Write a complete Java program that contains Main and Car classes. You will write a Car class that consists of car model, engine power, year and tax payment values as the instance variables. In your program, you will set the values, except tax payment, of above properties in the constructor method. Then, you will calculate the tax payment value in the
Write a complete Java program that contains Main and Car classes. You will write a Car class that consists of car model, engine power, year and tax payment values as the instance variables. In your program, you will set the values, except tax payment, of above properties in the constructor method. Then, you will calculate the tax payment value in the
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 9PE
Related questions
Question
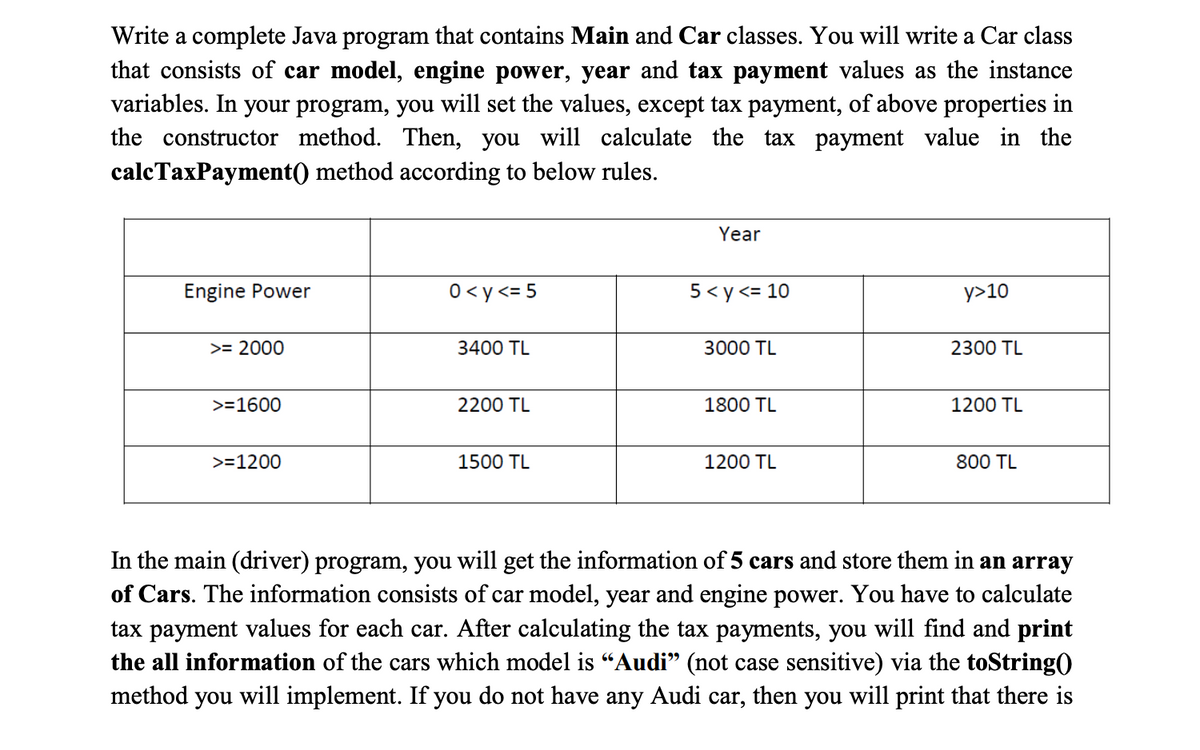
Transcribed Image Text:Write a complete Java program that contains Main and Car classes. You will write a Car class
that consists of car model, engine power, year and tax payment values as the instance
variables. In your program, you will set the values, except tax payment, of above properties in
the constructor method. Then, you will calculate the tax payment value in the
calcTaxPayment() method according to below rules.
