Please read my question and help me answer! Thanks! public class CustomString { String myString; boolean isSet; public CustomString() { // TODO Implement constructor this.myString = null; this.isSet = false;
Please read my question and help me answer! Thanks!
public class CustomString {
String myString;
boolean isSet;
public CustomString() {
// TODO Implement constructor
this.myString = null;
this.isSet = false;
}
/**
* Returns a new string version of the current string where the capitalization is reversed (i.e., lowercase to uppercase,
* and uppercase to lowercase) for the alphabetical characters specified in the given arg.
*
* All non-alphabetical characters are unaffected.
* If the current string is null, empty, or has not been set to a value, this method should return an empty string.
*
* Example(s):
* - For a current string "abc, XYZ; 123.", calling reverse("bcdxyz@3210.") would return "aBC, xyz; 123."
* - For a current string "abc, XYZ; 123.", calling reverse("6,.") would return "abc, XYZ; 123."
* - For a current string "abc, XYZ; 123.", calling reverse("") would return "abc, XYZ; 123."
* - For a current string "", calling reverse("") would return ""
*
* Remember: This method builds and returns a new string, and does not directly modify the myString field.
*
* @param arg the string containing the alphabetical characters to have their capitalization reversed in the current string
* @return new string in which the alphabetical characters specified in the arg are reversed
*/
public String reverse(String arg) {
//Write your code here
}
/**
* Returns a new string version of the current string where all the letters either >= or <= the given char n, are removed.
*
* The given letter may be a-z or A-Z, inclusive.
* The letters to be removed are case insensitive.
*
* If 'more' is false, all letters less than or equal to n will be removed in the returned string.
* If 'more' is true, all letters greater than or equal to n will be removed in the returned string.
*
* If the current string is null, the method should return an empty string.
* If n is not a letter (and the current string is not null), the method should return an empty string.
*
* Example(s):
* - For a current string "Hello 90, bye 2", calling filterLetters('h', false) would return "llo 90, y 2"
* - For a current string "Abcdefg", calling filterLetters('c', false) would return "defg"
* - For a current string "Hello 90, bye 2", calling filterLetters('h', true) would return "e 90, be 2"
* - For a current string "Abcdefg", calling filterLetters('c', true) would return "Ab"
*
* Remember: This method builds and returns a new string, and does not directly modify the myString field.
*
* @param n char to compare to
* @param more indicates whether letters <= or >= n will be removed
* @return new string with letters removed
*/
public String filterLetters(char n, boolean more) {
//Write your code here
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

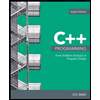
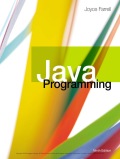
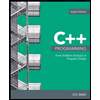
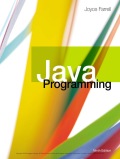