Problem: Create a base class called Vehicle that has the manufacturer’s name (typeString), number of cylinders in the engine (type int), and the owner (type Person). Use thePerson class developed this semester. Create a class automobile that is derived fromVehicle and has additional properties: number of passengers (type int) and weight in tons(type double). Create a class Truck which is derived from Vehicle and has additionalproperties: the load capacity in tons (type double, since it may contain a fractional part)and towing capacity in tons (type double). The classes should have:• Two constructors, a default and an overloaded constructor• All appropriate accessor and mutator methods (getters and setters).• An ‘equals’ method (must conform to the Person example and the Object class ).• A ‘toString’ method• A ‘copy’ constructor• A ‘clone’ method• A ‘finalize’ method Write a driver (client/test) class that tests ALL the methods. Be sure to invoke each of the constructors, and ALL methods. Demonstrate polymorphism by creating an array of various types of vehicles with varying property values. ----------------------------------------------------------------------------------- person class: // ------------------------------------------------// Person.java// ------------------------------------------------ public class Person {// Class data items - instance variablesprivate StringBuffer firstName;private StringBuffer lastName;private int age;private StringBuffer ssn;// Class methodspublic void setFirstName ( String x ) { firstName = new StringBuffer ( x ); }public StringBuffer getFirstName () { return firstName; }public void setLastName ( String x ) { lastName = new StringBuffer ( x ); }public StringBuffer getLastName () { return lastName; }public void setAge ( int a ) { age =a; }public int getAge ( ) { return age; }public void setSsn ( String x ) { ssn = new StringBuffer ( x ); }public StringBuffer getSsn () { return ssn; }public Person ( ) {this ( " ", " ", 0, " " );System.out.println ( "Person - default, no-arg constructor" );}public Person ( String fn, String ln, int a, String s ) {firstName = new StringBuffer ( fn );lastName = new StringBuffer ( ln );age = a;ssn = new StringBuffer ( s );System.out.println ( "Person - overloaded, 4-arg constructor" );}public String toString ( ){String x = " " + firstName + " " + lastName + " " + age + " " + ssn + " ";return x;} public boolean equals ( Object obj ) {if ( this == obj ) return true; // They're the same objectsif ( obj == null ) return false; // Argument is nullif ( getClass( ) != obj.getClass( ) ) return false; Person d = ( Person ) obj; if ( ( firstName.toString().equals ( d.firstName.toString() ) ) &&( lastName. toString().equals ( d.lastName. toString() ) ) &&( age == d.age ) ){return true;}else{return false;}}public void finalize ( ) {System.out.println ( "Person - finalize method" );}} ------------------------------------------------------------------------------------no dispose method -no finalize
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Problem: Create a base class called Vehicle that has the manufacturer’s name (type
String), number of cylinders in the engine (type int), and the owner (type Person). Use the
Person class developed this semester. Create a class automobile that is derived from
Vehicle and has additional properties: number of passengers (type int) and weight in tons
(type double). Create a class Truck which is derived from Vehicle and has additional
properties: the load capacity in tons (type double, since it may contain a fractional part)
and towing capacity in tons (type double). The classes should have:
• Two constructors, a default and an overloaded constructor
• All appropriate accessor and mutator methods (getters and setters).
• An ‘equals’ method (must conform to the Person example and the Object class ).
• A ‘toString’ method
• A ‘copy’ constructor
• A ‘clone’ method
• A ‘finalize’ method
Write a driver (client/test) class that tests ALL the methods. Be sure to invoke each of the constructors, and ALL methods. Demonstrate polymorphism by creating an array of various types of vehicles with varying property values.
-----------------------------------------------------------------------------------
person class:
// ------------------------------------------------
// Person.java
// ------------------------------------------------
public class Person {
// Class data items - instance variables
private StringBuffer firstName;
private StringBuffer lastName;
private int age;
private StringBuffer ssn;
// Class methods
public void setFirstName ( String x ) { firstName = new StringBuffer ( x ); }
public StringBuffer getFirstName () { return firstName; }
public void setLastName ( String x ) { lastName = new StringBuffer ( x ); }
public StringBuffer getLastName () { return lastName; }
public void setAge ( int a ) { age =a; }
public int getAge ( ) { return age; }
public void setSsn ( String x ) { ssn = new StringBuffer ( x ); }
public StringBuffer getSsn () { return ssn; }
public Person ( ) {
this ( " ", " ", 0, " " );
System.out.println ( "Person - default, no-arg constructor" );
}
public Person ( String fn, String ln, int a, String s ) {
firstName = new StringBuffer ( fn );
lastName = new StringBuffer ( ln );
age = a;
ssn = new StringBuffer ( s );
System.out.println ( "Person - overloaded, 4-arg constructor" );
}
public String toString ( ){
String x = " " + firstName + " " + lastName + " " + age + " " + ssn + " ";
return x;
}
public boolean equals ( Object obj ) {
if ( this == obj ) return true; // They're the same objects
if ( obj == null ) return false; // Argument is null
if ( getClass( ) != obj.getClass( ) ) return false;
Person d = ( Person ) obj;
if ( ( firstName.toString().equals ( d.firstName.toString() ) ) &&
( lastName. toString().equals ( d.lastName. toString() ) ) &&
( age == d.age ) )
{
return true;
}
else
{
return false;
}
}
public void finalize ( ) {
System.out.println ( "Person - finalize method" );
}
}
------------------------------------------------------------------------------------no dispose method
-no finalize

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

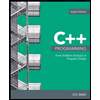
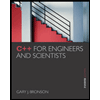
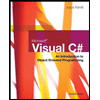
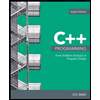
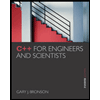
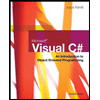