Year
Engine Power
0<y<= 5
5< y <= 10
y>10
>= 2000
3400 TL
3000 TL
2300 TL
>=1600
2200 TL
1800 TL
1200 TL
>=1200
1500 TL
1200 TL
800 TL
In the main (driver) program, you will get the information of 5 cars and store them in an array
of Cars. The information consists of car model, year and engine power. You have to calculate
tax payment values for each car. After calculating the tax payments, you will find and print
the all information of the cars which model is “Audi" (not case sensitive) via the toString()
method you will implement. If you do not have any Audi car, then you will print that there is
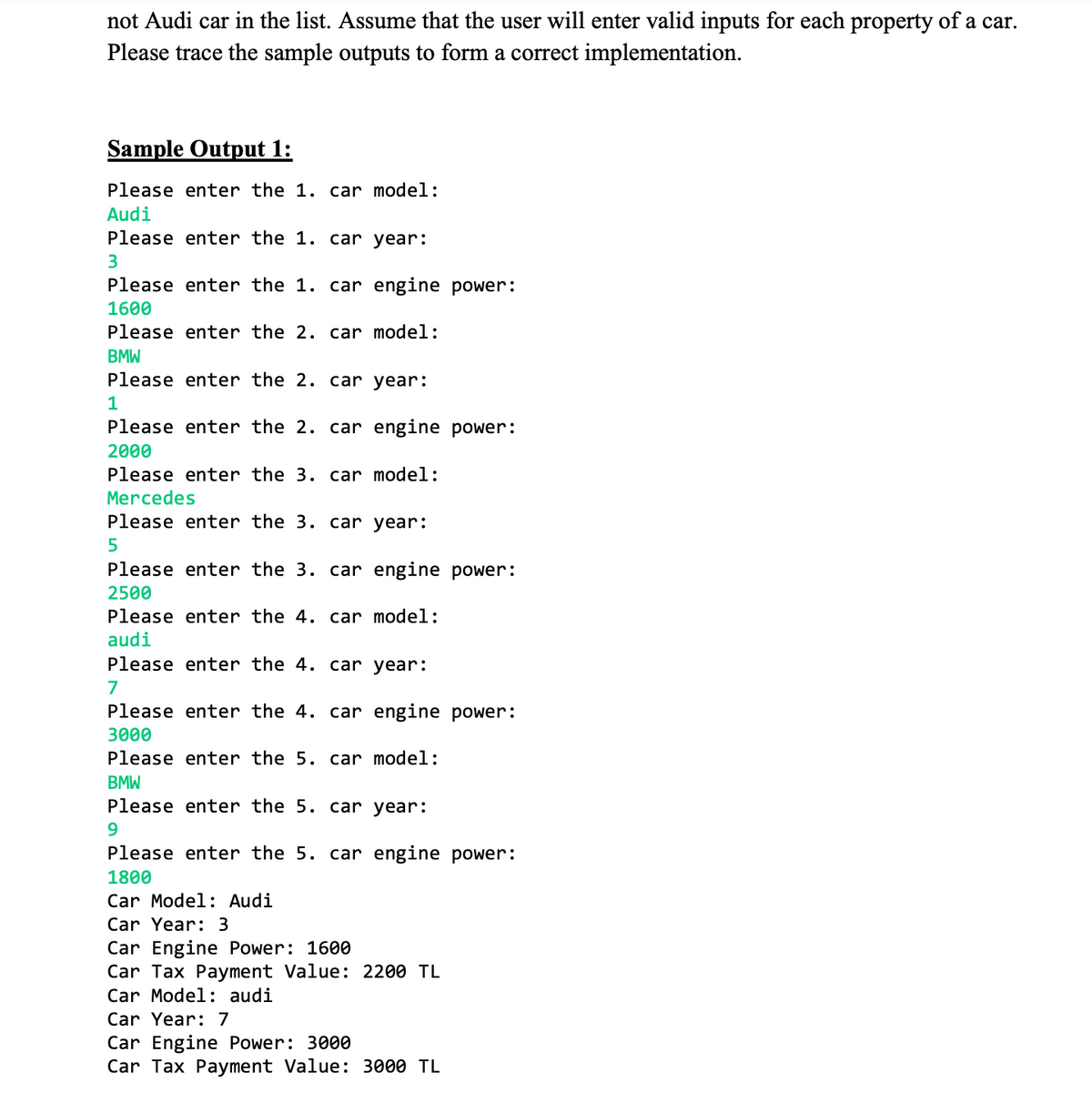
Transcribed Image Text:not Audi car in the list. Assume that the user will enter valid inputs for each property of a car.
Please trace the sample outputs to form a correct implementation.
Sample Output 1:
Please enter the 1. car model:
Audi
Please enter the 1. car year:
3
Please enter the 1. car engine power:
1600
Please enter the 2. car model:
BMW
Please enter the 2. car year:
1
Please enter the 2. car engine power:
2000
Please enter the 3. car model:
Mercedes
Please enter the 3. car year:
Please enter the 3. car engine power:
2500
Please enter the 4. car model:
audi
Please enter the 4. car year:
7
Please enter the 4. car engine power:
3000
Please enter the 5. car model:
BMW
Please enter the 5. car year:
9.
Please enter the 5. car engine power:
1800
Car Model: Audi
Car Year: 3
Car Engine Power: 1600
Car Tax Payment Value: 2200 TL
Car Model: audi
Car Year: 7
Car Engine Power: 3000
Car Tax Payment Value: 3000 TL
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
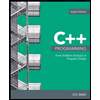
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
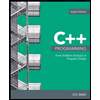
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning