#include #include #include using namespace std; void display(char ans); void display(int n); double calcPrice(double hour, int n) { double total; cout << "Total for " << hour << " hour(s): RM"; hour=hour/3; switch (n) { case 1: total=hour*6; cout << setprecision(4) << "" << total << endl; break; case 2: total=hour*5; cout << setprecision(4) << "" << total << endl; break; case 3: total=hour*7; cout << setprecision(4) << "" << total << endl; break; } return total; } struct Car { string car1; string car2; string car3; }; int main() { string name, ic, telNo; char ans, choice; int age, n; double hour; cout << "*** WELCOME TO CAR RENTAL SYSTEM ***" << endl << endl; Car types; types.car1 = "Sedan: Honda City, Honda Civic, Perodua Bezza"; types.car2 = "Hatchback: Perodua Axia, Proton Iriz, Perodua Myvi"; types.car3 = "SUV: Honda CRV, Honda BRV, Nissan X-Trail"; cout << "~ Types of Car ~" << endl << ' ' << types.car1 << ' ' << endl << ' ' << types.car2 << ' ' << endl << ' ' << types.car3 << endl << endl; cout << "Condition: If the return of the rented vehicle exceeds the rental period," << endl; cout << "a penalty of RM5.00 will be imposed for every half an hour late." << endl << endl; cout << "Please noted that car brands cannot be selected." << endl; cout << "It will be given randomly." << endl << endl; cout << "Enter name: "; getline(cin,name); cout << endl; cout << "Hello, " << name << " :)" << endl << endl; cout << "Enter IC number: "; getline(cin,ic); cout << "Enter phone number: "; getline(cin,telNo); cout << "Enter age: "; cin >> age; if (age >= 17) { cout<<"You may rent a car."<>ans; if (ans == 'y') { do { cout << endl; cout<<"Enter rental hours: "; cin>>hour; cout<> n; display(n); calcPrice(hour,n); cout << endl; cout << "Do you want to rent a car again? " << endl; cout << "If yes (y) but if not (n): "; cin >> choice; } while (choice == 'y'); } else { cout << "You can't rent a car" << endl; } } else { cout << endl; cout << "You are underage" << endl; cout << "You can't rent a car" << endl; } cout << endl; cout << "Thank you for using our service" << endl; cout << "Have a great day!!" << endl; return 0; } void display(char ans) { cout << "Do you have a license?" << endl; cout << "If yes (y) but if not (n): " << ans; } void display(int n) { switch(n) { case 1: cout << "For every 3 hours is RM6" << endl; break; case 2: cout << "For every 3 hours is RM5" << endl; break; case 3: cout << "For every 3 hours is RM7" << endl; break; } } Question: How to implement 2D array in the above code?
#include <iostream>
#include <string>
#include <iomanip>
using namespace std;
void display(char ans);
void display(int n);
double calcPrice(double hour, int n)
{
double total;
cout << "Total for " << hour << " hour(s): RM";
hour=hour/3;
switch (n)
{
case 1: total=hour*6;
cout << setprecision(4) << "" << total << endl;
break;
case 2: total=hour*5;
cout << setprecision(4) << "" << total << endl;
break;
case 3: total=hour*7;
cout << setprecision(4) << "" << total << endl;
break;
}
return total;
}
struct Car
{
string car1;
string car2;
string car3;
};
int main()
{
string name, ic, telNo;
char ans, choice;
int age, n;
double hour;
cout << "*** WELCOME TO CAR RENTAL SYSTEM ***" << endl << endl;
Car types;
types.car1 = "Sedan: Honda City, Honda Civic, Perodua Bezza";
types.car2 = "Hatchback: Perodua Axia, Proton Iriz, Perodua Myvi";
types.car3 = "SUV: Honda CRV, Honda BRV, Nissan X-Trail";
cout << "~ Types of Car ~"
<< endl << ' '
<< types.car1 << ' '
<< endl << ' '
<< types.car2 << ' '
<< endl << ' '
<< types.car3 << endl << endl;
cout << "Condition: If the return of the rented vehicle exceeds the rental period," << endl;
cout << "a penalty of RM5.00 will be imposed for every half an hour late." << endl << endl;
cout << "Please noted that car brands cannot be selected." << endl;
cout << "It will be given randomly." << endl << endl;
cout << "Enter name: ";
getline(cin,name);
cout << endl;
cout << "Hello, " << name << " :)" << endl << endl;
cout << "Enter IC number: ";
getline(cin,ic);
cout << "Enter phone number: ";
getline(cin,telNo);
cout << "Enter age: ";
cin >> age;
if (age >= 17)
{
cout<<"You may rent a car."<<endl<<endl;
display(ans);
cin>>ans;
if (ans == 'y')
{
do
{
cout << endl;
cout<<"Enter rental hours: ";
cin>>hour;
cout<<endl;
string carType[3]={"Sedan","Hatchback","SUV"};
cout <<"Choose a type of car: "<<endl;
cout << carType[0] << " " << carType[1] << " " << carType[2];
cout << endl;
cout << "Enter 1 (Sedan), 2 (Hatchback), 3 (SUV): ";
cin >> n;
display(n);
calcPrice(hour,n);
cout << endl;
cout << "Do you want to rent a car again? " << endl;
cout << "If yes (y) but if not (n): ";
cin >> choice;
} while (choice == 'y');
}
else
{
cout << "You can't rent a car" << endl;
}
}
else
{
cout << endl;
cout << "You are underage" << endl;
cout << "You can't rent a car" << endl;
}
cout << endl;
cout << "Thank you for using our service" << endl;
cout << "Have a great day!!" << endl;
return 0;
}
void display(char ans)
{
cout << "Do you have a license?" << endl;
cout << "If yes (y) but if not (n): " << ans;
}
void display(int n)
{
switch(n)
{
case 1: cout << "For every 3 hours is RM6" << endl;
break;
case 2: cout << "For every 3 hours is RM5" << endl;
break;
case 3: cout << "For every 3 hours is RM7" << endl;
break;
}
}
Question: How to implement 2D array in the above code?

Step by step
Solved in 2 steps

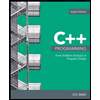
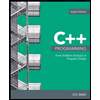