// EmployeeBonus2.cpp - This program calculates an employee's yearly bonus. #include #include using namespace std; int main() { // Declare and initialize variables. string employeeFirstName; string employeeLastName; double employeeSalary; int employeeRating; double employeeBonus; const double BONUS_1 = .25; const double BONUS_2 = .15; const double BONUS_3 = .10; const double NO_BONUS = 0.00; const int RATING_1 = 1; const int RATING_2 = 2; const int RATING_3 = 3; // This is the work done in the housekeeping() function // Get user input cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter employee's yearly salary: "; cin >> employeeSalary; cout << "Enter employee's performance rating: "; cin >> employeeRating; // This is the work done in the detailLoop() function // Use switch statement to calculate bonus based on rating // This is the work done in the endOfJob() function // Output cout << "Employee Name: " << employeeFirstName << " " << employeeLastName << endl; cout << "Employee Salary: $" << employeeSalary << endl; cout << "Employee Rating: " << employeeRating << endl; cout << "Employee Bonus: $" << employeeBonus << endl; return 0; }
// EmployeeBonus2.cpp - This program calculates an employee's yearly bonus. #include #include using namespace std; int main() { // Declare and initialize variables. string employeeFirstName; string employeeLastName; double employeeSalary; int employeeRating; double employeeBonus; const double BONUS_1 = .25; const double BONUS_2 = .15; const double BONUS_3 = .10; const double NO_BONUS = 0.00; const int RATING_1 = 1; const int RATING_2 = 2; const int RATING_3 = 3; // This is the work done in the housekeeping() function // Get user input cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter employee's yearly salary: "; cin >> employeeSalary; cout << "Enter employee's performance rating: "; cin >> employeeRating; // This is the work done in the detailLoop() function // Use switch statement to calculate bonus based on rating // This is the work done in the endOfJob() function // Output cout << "Employee Name: " << employeeFirstName << " " << employeeLastName << endl; cout << "Employee Salary: $" << employeeSalary << endl; cout << "Employee Rating: " << employeeRating << endl; cout << "Employee Bonus: $" << employeeBonus << endl; return 0; }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 3PE
Related questions
Question
// EmployeeBonus2.cpp - This program calculates an employee's yearly bonus.
#include <iostream>
#include <string>
using namespace std;
int main()
{
// Declare and initialize variables.
string employeeFirstName;
string employeeLastName;
double employeeSalary;
int employeeRating;
double employeeBonus;
const double BONUS_1 = .25;
const double BONUS_2 = .15;
