You are asked to write a text analyzer program that reads a text file and counts the number of lines, number of words (for example, King′s counts as one word) and the frequency of occurrence of each of the 26 letters of the alphabet. NOTE: • We ignore case: Treat upper and lowercase letters to be the same. • Assume that there is at least one space between words on the same line. • Only characters A-Z are to be counted. For example, if the text file TextAnalyzerData contained this text shown below: Baa, baa, black sheep Have you any wool? Yes sir, yes sir Three bags full. One for my master And one for the dame One for the little boy Who lives down the lane. The Analyzer should print the following information: Number of Lines: 8 Number of Words: 34 Letter Counts Frequency of A: 12 Frequency of B: 5 Frequency of C: 1 Frequency of D: 3 Frequency of E: 18 Frequency of F: 4 Frequency of G: 1 Frequency of H: 7 Frequency of I: 4 Frequency of J: 0 Frequency of K: 1 Frequency of L: 8 Frequency of M: 3 Frequency of N: 7 Frequency of O: 12 Frequency of P: 1 Frequency of Q: 0 Frequency of R: 7 Frequency of S: 8 Frequency of T: 7 Frequency of U: 2 Frequency of V: 2 Frequency of W: 3 Frequency of X: 0 Frequency of Y: 6 Frequency of Z: 0 SO HOW SHOULD YOU WRITE THIS PROGRAM? To begin, you need to write a Java class called TextAnalyzer. When thinking about writing this class, note that it must meet the following specifications or requirements: • The Java class TextAnalyzer should have the following instance variables (sometimes called attributes): private int lineCount; private int wordCount; private int[] frequencies = new int[26]; • The Java class TextAnalyzer should have ″get″ methods (getter methods) for each of the instance variables – e.g., it should have this method: public int getLineCount() { return lineCount; } Note about how we form the name of the ″get″ method. When we create the instance variable name, the name starts with lower case. For example, suppose the variable name was lineCount. Note also the ‘camel case’ as Java expects – note the first lower case ′l′ in the variable name. However, the getter method′s name already begins with ′get′ by convention, and is written as ′getLineCount()′, where the lower case ′l′ in the variable name is transformed to an upper case ′L′. You should use an identical style in all the getter methods you write. • The Java class called TextAnalyzer should have a method called: analyzeText(String fileName) that o Opens the specified file o Reads the data line by line. After it reads each line, the method should update the value in variable: lineCount o Splits the line it had just read into words. After this splitting, the method should count the number of words generated. How can it most easily do that? Once you figure this out, use this count to update the value in variable: wordCount o Scans the line just read character by character and updates the frequency count for each character. Remember: Only alphabetical characters ′A′ through ′Z′ are counted; lower-case letters are mapped to upper-case letters, and all other characters are ignored. Think: what is the best way to scan the line just read? Note that the line just read goes into a string, right? Scanning means looking at each character of the string containing the contents of the line. Which of the various string methods we discussed above is most suitable for looking at each character of the line? o Now, after figuring out how you will look up each character of the line, you need to figure out how to accomplish the following: suppose the character you just accessed from the line is ′A′ or ′a′. This means that in the frequencies array that you have declared in your instance variables, the value in frequencies[0]will be increased by 1. If the next character you accessed is a ′D′ or ′d′, then the value in frequencies[3]will be increased by 1. Similarly, on reading ′Z′ or ′z′, you will increase the value in frequencies[25]by 1. Think of how to do this? You could use an ′if′ or ′switch′ statement of course, but work with your partner, and think (google if necessary) to figure out how you can do this more efficiently.
