Please follow a consistent coding style (indentation, variable names, etc.) with comments. You are not allowed to use STL containers. You may (and should) implement any getter and setter methods when needed. Make use of constants and call by reference appropriately. Use cerr for printing error messages cout. Prin messages to the terminal. Do not create a .txt file to print into. Your codes will be tested with Calico. Therefore your outputs should be exactly same with the given example output. To make sure about your output correctness, please checkout the supplied "output.txt" file. All available print messages are available in the "output.txt".
Implementation Notes: Please follow a consistent coding style (indentation, variable names, etc.) with comments.
-
You are not allowed to use STL containers.
-
You may (and should) implement any getter and setter methods when needed.
-
Make use of constants and call by reference appropriately.
-
Use cerr for printing error messages cout.
-
Prin messages to the terminal. Do not create a .txt file to print into.
-
Your codes will be tested with Calico. Therefore your outputs should be exactly same with the given example output. To make sure about your output correctness, please checkout the supplied "output.txt" file. All available print messages are available in the "output.txt".
main.cpp file:
#include "Person.h"
#include "Mission.h"
#include "Agency.h"
#include <iostream>
#include <cstdlib>
using namespace std;
// Initialize the static member
int Mission::numMissions = 0;
int main(int argc, char const *argv[])
{
// Set the PRNG seed
srand(1773);
cout << "*** Agency creation ***" << endl;
// Create agency with given name, cash and ticket price
Agency newAgency = Agency("NASA",20000,-40);
cout << newAgency;
// Set the ticket price which is set to 0 before
newAgency.setTicketPrice(10000);
// Print agency information
cout << newAgency;
// Execute the next mission
newAgency.executeNextMission();
cout << endl << "*** Astronaut creation ***" << endl;
// Create astronauts
Astronaut* astronautList[5];
astronautList[0] = new Astronaut("Neil","Armstrong",3);
astronautList[1] = new Astronaut("Buzz","Aldrin",-4);
astronautList[2] = new Astronaut("Sally","Ride");
astronautList[3] = new Astronaut("Judith","Resnik",4);
astronautList[4] = new Astronaut("Yuri","Gagarin",5);
cout << endl << "*** Passenger creation ***" << endl;
// Create astronauts
Passenger* passengerList[5];
passengerList[0] = new Passenger("Dennis","Tito",12000);
passengerList[1] = new Passenger("Mark","Shuttleworth",10000);
passengerList[2] = new Passenger("Gregory","Olsen",15000);
passengerList[3] = new Passenger("Charles","Simonyi",24000);
passengerList[4] = new Passenger("Alperen","Kantarcı");
// Every passenger tries to buy a ticket
for(int i=0; i<5; i++){
passengerList[i]->buyTicket(newAgency.getTicketPrice());
}
cout << endl << "*** Moon mission creation ***" << endl;
// Create moon mission
Mission moonMission = Mission("MN-01",5000,20);
moonMission += astronautList[0];
moonMission += astronautList[1];
moonMission += astronautList[2];
moonMission += passengerList[0];
moonMission += passengerList[1];
moonMission += passengerList[2];
newAgency.addMission(moonMission);
cout << endl << "*** Venus mission creation ***" << endl;
// Create venus mission
Mission venusMission = Mission("VENUS-01",35000,80);
cout << "Name of the mission: " << venusMission.getName() << endl;
venusMission.setName("VS-01");
venusMission += astronautList[3];
venusMission += astronautList[4];
venusMission += passengerList[3];
venusMission += passengerList[4];
newAgency.addMission(venusMission);
cout << endl << "*** Mission creation finished ***" << endl;
// Show information of the agency
cout << newAgency;
cout << endl << "*** Lift off ***" << endl;
// Execute next mission
newAgency.executeNextMission();
cout << newAgency;
cout << endl << "*** Lift off ***" << endl;
// Execute another mission
newAgency.executeNextMission();
cout << newAgency << endl;
// Delete the allocated space
for(int i=0; i<5; i++){
delete astronautList[i];
delete passengerList[i];
}
return 0;
}
output.txt :
*** Agency creation ***
Ticket price can't be negative. It is set to 0.
Agency name: NASA, Total cash: 20000, Ticket Price: 0
Next Missions:
No missions available.
Completed Missions:
No missions completed before.
Agency name: NASA, Total cash: 20000, Ticket Price: 10000
Next Missions:
No missions available.
Completed Missions:
No missions completed before.
No available mission to execute!
*** Astronaut creation ***
Number of missions that astronaut completed can't be negative. It is set to 0.
*** Passenger creation ***
*** Moon mission creation ***
*** Venus mission creation ***
Given name does not satisfy the mission naming convention. Please set a new name!
Name of the mission: AA-00
Passenger Alex Baldwin does not have a valid ticket!
*** Mission creation finished ***
Agency name: NASA, Total cash: 20000, Ticket Price: 10000
Next Missions:
Mission number: 1 Mission name: MN-01 Cost: 5000
Mission number: 2 Mission name: VS-01 Cost: 35000
Completed Missions:
No missions completed before.
*** Lift off ***
MISSION MN-01 SUCCESSFUL!
Astronaut Neil Armstrong successfully completed 4 missions.
Astronaut Buzz Aldrin successfully completed 1 missions.
Astronaut Sally Ride successfully completed 1 missions.
Agency name: NASA, Total cash: 45000, Ticket Price: 10000
Next Missions:
Mission number: 2 Mission name: VS-01 Cost: 35000
Completed Missions:
Mission number: 1 Mission name: MN-01 Cost: 5000
*** Lift off ***
MISSION VS-01 FAILED!
Agency reschedules the mission.
Agency name: NASA, Total cash: 10000, Ticket Price: 10000
Next Missions:
Mission number: 2 Mission name: VS-01 Cost: 35000
Completed Missions:
Mission number: 1 Mission name: MN-01 Cost: 5000



Step by step
Solved in 2 steps

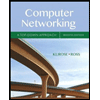
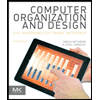
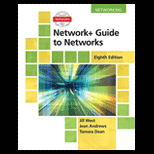
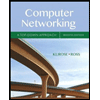
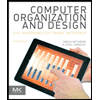
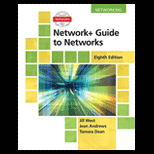
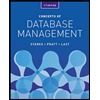
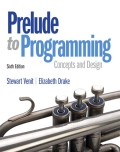
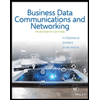