Problem A straight line can be defined by a pair of points pi(xX1, y1) and p2(x2, y2). The slope m of a line is defined as follow: Y2 - yı m = X2 - x1 Your program reads the points from a text file called 'points.txt' which contains the coordinates of unknown number of pairs of points as shown in Figure 1. Each line contains four values x1, y1, x2, y2, where x1, yl are the coordinates of the first point and x2, y2 are the coordinates of the second point. -2 15.5 10 7.5 -3.2 -4.6 12.5 4 21 -2 3p 6. 2 -3 -6 10 3 10 15 Figure 1. Input file contains unknown number of point pairs Use Spider, to create the following files: (i) (ii) The input file 'points.txt' shown in Figure 1. Your Python program that reads from the input file 'points.txt', the coordinates of unknown number of pairs of points, computes the corresponding slopes then prints the results on the screen as shown in Figure 2. ====== ==== ======= Line # X1 Y1 X2 Y2 slope ===: ==== 1 10.00 -2.00 7.50 -3.20 0.48 2 4.00 15.50 -4.60 21.00 -0.64 3 0.00 0.00 0.00 0.00 inf 4 2.00 5.00 -3.00 -6.00 2.20 5 10.00 3.00 10.00 15.00 inf ==== Figure 2. The output on screen Your program must define and use the following functions: 1. printHeader(...): prints on screen the three lines header of the table as shown in the sample output (Fig. 2). 2. getCoordinates(….): receives one parameter representing a text line read from the input file, and it returns four float values x1, y1, x2, y2. In case of any wrong value format, the function returns 0.0, 0.0, 0.0, 0.0. 3. computeSlope(...): receives four parameters representing the values x1, y1, x2, y2 and returns the slope value m that should be computed according to the given formula above. If x2 is equal to x1 the computeSlope function returns an infinite value. In Python, the infinite value is a float constant value called inf (available in math library). Thus, you need to import inf from the math library as follows: from math import inf 4. main(...): the main function receives the name of the input file 'points.txt'. This function opens the input file for reading, reads data from it, calls the various functions and finally prints the results on screen. If the input file is not found, an exception is raised and an error message will be displayed.
Problem A straight line can be defined by a pair of points pi(xX1, y1) and p2(x2, y2). The slope m of a line is defined as follow: Y2 - yı m = X2 - x1 Your program reads the points from a text file called 'points.txt' which contains the coordinates of unknown number of pairs of points as shown in Figure 1. Each line contains four values x1, y1, x2, y2, where x1, yl are the coordinates of the first point and x2, y2 are the coordinates of the second point. -2 15.5 10 7.5 -3.2 -4.6 12.5 4 21 -2 3p 6. 2 -3 -6 10 3 10 15 Figure 1. Input file contains unknown number of point pairs Use Spider, to create the following files: (i) (ii) The input file 'points.txt' shown in Figure 1. Your Python program that reads from the input file 'points.txt', the coordinates of unknown number of pairs of points, computes the corresponding slopes then prints the results on the screen as shown in Figure 2. ====== ==== ======= Line # X1 Y1 X2 Y2 slope ===: ==== 1 10.00 -2.00 7.50 -3.20 0.48 2 4.00 15.50 -4.60 21.00 -0.64 3 0.00 0.00 0.00 0.00 inf 4 2.00 5.00 -3.00 -6.00 2.20 5 10.00 3.00 10.00 15.00 inf ==== Figure 2. The output on screen Your program must define and use the following functions: 1. printHeader(...): prints on screen the three lines header of the table as shown in the sample output (Fig. 2). 2. getCoordinates(….): receives one parameter representing a text line read from the input file, and it returns four float values x1, y1, x2, y2. In case of any wrong value format, the function returns 0.0, 0.0, 0.0, 0.0. 3. computeSlope(...): receives four parameters representing the values x1, y1, x2, y2 and returns the slope value m that should be computed according to the given formula above. If x2 is equal to x1 the computeSlope function returns an infinite value. In Python, the infinite value is a float constant value called inf (available in math library). Thus, you need to import inf from the math library as follows: from math import inf 4. main(...): the main function receives the name of the input file 'points.txt'. This function opens the input file for reading, reads data from it, calls the various functions and finally prints the results on screen. If the input file is not found, an exception is raised and an error message will be displayed.