You are asked to write a text analyzer program that reads a text file and counts the number of lines, number of words (for example, King′s counts as one word) and the frequency of occurrence of each of the 26 letters of the alphabet. NOTE: • We ignore case: Treat upper and lowercase letters to be the same. • Assume that there is at least one space between words on the same line. • Only characters A-Z are to be counted. For example, if the text file TextAnalyzerData contained this text shown below: Baa, baa, black sheep Have you any wool? Yes sir, yes sir Three bags full. One for my master And one for the dame One for the little boy Who lives down the lane. The Analyzer should print the following information: Number of Lines: 8 Number of Words: 34 Letter Counts Frequency of A: 12 Frequency of B: 5 Frequency of C: 1 Frequency of D: 3 Frequency of E: 18 Frequency of F: 4 Frequency of G: 1 Frequency of H: 7 Frequency of I: 4 Frequency of J: 0 Frequency of K: 1 Frequency of L: 8 Frequency of M: 3 Frequency of N: 7 Frequency of O: 12 Frequency of P: 1 Frequency of Q: 0 Frequency of R: 7 Frequency of S: 8 Frequency of T: 7 Frequency of U: 2 Frequency of V: 2 Frequency of W: 3 Frequency of X: 0 Frequency of Y: 6 Frequency of Z: 0 SO HOW SHOULD YOU WRITE THIS PROGRAM? To begin, you need to write a Java class called TextAnalyzer. When thinking about writing this class, note that it must meet the following specifications or requirements: • The Java class TextAnalyzer should have the following instance variables (sometimes called attributes): private int lineCount; private int wordCount; private int[] frequencies = new int[26]; • The Java class TextAnalyzer should have ″get″ methods (getter methods) for each of the instance variables – e.g., it should have this method: public int getLineCount() { return lineCount; } Note about how we form the name of the ″get″ method. When we create the instance variable name, the name starts with lower case. For example, suppose the variable name was lineCount. Note also the ‘camel case’ as Java expects – note the first lower case ′l′ in the variable name. However, the getter method′s name already begins with ′get′ by convention, and is written as ′getLineCount()′, where the lower case ′l′ in the variable name is transformed to an upper case ′L′. You should use an identical style in all the getter methods you write. • The Java class called TextAnalyzer should have a method called: analyzeText(String fileName) that o Opens the specified file o Reads the data line by line. After it reads each line, the method should update the value in variable: lineCount o Splits the line it had just read into words. After this splitting, the method should count the number of words generated. How can it most easily do that? Once you figure this out, use this count to update the value in variable: wordCount o Scans the line just read character by character and updates the frequency count for each character. Remember: Only alphabetical characters ′A′ through ′Z′ are counted; lower-case letters are mapped to upper-case letters, and all other characters are ignored. Think: what is the best way to scan the line just read? Note that the line just read goes into a string, right? Scanning means looking at each character of the string containing the contents of the line. Which of the various string methods we discussed above is most suitable for looking at each character of the line? o Now, after figuring out how you will look up each character of the line, you need to figure out how to accomplish the following: suppose the character you just accessed from the line is ′A′ or ′a′. This means that in the frequencies array that you have declared in your instance variables, the value in frequencies[0]will be increased by 1. If the next character you accessed is a ′D′ or ′d′, then the value in frequencies[3]will be increased by 1. Similarly, on reading ′Z′ or ′z′, you will increase the value in frequencies[25]by 1. Think of how to do this? You could use an ′if′ or ′switch′ statement of course, but work with your partner, and think (google if necessary) to figure out how you can do this more efficiently.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter5: Repetition Statements
Section5.5: A Closer Look: Loop Programming Techniques
Problem 12E: (Program) Write a program that tests the effectiveness of the rand() library function. Start by...
Related questions
Topic Video
Question
The problem:
You are asked to write a text analyzer program that reads a text file and counts the number of lines, number of words (for example, King′s counts as one word) and the frequency of occurrence of each of the 26 letters of the alphabet.
NOTE:
• We ignore case: Treat upper and lowercase letters to be the same.
• Assume that there is at least one space between words on the same line.
• Only characters A-Z are to be counted.
For example, if the text file TextAnalyzerData contained this text shown below:
Baa, baa, black sheep
Have you any wool?
Yes sir, yes sir
Three bags full.
