PROBLEM: When creating account: It should be 6 digit pin. (if there is a letter or not 6 digits were put in, output will say "Please retry. It should be a 6 digit pin."). It should also have an authenticator for the pin and if the inputted pin is wrong show "Invalid Pin. Please try again." and if it is still wrong in 3 tries, it'll say "Too many incorrect tries. Exiting program." CODE: import random import sys class ATM(): def __init__(customer, name, account_number, balance = 0): customer.name = name customer.account_number = account_number customer.balance = balance def account_detail(customer): print("\n-ACCOUNT DETAIL-") print(f"Account Holder: {customer.name.upper()}") print(f"Account Number: {customer.account_number}") print(f"Available balance: Nu.{customer.balance}\n") def deposit(customer, amount): customer.amount = amount customer.balance = customer.balance + customer.amount if amount >= 8000: print("Your balance is Above PHP 8000") print("Current account balance: ₱", customer.balance) def withdraw(customer, amount): customer.amount = amount if customer.amount > customer.balance: print("Insufficient fund!") print(f"Your balance is PHP{customer.balance} only.") print("Try with lesser amount than balance.") print() else: customer.balance = customer.balance - customer.amount print(f"Nu.{amount} withdrawal successful!") if customer.balance < 8000: print("Your balance is Below PHP 8000") print("Current account balance: ₱", customer.balance) print() def check_balance(customer): if customer.balance >= 8000: print("Your balance is Above PHP 8000") else: print("Your balance is Below PHP 8000") print("Available balance: ₱", customer.balance) print() def transaction(customer): print(""" TRANSACTION ********************* Menu: 1. Account Detail 2. Check Balance 3. Deposit 4. Withdraw 5. Exit ********************* """) while True: try: option = int(input("Enter 1, 2, 3, 4 or 5:")) except: print("Error: Enter 1, 2, 3, 4, or 5 only.\n") continue else: if option == 1: atm.account_detail() elif option == 2: atm.check_balance() elif option == 3: amount = int(input("How much you want to deposit(₱.):")) atm.deposit(amount) elif option == 4: amount = int(input("How much you want to withdraw(₱.):")) atm.withdraw(amount) elif option == 5: print(f""" printing receipt.............. ************************************************************ | • Transaction is now complete. | • Transaction number: {random.randint(10000, 1000000)} | • Account holder: {customer.name.upper()} | • Account number: {customer.account_number} | • Available balance: ₱.{customer.balance} | | Thanks for choosing us as your bank ☺ ************************************************************ """) sys.exit() print(""" |=================== Welcome Customer! ==================| |==============- Automated Teller Machine -===========| """) print("----------ACCOUNT CREATION----------") name = input("Enter your name: ") account_number = input("Enter your account number: ") print("Congratulations! Account created successfully......\n") atm = ATM(name, account_number) while True: print("|-------------TRANSACTION-------------|") trans = input("Do you want to do any transaction?(yes/no):") if trans == "yes": atm.transaction() elif trans == "no": print(""" ------------------------------------- | Thanks for choosing us as your bank | Visit us again! ------------------------------------- """) break else: print("Wrong command! 'Enter yes' or 'no'.\n")
PROBLEM: When creating account: It should be 6 digit pin. (if there is a letter or not 6 digits were put in, output will say "Please retry. It should be a 6 digit pin."). It should also have an authenticator for the pin and if the inputted pin is wrong show "Invalid Pin. Please try again." and if it is still wrong in 3 tries, it'll say "Too many incorrect tries. Exiting program." CODE: import random import sys class ATM(): def __init__(customer, name, account_number, balance = 0): customer.name = name customer.account_number = account_number customer.balance = balance def account_detail(customer): print("\n-ACCOUNT DETAIL-") print(f"Account Holder: {customer.name.upper()}") print(f"Account Number: {customer.account_number}") print(f"Available balance: Nu.{customer.balance}\n") def deposit(customer, amount): customer.amount = amount customer.balance = customer.balance + customer.amount if amount >= 8000: print("Your balance is Above PHP 8000") print("Current account balance: ₱", customer.balance) def withdraw(customer, amount): customer.amount = amount if customer.amount > customer.balance: print("Insufficient fund!") print(f"Your balance is PHP{customer.balance} only.") print("Try with lesser amount than balance.") print() else: customer.balance = customer.balance - customer.amount print(f"Nu.{amount} withdrawal successful!") if customer.balance < 8000: print("Your balance is Below PHP 8000") print("Current account balance: ₱", customer.balance) print() def check_balance(customer): if customer.balance >= 8000: print("Your balance is Above PHP 8000") else: print("Your balance is Below PHP 8000") print("Available balance: ₱", customer.balance) print() def transaction(customer): print(""" TRANSACTION ********************* Menu: 1. Account Detail 2. Check Balance 3. Deposit 4. Withdraw 5. Exit ********************* """) while True: try: option = int(input("Enter 1, 2, 3, 4 or 5:")) except: print("Error: Enter 1, 2, 3, 4, or 5 only.\n") continue else: if option == 1: atm.account_detail() elif option == 2: atm.check_balance() elif option == 3: amount = int(input("How much you want to deposit(₱.):")) atm.deposit(amount) elif option == 4: amount = int(input("How much you want to withdraw(₱.):")) atm.withdraw(amount) elif option == 5: print(f""" printing receipt.............. ************************************************************ | • Transaction is now complete. | • Transaction number: {random.randint(10000, 1000000)} | • Account holder: {customer.name.upper()} | • Account number: {customer.account_number} | • Available balance: ₱.{customer.balance} | | Thanks for choosing us as your bank ☺ ************************************************************ """) sys.exit() print(""" |=================== Welcome Customer! ==================| |==============- Automated Teller Machine -===========| """) print("----------ACCOUNT CREATION----------") name = input("Enter your name: ") account_number = input("Enter your account number: ") print("Congratulations! Account created successfully......\n") atm = ATM(name, account_number) while True: print("|-------------TRANSACTION-------------|") trans = input("Do you want to do any transaction?(yes/no):") if trans == "yes": atm.transaction() elif trans == "no": print(""" ------------------------------------- | Thanks for choosing us as your bank | Visit us again! ------------------------------------- """) break else: print("Wrong command! 'Enter yes' or 'no'.\n")
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
PROBLEM:
When creating account:
It should be 6 digit pin. (if there is a letter or not 6 digits were put in, output will say "Please retry. It should be a 6 digit pin."). It should also have an authenticator for the pin and if the inputted pin is wrong show "Invalid Pin. Please try again." and if it is still wrong in 3 tries, it'll say "Too many incorrect tries. Exiting program."