const double BONUS_3 = .10;
const double NO_BONUS = 0.00;
const int RATING_1 = 1;
const int RATING_2 = 2;
const int RATING_3 = 3;
// This is the work done in the housekeeping() function
// Get user input
cout << "Enter employee's first name: ";
cin >> employeeFirstName;
cout << "Enter employee's last name: ";
cin >> employeeLastName;
cout << "Enter employee's yearly salary: ";
cin >> employeeSalary;
cout << "Enter employee's performance rating: ";
cin >> employeeRating;
// This is the work done in the detailLoop() function
// Use switch statement to calculate bonus based on rating
// This is the work done in the endOfJob() function
// Output
cout << "Employee Name: " << employeeFirstName << " " << employeeLastName << endl;
cout << "Employee Salary: $" << employeeSalary << endl;
cout << "Employee Rating: " << employeeRating << endl;
cout << "Employee Bonus: $" << employeeBonus << endl;
return 0;
}
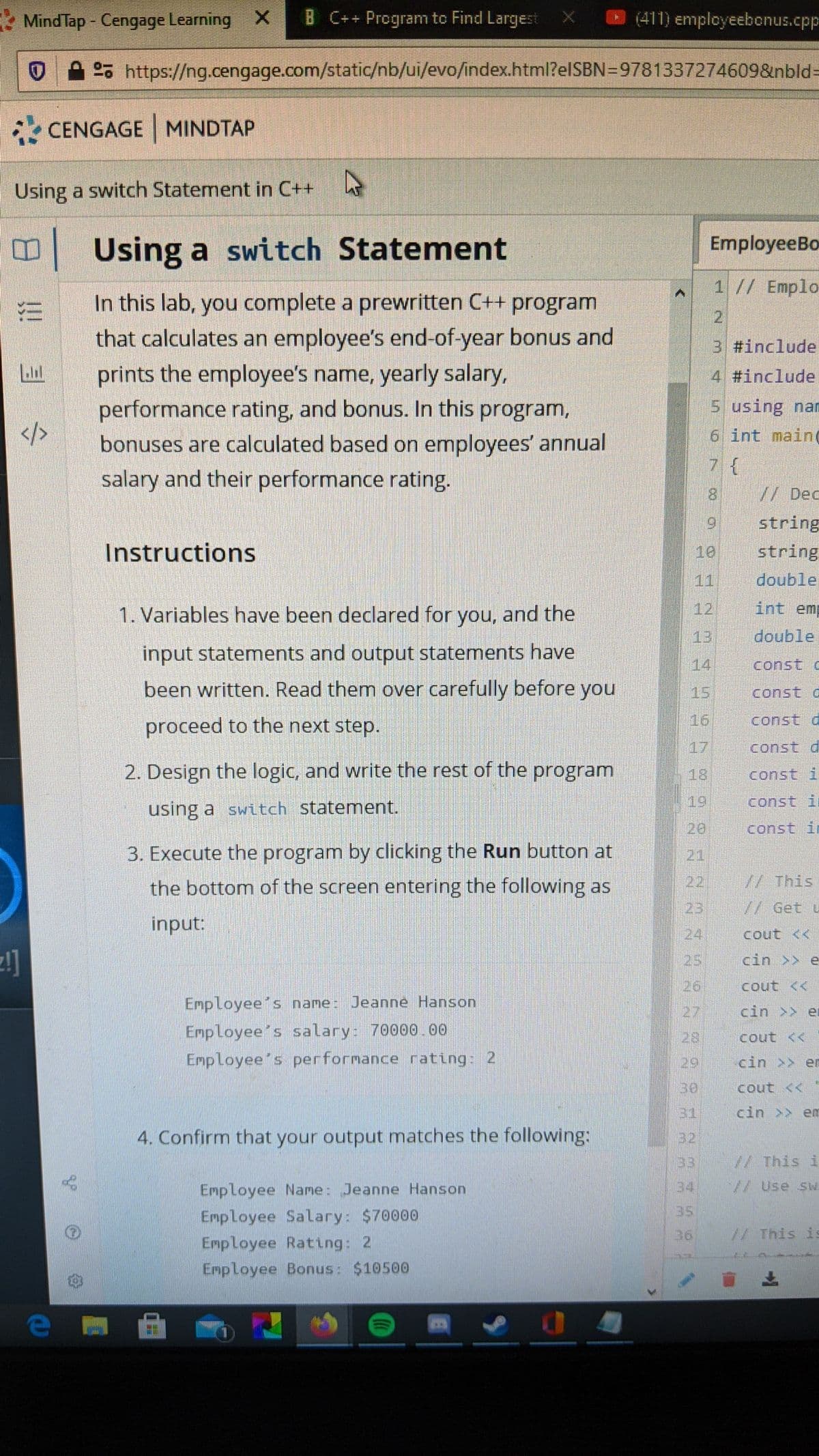
Transcribed Image Text:* Mind Tap - Cengage Learning X
B C++ Program to Find Largest
O (411) employeebonus.cpp
25 https://ng.cengage.com/static/nb/ui/evo/index.html?elSBN=9781337274609&nbld-
• CENGAGE MINDTAP
Using a switch Statement in C++
B Using a switch Statement
EmployeeBo
1 // Emplo
In this lab, you complete a prewritten C++ program
that calculates an employee's end-of-year bonus and
prints the employee's name, yearly salary,
performance rating, and bonus. In this program,
bonuses are calculated based on employees' annual
3 #include
4 #include
5 using nam
6 int main
</>
salary and their performance rating.
8.
//Dec
9,
string
Instructions
10
string
11
double
1. Variables have been declared for you, and the
12
int emp
13
double
input statements and output statements have
14
const c
been written. Read them over carefully before you
15
const o
proceed to the next step.
16
const d
17
const d
2. Design the logic, and write the rest of the program
18
const i
(19
const in
using a switch statement.
20
const ir
3. Execute the program by clicking the Run button at
21
the bottom of the screen entering the following as
22 // This
23
7/ Get u
input:
24
cout <<
25
cin >> e
26
cout <<
Employee's name: Jeanne Hanson
27
cin >> er
Employee's salary: 70000.00
Employee's performance rating: 2
28
cout <<
29
cin >> en
30
cout <<
31
cin >> em
4. Confirm that your output matches the following:
32
33
//This i
Employee Name: Jeanne Hanson
34
// Use sw
35
Employee Salary: $70000
Employee Rating: 2
36
//This is
Employee Bonus: $10500
!!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
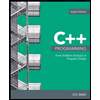
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
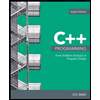
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning