Write a class to represent Roman numerals. a. The class should have two constructors.: (1) A default constructor (2) A constructor that takes a string as a paramter (i.e., string representing a roman numeral) b. This class should have the following member functions, in addition to the above constructors: (1) set - sets the member variable to the values in the function parameter (2) print - print the converted integer value (3) convert the roman number string to a positive integer (4) print the roman number string c. This class should have two PRIVATE member variables: (1) string to hold the roman numeral string (2) integer to hold the converted value 3. Create: a. a class definition file (*.h) b. a class implementation file (*.cpp) c. a client program to test your class (*.cpp) d. a project for these code files 4. The client program should: a. create a roman numeral object using the default constructor b. convert the roman number to the integer equivalent c. output (cout) the roman number entered and the equivalent integer value d. create a roman numeral object using the overloaded constructor e. convert the roman number to the integer equivalent f. output (cout) the roman number entered and the equivalent integer value g. create an array of the roman class of size 3 h. read, from the console, three roman numerals into the array, in a loop i. convert each array component into the equivalent integer value, in a separate loop j. output (cout) the roman number entered and the equivalent integer value for each component of the array. May be in the conversion loop. For this program, enter only valid roman numerals that convert to integers less than or equal to 3999 Test with the values MCXIV, CCCLIX, MDCLXVI Continuing to get a compiling error for this code: "fatal error: Roman.h: no such file or directory" // Chapter 10 Lab - Roman Numerals // Meredith Petersen // February 23 2020 // CSC 210 Spring 2020 using namespace std; int main() { class Roman{ // Returning value of Roman numeral public: int value(char r) { if (r == 'I') return 1; if (r == 'V') return 5; if (r == 'X') return 10; if (r == 'L') return 50; if (r == 'C') return 100; if (r == 'D') return 500; if (r == 'M') return 1000; return -1; } }; #include "Roman.h" using namespace std; class RomanImplementation{ private: string str; int convertedValue; void setConvertedValue(int convertedValue){ this->convertedValue=convertedValue; } public: RomanImplementation(){ } RomanImplementation(string str){ setStr(str); } void setStr(string str){ this->str=str; } void printValue(){ cout<<"Roman to integer is "<= s2) { // If the value of the current symbol is greater than or equal to the next symbol res = res + s1; } else { res = res + s2 - s1; i++; // If the value of the current symbol is less than the next symbol } } else { res = res + s1; i++; } } setConvertedValue(res); } }; roman_test.cpp #include "roman_implementation.cpp" // Main int main() { // Considering inputs given are valid cout<<"Create a roman numeral object using the default constructor\n"; RomanImplementation romanImplementation1; romanImplementation1.setStr("MCMLIII"); romanImplementation1.romanToInteger() ; romanImplementation1.printValue(); romanImplementation1.printRomanString(); cout<<"Create a roman numeral object using the overloaded constructor\n"; RomanImplementation romanImplementation2("MMMCCCIII"); romanImplementation2.romanToInteger() ; romanImplementation2.printValue(); romanImplementation2.printRomanString(); cout<<"Create an array of the roman class of size 3\nInput strings\n"; RomanImplementation romanImplementation3[3]; string str[3]; for(int i=0;i<3;i++){ cin>>str[i]; } for(int i=0;i<3;i++){ romanImplementation3[i].setStr(str[i]); romanImplementation3[i].romanToInteger() ; romanImplementation3[i].printValue(); romanImplementation3[i].printRomanString(); } return 0; }
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
PLEASE HELP (AGAIN): Perhaps I am simply in the dark about how to use .h files. I have no file to add. These are the instructions:
Continuing to get a compiling error for this code:
"fatal error: Roman.h: no such file or directory"
// Chapter 10 Lab - Roman Numerals using namespace std; int main()
return -1; #include "Roman.h" using namespace std; private: void setConvertedValue(int convertedValue){ void romanToInteger() for (int i=0; i<str.length(); i++) // Obtaining value of symbol s[i] if (i+1 < str.length()) { // Comparing both values roman_test.cpp #include "roman_implementation.cpp" // Main cout<<"Create a roman numeral object using the default constructor\n"; RomanImplementation romanImplementation1; cout<<"Create a roman numeral object using the overloaded constructor\n"; RomanImplementation romanImplementation2("MMMCCCIII"); cout<<"Create an array of the roman class of size 3\nInput strings\n"; RomanImplementation romanImplementation3[3]; romanImplementation3[i].setStr(str[i]); |

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

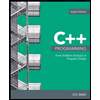
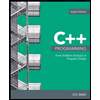