CODE:
import random
import sys
class ATM():
def __init__(customer, name, account_number, balance = 0):
customer.name = name
customer.account_number = account_number
customer.balance = balance
def account_detail(customer):
print("\n-ACCOUNT DETAIL-")
print(f"Account Holder: {customer.name.upper()}")
print(f"Account Number: {customer.account_number}")
print(f"Available balance: Nu.{customer.balance}\n")
def deposit(customer, amount):
customer.amount = amount
customer.balance = customer.balance + customer.amount
if amount >= 8000:
print("Your balance is Above PHP 8000")
print("Current account balance: ₱", customer.balance)
def withdraw(customer, amount):
customer.amount = amount
if customer.amount > customer.balance:
print("Insufficient fund!")
print(f"Your balance is PHP{customer.balance} only.")
print("Try with lesser amount than balance.")
print()
else:
customer.balance = customer.balance - customer.amount
print(f"Nu.{amount} withdrawal successful!")
if customer.balance < 8000:
print("Your balance is Below PHP 8000")
print("Current account balance: ₱", customer.balance)
print()
def check_balance(customer):
if customer.balance >= 8000:
print("Your balance is Above PHP 8000")
else:
print("Your balance is Below PHP 8000")
print("Available balance: ₱", customer.balance)
print()
def transaction(customer):
print("""
TRANSACTION
*********************
Menu:
1. Account Detail
2. Check Balance
3. Deposit
4. Withdraw
5. Exit
*********************
""")
while True:
try:
option = int(input("Enter 1, 2, 3, 4 or 5:"))
except:
print("Error: Enter 1, 2, 3, 4, or 5 only.\n")
continue
else:
if option == 1:
atm.account_detail()
elif option == 2:
atm.check_balance()
elif option == 3:
amount = int(input("How much you want to deposit(₱.):"))
atm.deposit(amount)
elif option == 4:
amount = int(input("How much you want to withdraw(₱.):"))
atm.withdraw(amount)
elif option == 5:
print(f"""
printing receipt..............
************************************************************
| • Transaction is now complete.
| • Transaction number: {random.randint(10000, 1000000)}
| • Account holder: {customer.name.upper()}
| • Account number: {customer.account_number}
| • Available balance: ₱.{customer.balance}
|
| Thanks for choosing us as your bank ☺
************************************************************
""")
sys.exit()
print("""
|=================== Welcome Customer! ==================|
|==============- Automated Teller Machine -===========|
""")
print("----------ACCOUNT CREATION----------")
name = input("Enter your name: ")
account_number = input("Enter your account number: ")
print("Congratulations! Account created successfully......\n")
atm = ATM(name, account_number)
while True:
print("|-------------TRANSACTION-------------|")
trans = input("Do you want to do any transaction?(yes/no):")
if trans == "yes":
atm.transaction()
elif trans == "no":
print("""
-------------------------------------
| Thanks for choosing us as your bank
| Visit us again!
-------------------------------------
""")
break
else:
print("Wrong command! 'Enter yes' or 'no'.\n")
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Recommended textbooks for you
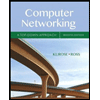
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
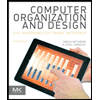
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
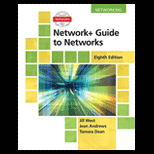
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
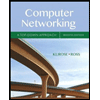
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
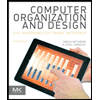
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
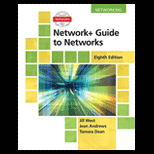
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
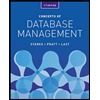
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
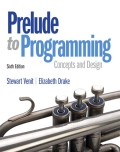
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
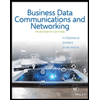
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY