Question
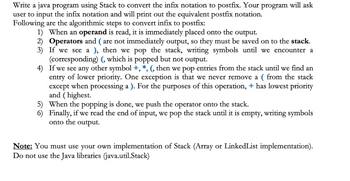
Transcribed Image Text:Write a java program using Stack to convert the infix notation to postfix. Your program will ask
user to input the infix notation and will print out the equivalent postfix notation.
Following are the algorithmic steps to convert infix to postfix:
1) When an operand is read, it is immediately placed onto the output.
2) Operators and ( are not immediately output, so they must be saved on to the stack.
3) If we see a ), then we pop the stack, writing symbols until we encounter a
(corresponding) (, which is popped but not output.
4) If we see any other symbol +, *, (, then we pop entries from the stack until we find an
entry of lower priority. One exception is that we never remove a (from the stack
except when processing a ). For the purposes of this operation, + has lowest priority
and (highest.
5) When the popping is done, we push the operator onto the stack.
6)
Finally, if we read the end of input, we pop the stack until it is empty, writing symbols
onto the
output.
Note: You must use your own implementation of Stack (Array or LinkedList implementation).
Do not use the Java libraries (java.util.Stack)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 2 images

Knowledge Booster
Similar questions
- Use stack as a data structure in creating a program that enable to reversed the inputstring.Create a java program that asks a user to input student’s full name and reverses the string.The program should be able to print how the string is reversed and display the reversed string. The program should display one by one on how to reverse the string and then display the final output of the reversed string.arrow_forwardWe need to add the first two digits and return the sum as a linked list. Please do code in c++ Output and screenshot of code is necessary. Logic diagram given below. Example 1: Input 11 = 12 = 2 5 [2,4,3] Output 7 [5,6,4] [7,0,8] 6 3 8arrow_forwardSolve the problem in the imagearrow_forward
- In evaluating a postfix expression, if the symbol just read is the variable A then the next action is: (Points: 2) A. Push A onto the stack B. Write A to output string. C. Pop the stack. D. Read another symbol from input stringarrow_forwardUsing c++ Contact list: Binary Search A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Frank 867-5309 Joe…arrow_forwardWrite a program that uses stacks to evaluate an arithmetic expression in infix notation without converting it into postfix notation. The program takes as input a numeric expression in infix notation, such as 3+4*2, and outputs the result. 1) Operators are +, -, * 2) Assume that the expression is formed correctly so that each operation has two arguments. 3) The expression can have parenthesis, for example: 3*(4-2)+6. 4) The expression can have negative numbers. 5) The expression can have spaces in it, for example: 3 * (4-2) +6. Here are some useful functions that you may need: char cin.peek(); -- retums the next character of the cin input stream ( without reading it) bool isdigit(char c); -- returns true if e is one of the digits '0' through *9', false otherwise cin.ignore(); -- reads and discards the next character from the cin input stream cin.get(char &c); -- reads a character in e ( could be a space or the new line )arrow_forward
- PYTHON PROGRAMMING ONLY PLZ( USING STACKS AND QUEUES)(DO NOT JUST PRINT THE RAW LIST MUST BE IN A STACK) my code and an example of a stack will be below just need help a little confused. Write a small program that simulates a doctor's Office. Your office will perform the following tasks Keep track of the waiting list using a queue Keep track of what checks need to happen to each person in a stack (check temperature, check weight, etc..). Your program will Ask the patient for their name. (you will store this data in a queue) (You must have at least 4 names in your queue before calling them to the doctor) Ask the customers what brings them in today Display to the console You will show your patients being called to the doctor and the queue changing. Describe your queue do not just print the raw list You will show the doctor administering the checks in the stack and your stack being updated. Describe your stack do not just print the raw stack How you create the doctor's…arrow_forwardWrite a Java program and screenshot of the output using Stack class to show the following points: a. Create three empty stacks named stackOne, stackTwo and stackThree respectively. b. Push the numbers [a, b, c, d] "for example [7, 5, 8, 22]" into stackOne and [w, x, y, z] "for example [5, 4, 2, 11]” into stackTwo. (Note: value of the numbers [a,b,c,d, etc] for each of the student should be different) c. Pop the top number of stackOne and top number of stackTwo and add them. Then, push the addition result into stack Three. d. Pop the top number of stackOne and top number of stackTwo and subtract them. Push the result of subtraction into stackThree. e. Print StackOne, stackTwo and stackThree. f. Print the largest number in stack Threearrow_forward
arrow_back_ios
arrow_forward_ios