One for my master
And one for the dame
One for the little boy
Who lives down the lane.
The Analyzer should print the following information:
Number of Lines: 8
Number of Words: 34
Letter Counts
Frequency of A: 12
Frequency of B: 5
Frequency of C: 1
Frequency of D: 3
Frequency of E: 18
Frequency of F: 4
Frequency of G: 1
Frequency of H: 7
Frequency of I: 4
Frequency of J: 0
Frequency of K: 1
Frequency of L: 8
Frequency of M: 3
Frequency of N: 7
Frequency of O: 12
Frequency of P: 1
Frequency of Q: 0
Frequency of R: 7
Frequency of S: 8
Frequency of T: 7
Frequency of U: 2
Frequency of V: 2
Frequency of W: 3
Frequency of X: 0
Frequency of Y: 6
Frequency of Z: 0
SO HOW SHOULD YOU WRITE THIS PROGRAM?
To begin, you need to write a Java class called TextAnalyzer. When thinking about writing this class, note that it must meet the following specifications or requirements:
• The Java class TextAnalyzer should have the following instance variables (sometimes called attributes):
private int lineCount;
private int wordCount;
private int[] frequencies = new int[26];
• The Java class TextAnalyzer should have ″get″ methods (getter methods) for each of the instance variables – e.g., it should have this method:
public int getLineCount()
{
return lineCount;
}
Note about how we form the name of the ″get″ method. When we create the instance variable name, the name starts with lower case. For example, suppose the variable name was lineCount. Note also the ‘camel case’ as Java expects – note the first lower case ′l′ in the variable name. However, the getter method′s name already begins with ′get′ by convention, and is written as ′getLineCount()′, where the lower case ′l′ in the variable name is transformed to an upper case ′L′. You should use an identical style in all the getter methods you write.
• The Java class called TextAnalyzer should have a method called: analyzeText(String fileName) that
o Opens the specified file
o Reads the data line by line. After it reads each line, the method should update the value in variable: lineCount
o Splits the line it had just read into words. After this splitting, the method should count the number of words generated. How can it most easily do that? Once you figure this out, use this count to update the value in variable: wordCount
o Scans the line just read character by character and updates the frequency count for each character. Remember: Only alphabetical characters ′A′ through ′Z′ are counted; lower-case letters are mapped to upper-case letters, and all other characters are ignored. Think: what is the best way to scan the line just read? Note that the line just read goes into a string, right? Scanning means looking at each character of the string containing the contents of the line. Which of the various string methods we discussed above is most suitable for looking at each character of the line?
o Now, after figuring out how you will look up each character of the line, you need to figure out how to accomplish the following: suppose the character you just accessed from the line is ′A′ or ′a′. This means that in the frequencies array that you have declared in your instance variables, the value in frequencies[0]will be increased by 1. If the next character you accessed is a ′D′ or ′d′, then the value in frequencies[3]will be increased by 1. Similarly, on reading ′Z′ or ′z′, you will increase the value in frequencies[25]by 1. Think of how to do this? You could use an ′if′ or ′switch′ statement of course, but work with your partner, and think (google if necessary) to figure out how you can do this more efficiently.
Use the following TextAnalyzerTester class to test your program:
package lab02;
import java.util.Scanner;
public class TextAnalyzerTester {
public static void main(String[] args) {
TextAnalyzer ta = new TextAnalyzer();
Scanner sc = new Scanner(System.in);
System.out.println("Enter the name of the data file: ");
String filename = sc.next();
ta.analyzeText(filename);
System.out.println("Number of Lines: " + ta.getLineCount());
System.out.println("Number of Words: " + ta.getWordCount());
int[] freq = ta.getFrequencies();
for (int i = 0; i< freq.length; i++) {
System.out.print("Frequency of " + (char)('A' + i) +": " + freq[i] + "\n");
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 14 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
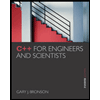
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
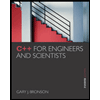
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr