Please use the sample input to see if the code works, as the output of the final code, must be the same as the correct output. Follow the details and notes provided and show the complete working sample code. Additionally, include comments to make the code easier to understand. I will show how the output was found (check K); Note: The final code should result in correct output (check I) if sample input A (check H) is used, or else I will give an automatic thumbs down A. TASK The user inputs a path system and the work needed to traverse them. The goal is to Identify the relationships between paths, whether they have sequential, adjacent, or null relationships. For every sequential and adjacent relationship, give their work done based on the formula (check D). B. SAMPLE A path system illustration //See Image C. Relationship Examples with Sample A as basis Sequential relationships: Path1 and Path2 as they are single paths that are continuously connected Adjacent relationships: Path3 and Path4; Path4 and Path5 as they are multiple paths with the same stage in and stage out Null relationships occur when the paths are neither sequential nor adjacent. Examples: Path2 and Path3; Path2 and Path6, etc. D. Formula The goal is to transform those with relationships into a single path via the formula. For paths having sequential relationships, you sum them. Ex. Path1Work + Path2Work For paths having adjacent relationships, you use this formula: (Path(x)work * Path(y)work * 2)/(Path(x)work+Path(y)work); Ex. (Path3Work * Path4Work * 2) / (Path3Work + Path4Work) E. Limitations: - You can only use standard libraries, for example, Math and Sys. - You can use famous algorithms like Prim's, Kruskal's, Dijkstra's, Bellman-Ford, Floyd-Warshall, and others. - 1 ≤ Path, Relationship ≤ 1000 - 0 ≤ Work ≤ 109 F. Input Format (This should be only what is asked, check H) Note: Use Loop and input().split() to receive the data from the user 1. The first line asks for two integer inputs, P and R; P is for the number of paths, and R is for the number of relationships to check. 2. Depending on the value of P, the code will ask for three strings and one integer for the pathname, stagein, stageout, and pathwork. Note: Pathwork is the integer value used in the formulas. 3. Depending on the value of R, the code will ask for two string inputs containing pathname1 and pathname2, whose relationship will be compared. G. Output Format (This should be only what is asked, check I) 1. Depending on the number of R, each line will show the relationship per pathname1 and pathname2 combination if it is Sequential / Adjacent / Null. 2. Display all those with Sequential and Adjacent relationships and their work done after the formula is used in order. H. Sample Input A (Based on Sample A) 6 4 Path1 Start Stage2 100 Path2 Stage2 Stage3 60 Path3 Stage3 Stage4 100 Path4 Stage3 Stage4 50 Path5 Stage3 Stage4 100 Path6 Stage4 End 20 Path1 Path2 Path2 Path3 Path4 Path5 Path2 Path6 I. Correct Output of Sample Input A Sequential Null Adjacent Null [Path1, Path2] 200 [Path3, Path4] 67 [Path3, Path5] 100 [Path4, Path5] 67 J. Illustration of Sample A path system with work values based on (H) //See Image K. Explanation of how the output was reached: #1 Path1 Path2 is Sequential Path2 Path3 is Null as it is neither sequential nor adjacent Path4 Path5 is Adjacent Path2 Path6 is Null as it is neither sequential nor adjacent #2 Path1 and Path2 are sequential; 100+100=200 Path3 and Path4 are adjacent; (100 * 50 * 2) / (100 + 50)=66.666… //But the variable is an integer, so roundoff = 67 Path3 and Path5 are adjacent; (100 * 100 * 2) / (100 + 100)=100 Path4 and Path5 are adjacent; (100 * 50 * 2) / (100 + 50)=66.666… //But the variable is an integer, so roundoff = 67 Note: These are the only four that should appear as part of the #2 requirement of the Output Format with Sample A Input L. Sample Input to what I mean (You can change or edit this as long as input() is used) P, R = input().split() for a in range(P): Path, StageIn, StageOut, PathWork = input().split() Val = int(PathWork) ……. for b in range(R): pathname1, pathname2 = input().split() …….. //Please test the Final Code with Sample Input A (H). It should result in the correct output (I) for thumbs up.
[Updated[ Python Code Pt1:
Please use the sample input to see if the code works, as the output of the final code, must be the same as the correct output. Follow the details and notes provided and show the complete working sample code. Additionally, include comments to make the code easier to understand. I will show how the output was found (check K);
Note: The final code should result in correct output (check I) if sample input A (check H) is used, or else I will give an automatic thumbs down
A. TASK
The user inputs a path system and the work needed to traverse them. The goal is to Identify the relationships between paths, whether they have sequential, adjacent, or null relationships. For every sequential and adjacent relationship, give their work done based on the formula (check D).
B. SAMPLE A path system illustration
//See Image
C. Relationship Examples with Sample A as basis
Sequential relationships: Path1 and Path2 as they are single paths that are continuously connected
Adjacent relationships: Path3 and Path4; Path4 and Path5 as they are multiple paths with the same stage in and stage out
Null relationships occur when the paths are neither sequential nor adjacent. Examples: Path2 and Path3; Path2 and Path6, etc.
D. Formula
The goal is to transform those with relationships into a single path via the formula.
For paths having sequential relationships, you sum them. Ex. Path1Work + Path2Work
For paths having adjacent relationships, you use this formula: (Path(x)work * Path(y)work * 2)/(Path(x)work+Path(y)work);
Ex. (Path3Work * Path4Work * 2) / (Path3Work + Path4Work)
E. Limitations:
- You can only use standard libraries, for example, Math and Sys.
- You can use famous algorithms like Prim's, Kruskal's, Dijkstra's, Bellman-Ford, Floyd-Warshall, and others.
- 1 ≤ Path, Relationship ≤ 1000
- 0 ≤ Work ≤ 109
F. Input Format (This should be only what is asked, check H)
Note: Use Loop and input().split() to receive the data from the user
1. The first line asks for two integer inputs, P and R; P is for the number of paths, and R is for the number of relationships to check.
2. Depending on the value of P, the code will ask for three strings and one integer for the pathname, stagein, stageout, and pathwork.
Note: Pathwork is the integer value used in the formulas.
3. Depending on the value of R, the code will ask for two string inputs containing pathname1 and pathname2, whose relationship will be compared.
G. Output Format (This should be only what is asked, check I)
1. Depending on the number of R, each line will show the relationship per pathname1 and pathname2 combination if it is Sequential / Adjacent / Null.
2. Display all those with Sequential and Adjacent relationships and their work done after the formula is used in order.
H. Sample Input A (Based on Sample A)
6 4
Path1 Start Stage2 100
Path2 Stage2 Stage3 60
Path3 Stage3 Stage4 100
Path4 Stage3 Stage4 50
Path5 Stage3 Stage4 100
Path6 Stage4 End 20
Path1 Path2
Path2 Path3
Path4 Path5
Path2 Path6
I. Correct Output of Sample Input A
Sequential
Null
Adjacent
Null
[Path1, Path2] 200
[Path3, Path4] 67
[Path3, Path5] 100
[Path4, Path5] 67
J. Illustration of Sample A path system with work values based on (H)
//See Image
K. Explanation of how the output was reached:
#1
Path1 Path2 is Sequential
Path2 Path3 is Null as it is neither sequential nor adjacent
Path4 Path5 is Adjacent
Path2 Path6 is Null as it is neither sequential nor adjacent
#2
Path1 and Path2 are sequential; 100+100=200
Path3 and Path4 are adjacent; (100 * 50 * 2) / (100 + 50)=66.666… //But the variable is an integer, so roundoff = 67
Path3 and Path5 are adjacent; (100 * 100 * 2) / (100 + 100)=100
Path4 and Path5 are adjacent; (100 * 50 * 2) / (100 + 50)=66.666… //But the variable is an integer, so roundoff = 67
Note: These are the only four that should appear as part of the #2 requirement of the Output Format with Sample A Input
L. Sample Input to what I mean (You can change or edit this as long as input() is used)
P, R = input().split()
for a in range(P):
Path, StageIn, StageOut, PathWork = input().split()
Val = int(PathWork)
…….
for b in range(R):
pathname1, pathname2 = input().split()
……..
//Please test the Final Code with Sample Input A (H). It should result in the correct output (I) for thumbs up.


Step by step
Solved in 3 steps

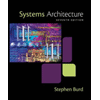
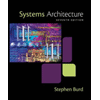