Please provide proper comments. Define the following code. #include 2 #include 3 4 #define STRING_LEN 20 5 #define SONG_BANK 5 6 #define SONGS 3 7 8 struct Song 9 { 10 char title[STRING_LEN + 1]; 11 int seconds; 12 }; 13 14 void calculateTimeFormat(int tSeconds, int* minutes, int* seconds); 15 void createPlaylist(struct Song playlist[], const struct Song bank[], int offset, int seed, int order[]); 16 void playSongs(const struct Song songs[], const int order[], int crossFade); 17 18 int main(void) 19 { 20 // 21 struct Song songBank[SONG_BANK] = { {"Song1", 546}, 22 {"Song2", 295}, 23 {"Song3", 399}, 24 {"Song4", 234}, 25 {"Song5", 111} }, 26 playlist[SONGS] = { {0} }; 27 28 // 29 // 30 // 31 int shuffled[SONGS] = { 0 }, config1 = 1, config2 = 26, config3 = 9; 32 33 createPlaylist(playlist, songBank, config1, config2, shuffled); 34 playSongs(playlist, shuffled, config3); 35 36 return 0; 37 } 38 39 void calculateTimeFormat(const int tSeconds, int* minutes, int* seconds) 40 { 41 *minutes = tSeconds / 60; 42 *seconds = tSeconds % 60; 43 } 44 45 void createPlaylist(struct Song playlist[], const struct Song bank[], int offset, int seed, int order[]) 46 { 47 int i = 0, idx = seed % SONGS; 48 49 do 50 { 51 order[i] = idx + 2 < SONGS ? idx + 2 : idx + 2 - SONGS; 52 53 if (offset < 1 || offset > SONG_BANK) 54 { 55 offset = SONG_BANK; 56 } 57 58 playlist[i++] = bank[--offset]; 59 idx = idx + 1 < SONGS ? idx + 1 : idx + 1 - SONGS; 60 61 } while (i < SONGS); 62 } 63 64 void playSongs(const struct Song songs[], const int order[], int crossFade) 65 { 66 int i, min, sec, total = 0; 67 68 for (i = 0; i < SONGS; i++) 69 { 70 total += songs[order[i]].seconds; 71 72 if (crossFade && (i + 1 < SONGS)) 73 { 74 total -= crossFade; 75 calculateTimeFormat(songs[order[i]].seconds - crossFade, &min, &sec); 76 } 77 else 78 { 79 calculateTimeFormat(songs[order[i]].seconds, &min, &sec); 80 } 81 printf("%-20s %2d:%02d\n", songs[order[i]].title, min, sec); 82 } 83 84 calculateTimeFormat(total, &min, &sec); 85 printf("Total playing time: %3d:%02d\n", min, sec); 86 } 87
Please provide proper comments.
Define the following code.
#include <stdio.h>
2 #include <string.h>
3
4 #define STRING_LEN 20
5 #define SONG_BANK 5
6 #define SONGS 3
7
8 struct Song
9 {
10 char title[STRING_LEN + 1];
11 int seconds;
12 };
13
14 void calculateTimeFormat(int tSeconds, int* minutes, int* seconds);
15 void createPlaylist(struct Song playlist[], const struct Song bank[], int offset, int seed, int order[]);
16 void playSongs(const struct Song songs[], const int order[], int crossFade);
17
18 int main(void)
19 {
20 //
21 struct Song songBank[SONG_BANK] = { {"Song1", 546},
22 {"Song2", 295},
23 {"Song3", 399},
24 {"Song4", 234},
25 {"Song5", 111} },
26 playlist[SONGS] = { {0} };
27
28 //
29 //
30 //
31 int shuffled[SONGS] = { 0 }, config1 = 1, config2 = 26, config3 = 9;
32
33 createPlaylist(playlist, songBank, config1, config2, shuffled);
34 playSongs(playlist, shuffled, config3);
35
36 return 0;
37 }
38
39 void calculateTimeFormat(const int tSeconds, int* minutes, int* seconds)
40 {
41 *minutes = tSeconds / 60;
42 *seconds = tSeconds % 60;
43 }
44
45 void createPlaylist(struct Song playlist[], const struct Song bank[], int offset, int seed, int order[])
46 {
47 int i = 0, idx = seed % SONGS;
48
49 do
50 {
51 order[i] = idx + 2 < SONGS ? idx + 2 : idx + 2 - SONGS;
52
53 if (offset < 1 || offset > SONG_BANK)
54 {
55 offset = SONG_BANK;
56 }
57
58 playlist[i++] = bank[--offset];
59 idx = idx + 1 < SONGS ? idx + 1 : idx + 1 - SONGS;
60
61 } while (i < SONGS);
62 }
63
64 void playSongs(const struct Song songs[], const int order[], int crossFade)
65 {
66 int i, min, sec, total = 0;
67
68 for (i = 0; i < SONGS; i++)
69 {
70 total += songs[order[i]].seconds;
71
72 if (crossFade && (i + 1 < SONGS))
73 {
74 total -= crossFade;
75 calculateTimeFormat(songs[order[i]].seconds - crossFade, &min, &sec);
76 }
77 else
78 {
79 calculateTimeFormat(songs[order[i]].seconds, &min, &sec);
80 }
81 printf("%-20s %2d:%02d\n", songs[order[i]].title, min, sec);
82 }
83
84 calculateTimeFormat(total, &min, &sec);
85 printf("Total playing time: %3d:%02d\n", min, sec);
86 }
87

Step by step
Solved in 2 steps with 3 images

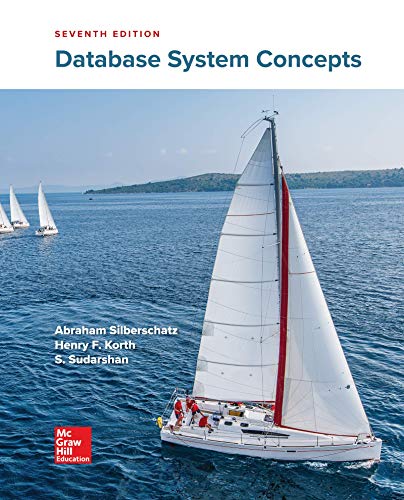
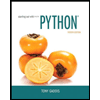
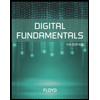
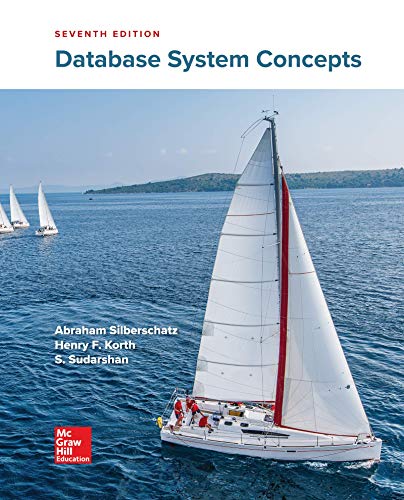
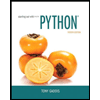
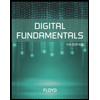
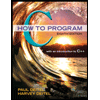
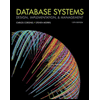
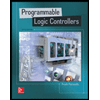