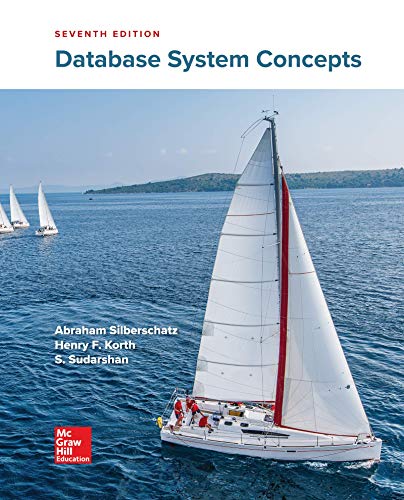
In java language
Write a Rectangle class. A Rectangle has properties of width and length. You construct a Rectangle object by providing the width and length in that order. If no width and length are provided to the constructor, construct a Rectangle with width 0.0 and length 0.0. We want to be able to get and set both the width and the length independently. We also want to be able to ask for the area of the rectangle and the perimeter of the rectangle.
What will the object need to remember? width and length - those are the instance variables.
Rectangle class has these constructors :
- public Rectangle() - Constructs a new rectangle with width and length of 0.0.
- public Rectangle(double width, double length) - Constructs a new rectangle with the given width and length. Remember that the job of the constructor is to initialize the instance variables.
It has these methods.
- public double getWidth() - Gets the width of this Rectangle
- public double getLength() - Gets the length of this Rectangle
- public void setWidth(double aWidth) - Sets a new width for this Rectangle
- public void setLength(double aLength) - Sets a new length for this Rectangle
- public double area() - Calculates and returns this Rectangle's area (product of width and length)
- public double perimeter() - Calculates and returns this Rectangle's perimeter (sum of all four sides)
- public void printDimensions() - Displays the width and length of this Rectangle in the following format (to two decimal places):
- Width: 3.48
- Height: 7.27
The methods and constructor are provided as stubs in the starter file. A stub has a method header and a body with no implementation.
- The stub for an accessor returns 0 for numbers or null for objects like strings.
- A stub for a mutator method has no body at all.
A class with stubs for methods will compile, but it does not yet behave correctly. You still need to supply implementation and the correct return values. The idea is that you can implement one method at a time and test it since the class will compile. This technique is frequently used in development of applications.
You are given a RectangleTester class in Codecheck along with the starter for the Rectangle class. Copy both into your BlueJ project. RectangleTester is an application with a main method. To run your application, right click on it and execute its main method.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- Write up Java source codes for compiling and execution of program below. There is a very simple solution to keeping Tic-tac-toe fresh and interesting, though, and it has been thought up by a group of mathematicians. It’s being called Ultimate Tic-tac-toe and simply embeds a Tic-tac-toe board in each of the nine squares of the original game. Now instead of just winning the main board, you also need to win each of the smaller boards first until you have a line of three. New Rule: You can only place a mark on the board determined by the position of your opponent’s last placed mark. So, if they put an X or O in the top right corner of a square, your next move must occur in the top right board. By adding that rule the game is no longer about winning a single board, it’s about tactically managing up to 9 games at once and plotting ahead. You still win by marking three squares in a row, but that now involves winning three games. The effects of the New Rule: 1: Your opponent can force you…arrow_forwardin Java: Create a class LukcyNumber which checks if the input is a lucky number. A lucky number is a number where the sum of squares of every even positioned digit(starting from the second position) is a multiple of 9.arrow_forwardImplement the main method below in a class called Grade. The program should read integer points (use the Scanner class) and print the grade (“A”, “B”, “C”, “D”, or “F”) based on the final score associated with a student. Grades are classified as follows: Number of Points Final Grade Greater than or equal to 90 A Between 80 (inclusive) and 90 (exclusive) B Between 70 (inclusive) and 80 (exclusive) C Between 60 (inclusive) and 70 (exclusive) D Less than 60 F Restrictions/Assumptions Use the message “Enter final score” to prompt the user to enter the number of points. Use the Scanner class to read the number of points, i.e., Scanner sc = new Scanner(System.in); Use System.out.println(...) to print the final grade. Assume that the user correctly enters an integer score. Start with this code: import java.util.Scanner; public class Grade { public static void main(String[] args) { For the toolbar, press ALT+F10 (PC) or ALT+FN+F10 (Mac)arrow_forward