Chapter13: File Input And Output
Section: Chapter Questions
Problem 6PE
Related questions
Topic Video
Question
100%
Please use python not java or C++
A straight line can be defined by a pair of points p1(x1, y1) and p2(x2, y2). The slope m of a line is defined
as follow:
? = ?% − ?' ?% − ?'
Your program reads the points from a text file called ‘points.txt’ which contains the coordinates of unknown number of pairs of points as shown in Figure 1. Each line contains four values x1, y1, x2, y2, where x1, y1 are the coordinates of the first point and x2, y2 are the coordinates of the second point.
Figure 1. Input file contains unknown number of point pairs
Use Spider, to create the following files:
(i) The input file ‘points.txt’ shown in Figure 1.
(ii) Your Python program that reads from the input file ‘points.txt’, the coordinates of unknown
number of pairs of points, computes the corresponding slopes then prints the results on the screen as shown in Figure 2.
Figure 2. The output on screen
Your program must define and use the following functions:
1. printHeader(...): prints on screen the three lines header of the table as shown in the sample output (Fig. 2).
2. getCoordinates(...): receives one parameter representing a text line read from the input file, and it returns four float values x1, y1, x2, y2. In case of any wrong value format, the function returns 0.0, 0.0, 0.0, 0.0.
3. computeSlope(...): receives four parameters representing the values x1, y1, x2, y2 and returns the slope value m that should be computed according to the given formula above. If x2 is equal to x1 the computeSlope function returns an infinite value. In Python, the infinite value is a float constant value called inf (available in math library). Thus, you need to import inf from the math library as follows:
from math import inf
4. main(...): the main function receives the name of the input file ‘points.txt’. This function opens the input file for reading, reads data from it, calls the various functions and finally prints the results on screen. If the input file is not found, an exception is raised and an error message will be displayed.
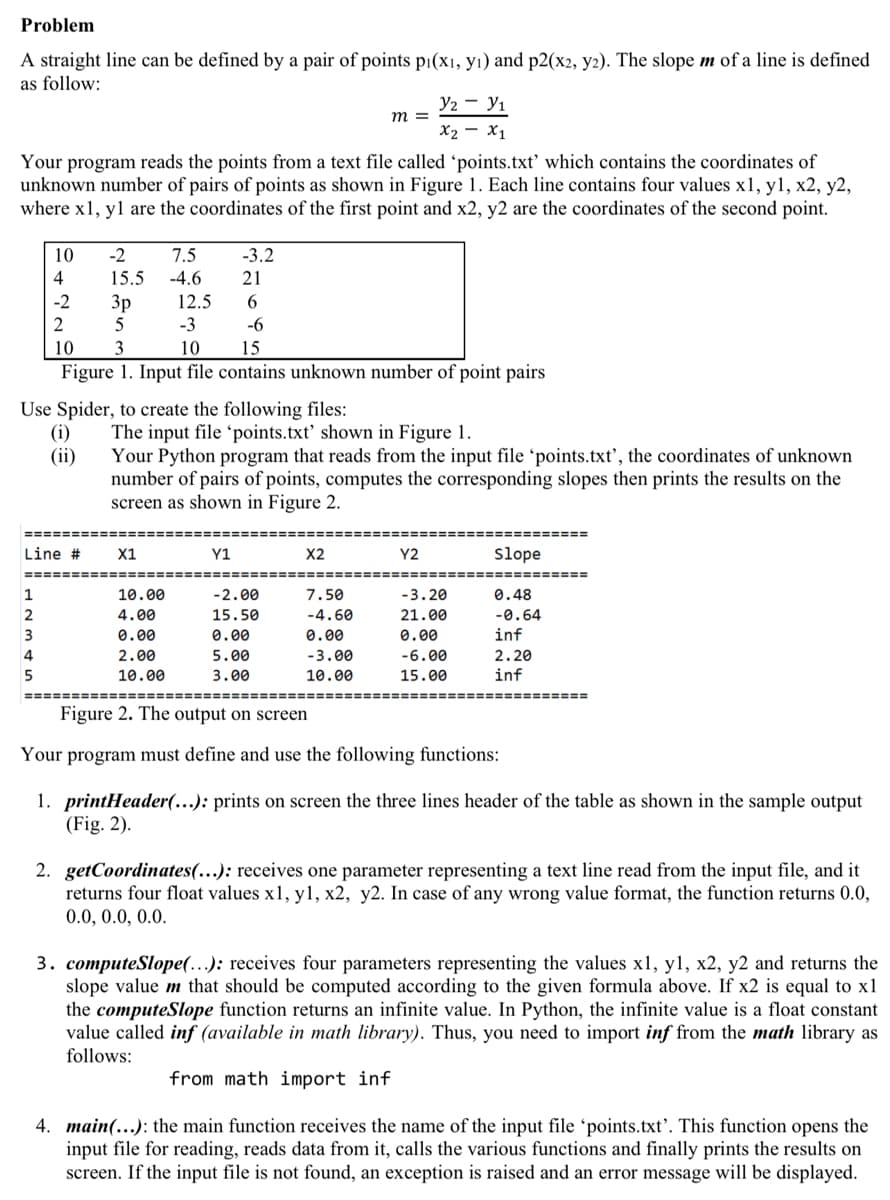
Transcribed Image Text:Problem
A straight line can be defined by a pair of points pi(x1, yı) and p2(x2, y2). The slope m of a line is defined
as follow:
Y2 - y1
m =
X2 - X1
Your program reads the points from a text file called 'points.txt' which contains the coordinates of
unknown number of pairs of points as shown in Figure 1. Each line contains four values x1, y1, x2, y2,
where x1, yl are the coordinates of the first point and x2, y2 are the coordinates of the second point.
10
-2
7.5
-3.2
4
15.5
-4.6
21
-2
Зр
5
12.5
6.
2
-3
-6
10
3
10
15
Figure 1. Input file contains unknown number of point pairs
Use Spider, to create the following files:
(i)
(ii)
The input file 'points.txt' shown in Figure 1.
Your Python program that reads from the input file 'points.txt', the coordinates of unknown
number of pairs of points, computes the corresponding slopes then prints the results on the
screen as shown in Figure 2.
Line #
X1
Y1
X2
Y2
Slope
1
10.00
-2.00
7.50
-3.20
0.48
2
4.00
15.50
-4.60
21.00
-0.64
0.00
0.00
0.00
0.00
inf
2.20
inf
4
2.00
5.00
-3.00
-6.00
10.00
3.00
10.00
15.00
Figure 2. The output on screen
Your program must define and use the following functions:
1. printHeader(...): prints on screen the three lines header of the table as shown in the sample output
(Fig. 2).
2. getCoordinates(...): receives one parameter representing a text line read from the input file, and it
returns four float values x1, y1, x2, y2. In case of any wrong value format, the function returns 0.0,
0.0, 0.0, 0.0.
3. computeSlope(.…): receives four parameters representing the values x1, y1, x2, y2 and returns the
slope value m that should be computed according to the given formula above. If x2 is equal to x1
the computeSlope function returns an infinite value. In Python, the infinite value is a float constant
value called inf (available in math library). Thus, you need to import inf from the math library as
follows:
from math import inf
4. main(...): the main function receives the name of the input file 'points.txt'. This function opens the
input file for reading, reads data from it, calls the various functions and finally prints the results on
screen. If the input file is not found, an exception is raised and an error message will be displayed.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
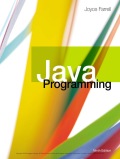
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
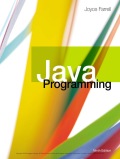
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT