q' as a play.
Sample Output
Enter dollar amount to play ==> 5
Apple Apple Orange Orange
<< Not a winner this time >>
Balance is: $4
Play Again (P) or Quit (Q) p
Banana Banana Orange Cherry
<< Not a winner this time >>
Balance is: $3
Play Again (P) or Quit (Q) p
Banana Apple Banana Banana
<< You matched 3! That's $2 >>
Balance is: $5
Play Again (P) or Quit (Q) q
Thanks for playing! Cash Balance is $5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Validating User Input Summary In this lab, you will make additions to a Java program that is provided. The program is a guessing game. A random number between 1 and 10 is generated in the program. The user enters a number between 1 and 10, trying to guess the correct number. If the user guesses correctly, the program congratulates the user, and then the loop that controls guessing numbers exits; otherwise, the program asks the user if he or she wants to guess again. If the user enters a "Y", he or she can guess again. If the user enters "N", the loop exits. You can see that the "Y" or an "N" is the sentinel value that controls the loop. Note that the entire program has been written for you. You need to add code that validates correct input, which is "Y" or "N", when the user is asked if he or she wants to guess a number, and a number in the range of 1 through 10 when the user is asked to guess a number. Instructions Ensure the file named GuessNumber.java is open. Write loops…arrow_forwardDecrease-by-Constant-Factor Fake-Coin puzzle method in Java or C++ to find the fake coin out of n coins. Assume the false coin is lighter. Randomly place the false coin among the n coins. Submit results images and code files.arrow_forwardA for construct is a loop that processes a list of items. As a consequence, it continues to run as long as there are items to process. Is this statement true or false?arrow_forward
- Overview In this assignment, you will gain more practice with designing a program. Specifically, you will create pseudocode for a higher/lower game. This will give you practice designing a more complex program and allow you to see more of the benefits that designing before coding can offer. The higher/lower game will combine different programming constructs that you have been learning about, such as input and output, decision branching, and a loop. Higher/Lower Game DescriptionYour friend Maria has come to you and said that she has been playing the higher/lower game with her three-year-old daughter Bella. Maria tells Bella that she is thinking of a number between 1 and 10, and then Bella tries to guess the number. When Bella guesses a number, Maria tells her whether the number she is thinking of is higher or lower or if Bella guessed it. The game continues until Bella guesses the right number. As much as Maria likes playing the game with Bella, Bella is very excited to play the game…arrow_forwardC#Write a method MyMethod which takes an int parameter named x. This method calculates the sum of integers from 1 to x and returns the sum. Use a for loop.arrow_forwardUsing a Sentinel Value to Control a while Loop in Java Summary In this lab, you write a while loop that uses a sentinel value to control a loop in a Java program that has been provided. You also write the statements that make up the body of the loop. The source code file already contains the necessary variable declarations and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Ensure the file named MovieGuide.java is open. Write the while loop using a sentinel value to control the loop, and write the statements that make up the body of the loop to calculate the average star rating. Execute the program by clicking Run. Input the following: 0, 3, 4, 4, 1, 1, 2, -1 Check that the…arrow_forward
- flow chart and dimple program. Python thank youarrow_forwardAccumulating Totals in a Loop Summary In this lab, you add a loop and the statements that make up the loop body to a Java program that is provided. When completed, the program should calculate two totals: the number of left-handed people and the number of right-handed people in your class. Your loop should execute until the user enters the character X instead of L for left-handed or R for right-handed. The inputs for this program are as follows: R, R, R, L, L, L, R, L, R, R, L, X Variables have been declared for you, and the input and output statements have been written. Instructions Ensure the file named LeftOrRight.java is open. Write a loop and a loop body that allows you to calculate a total of left-handed and right-handed people in your class. Execute the program by clicking Run and using the data listed above and verify that the output is correct.arrow_forwardPrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forward
- Computer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forwardUsing jGRASP please de-bug this /**This program demonstrates an array of String objects.It should print out the days and the number of hours spent at work each day.It should print out the day of the week with the most hours workedIt should print out the average number of hours worked each dayThis is what should display when the program runs as it shouldSunday has 12 hours worked.Monday has 9 hours worked.Tuesday has 8 hours worked.Wednesday has 13 hours worked.Thursday has 6 hours worked.Friday has 4 hours worked.Saturday has 0 hours worked.Highest Day is Wednesday with 13 hours workedAverage hours worked in the week is 7.43 hours*/{public class WorkDays{public static void main(String[] args){String[] days = { "Sunday", "Monday", "Tuesday","Wednesday", "Thursday", "Friday", "Saturday"}; int[] hours = { 12, 9, 8, 13, 6, 4}; int average = 0.0;int highest = 0;String highestDay = " ";int sum = 0; for (int index = 0; index < days.length; index++){System.out.println(days[index] + " has "…arrow_forwardJava Calculating the User's Sum Write a complete program that reads in numbers and calculates the sum of the numbers in that range. Code Specifications In the main method, read input from the user. Read in two integers from the user: a lower end of the range and an upper end of the range. Check if the numbers are valid: the lower number cannot be greater than the upper number. If the numbers are invalid, use a loop to ask for new numbers. Continue looping until you get two valid values. Write a method called calculateTheSum. The method takes in a lower and upper end of the range. The method calculates the sum of all values from lower (inclusive) to upper (inclusive). Invoke the method from main the output the result. Test Cases I recommend testing your code using the test cases below. I've listed the user inputs along with a sample of the result. User Inputs Result lower = 10, upper = 1 the program should ask for new input lower = 5, upper = -5 the program should ask…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
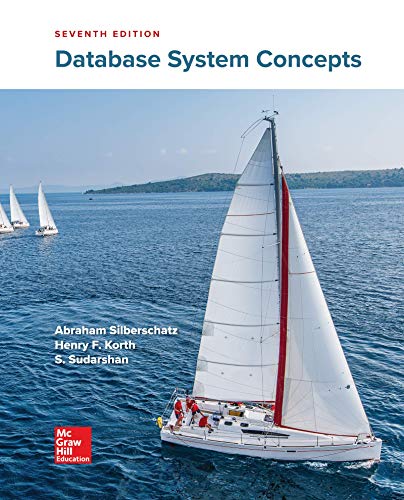
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
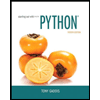
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
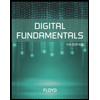
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
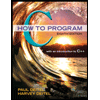
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
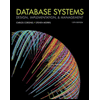
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
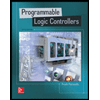
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education