- WRITE IN JAVA USING JOptionPane.showInputDialog Write a program that reads a sentence as input and converts each word to “Pig Latin”. In one version of Pig Latin, you convert a word by removing the first letter, placing that letter at the end of the word, and then appending “ay” to the word. Here is an Example: English: I SLEPT MOST OF THE NIGHT Pig Latin: IAY LEPTSAY OSTMAY FOAY HETAY IGHTNAY EXAMPLE:arrow_forwardJavaarrow_forwardJava Write a method drivingCost.arrow_forward
- Computer Science Create a UML diagram of the following methods & classes (java language) using LucidApp. Class Name Method Description Calculation Multiply Multiplies two variables with data type double and returns the product Returns the area of the Rectangle using the method "Multiply" of the "Calculation" class in the calculation Rectangle Area Rec_Prism Volume - Returns the volume of a Rectangular Prism - Inherits class "Rectangle" and utilizes the method "Area" in the calculation Triangle Returns the area of the Triangle using the method “Multiply" of the "Calculation" class in the calculation Area Tri_Prism Volume Returns the volume of a Triangular Prism - Inherits class "Triangle" and utilizes the method "Area" in the calculation Reflexive Power - Inherits class "Calculation" - Returns the product of a number multiplied by itself based on the exponent - Requires two input parameters: a = the number b= the exponent - Calls the method "Multiply" to perform the multiply operation.…arrow_forwardOutput the numbers 1-100 inclusive starting with 1. Please a comma and a space after each number except the last. Output a new line after the last number. Write a code in Java that results like test case 1arrow_forwardComputer Science Questionarrow_forward
- java please dont take other website'answer. rthis is actually practice question ANIMALCLASS Create an Animal class. Each animal has a name, an x and y integer coordinate. The Animal class should have at minimum the following methodsbelowbut you may want to add more if necessary: Also note, everyanimal will need to have to have “z”passed to it so that it knows how big the map is. •A constructor that starts the animal at 0,0 with a name of "Unknown Animal"and only accepts a single int value (int mapSize). •A parameter constructor that allows the programmerto input all 4pieces of information.(x,y, name, mapSize)oCheck the parameters for valid input based on the constraints. Ifany of the input valuesis invalid, adjust it any way you deem necessary in order to make it valid. •getX()and getY() •getName() •toString(). o This should print out the name and coordinates of the animal. •touching(Animal x) This method should determine if the animal is on the same spot as a secondanimal(x). It…arrow_forwardJAVA SWINGI have definied a straight TUBE as: int rectWidth = 25; // replace 50 with the desired width of the rectangleint rectHeight = 200; // replace 200 with the desired height of the rectangleint rectX = (this.getWidth() - rectWidth) / 2;int rectY = (this.getHeight() - rectHeight) / 2;game.fillRect(rectX, rectY, rectWidth, rectHeight);I need L tube, which connects the straight tubes. How to define L tubes?arrow_forwardWrite a java program that will create a Deck object and shuffle the deck. Then, you will draw ten cards from the deck and perform selection statements that carry out the following: If the card is red, then print “Card is red” to the screen. Otherwise, print “Card is black” to the screen. If the card is a picture card, print “That's a picture card”. If the card is an ace, print “That's an Ace”. If the card has a value less than 6, print “Card has a small value”. If the card has a value between 6 and 10 (inclusive), print “Card has a large value”. In the source code, write an algorithm for the program in a comment block. Also, in a comment block, paste the results of running your program. Print out and turn in the completed source code with those comments.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
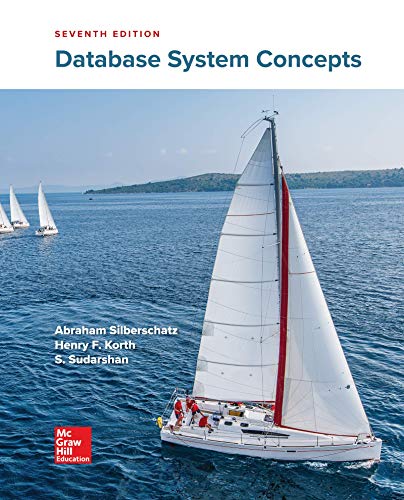
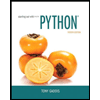
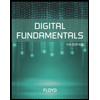
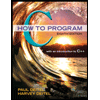
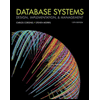
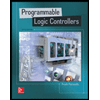