Modify the method beginsWithVowel. Use a different way to implement the method (try method indexOf() from the String class). 2. Modify the driver class to iteratively ask the user to enter a sentence, and then translate the sentence, until the user decides to quit the program. import java.util.Scanner; public class Translator { //instance variables //constructors //methods public static String translate(String sentence)// My name is Patrick Casseus { String result = ""; sentence = sentence.toLowerCase(); Scanner scan = new Scanner(sentence); while(scan.hasNext()) { result = result += translateWord(scan.next()) + " "; } return result; } private static String translateWord(String word) { String result = ""; if(startWithVowel(word)) result = word + "yay"; else if(startWithBlend(word)) // chair -> air + ch + ay result = word.substring(2) + word.substring(0,2) + "ay"; else result = word.substring(1) + word.substring(0,1) + "ay"; //can also do word.charAt(0) ) return result; } private static boolean startWithVowel(String word) { char letter = word.charAt(0); if(letter == 'a' || letter == 'e' || letter == 'i' || letter == 'o' || letter == 'u') return true; else return false; } private static boolean startWithBlend(String word) { String firstTwo = word.substring(0,2); if(firstTwo.equals("bl")||firstTwo.equals("br")||firstTwo.equals("ch")||firstTwo.equals("cl")||firstTwo.equals("cr")||firstTwo.equals("ch")||firstTwo.equals("sh")) return true; else return false; } } Driver Class import java.util.*; public class PigLatin { public static void main(String [] args) { Scanner scan = new Scanner(System.in); System.out.println("Enter a sentence: "); String sentence = scan.nextLine(); String result = Translator.translate(sentence); System.out.println("Translated sentence: " + result); } }
1. Modify the method beginsWithVowel. Use a different way to implement the method (try method indexOf() from the String class).
2. Modify the driver class to iteratively ask the user to enter a sentence, and then translate the sentence, until the user decides to quit the program.
import java.util.Scanner;
public class Translator {
//instance variables
//constructors
//methods
public static String translate(String sentence)// My name is Patrick Casseus
{
String result = "";
sentence = sentence.toLowerCase();
Scanner scan = new Scanner(sentence);
while(scan.hasNext())
{
result = result += translateWord(scan.next()) + " ";
}
return result;
}
private static String translateWord(String word)
{
String result = "";
if(startWithVowel(word))
result = word + "yay";
else if(startWithBlend(word)) // chair -> air + ch + ay
result = word.substring(2) + word.substring(0,2) + "ay";
else
result = word.substring(1) + word.substring(0,1) + "ay"; //can also do word.charAt(0) )
return result;
}
private static boolean startWithVowel(String word)
{
char letter = word.charAt(0);
if(letter == 'a' || letter == 'e' || letter == 'i' || letter == 'o' || letter == 'u')
return true;
else
return false;
}
private static boolean startWithBlend(String word)
{
String firstTwo = word.substring(0,2);
if(firstTwo.equals("bl")||firstTwo.equals("br")||firstTwo.equals("ch")||firstTwo.equals("cl")||firstTwo.equals("cr")||firstTwo.equals("ch")||firstTwo.equals("sh"))
return true;
else
return false;
}
}
Driver Class
import java.util.*;
public class PigLatin {
public static void main(String [] args) {
Scanner scan = new Scanner(System.in);
System.out.println("Enter a sentence: ");
String sentence = scan.nextLine();
String result = Translator.translate(sentence);
System.out.println("Translated sentence: " + result);
}
}

Step by step
Solved in 3 steps with 1 images

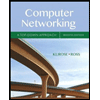
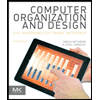
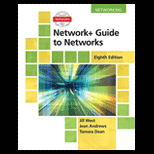
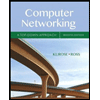
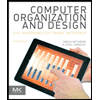
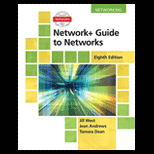
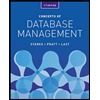
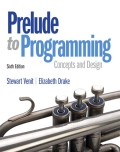
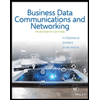