PROGRAM DESCRIPTION A new video store in your neighborhood is about to open. However, it does not have a program to keep track of its videos and customers. The store managers want someone to write a program for their system so that the video store can operate. The program will require you to design 2 ADTs as described below: [1] VIDEO ADT Data Operations Video_ID (preferably int, auto-generated) Movie Title Genre Production Number of Copies [1] Insert a new video [2] Rent a video; that is, check out a video [3] Return a video, or check in, a video [4] Show the details of a particular video [5] Display all videos in the store [6] Check whether a particular video is in the store [2] CUSTOMER PARENT ADT Data Operations Customer_ID (preferably int, auto-generated) Name Address [1] Add Customer [2] Show the customer details [3] Print list of all customers [3] CUSTOMER-RENT CHILD ADT Customer_ID ( Video_ID (of all rented videos of a customer) [1] Rent a Video (Add to the video ids rented) [2] Return a Video (Remove the video id from the list) [3] Print list of all videos rented by each customer The program will require you to maintain 3 text files with specifications below: Text File Requirements [1] VIDEO Text File [1] Will store the information about the Videos [2] Should contain the following by default: 5 Horror Movies 5 Romance Movies 5 Sci-Fi Movies 5 Action Movies 5 Comedy Movies **Must be authentic and true.** [2] CUSTOMER Text File [1] Will store the basic information about the Customers [2] Should contain at least 10 customers by default **Must be authentic and true.** [3] CUSTOMER-RENT [1] Will store all customers that RENT a VIDEO [2] Will store all the Video_IDs of all rented videos [3] Will delete Video_IDs and Customer_ID when videos are returned NOTE: IF YOU FIND THE NEED TO ADD OR MAKE ALTERATIONS TO THE SPECIFICATIONS OF THE ADT AND TEXT FILES ABOVE, PLEASE DO SO. JUST MAKE SURE THAT YOU HAVE AN ACCEPTABLE LOGICAL REASON. INSTRUCTIONS: The program will have the following options/menus: [1] New Video //Programmer1 [2] Rent a Video //Lead Programmer [3] Return a Video //Lead Programmer [4] Show Video Details //Programmer2 [5] Display all Videos //Programmer1 [6] Check Video Availability //Programmer2 [7] Customer Maintenance [1] Add New Customer //Programmer1 [2] Show Customer Details //Programmer2 [3] List of Videos Rented by a Customer //Lead Programmer [8] Exit Program //Programmer1 Follow the suggested screen dialogs below for each of the option above: OTHER REQUIREMENTS: You must store your videos in a linked list when you retrieved them from the text file. They must also be stored in a linked list data structure during processing. Saving back to the text file will be done when the user chooses [8] Exit Program. You must store you customers in a queue when you retrieved them from the text file. They must also be stored in a queue during processing. Saving back to the text file will be done when the user chooses [8] Exit Program. Rented videos will be stored in a stack and will be saved in the CUSTOMER-RENT text file when the user chooses [8] Exit Program. Use data structures, files, functions, ADTs, STLs and algorithms in your program. Put necessary comments to your program. Provide error messages whenever necessary.
A new video store in your neighborhood is about to open. However, it does not have a program to keep track of its videos and customers. The store managers want someone to write a program for their system so that the video store can operate.
The program will require you to design 2 ADTs as described below:
[1] VIDEO ADT |
|
Data |
Operations |
Video_ID (preferably int, auto-generated) Movie Title Genre Production Number of Copies
|
[1] Insert a new video [2] Rent a video; that is, check out a video [3] Return a video, or check in, a video [4] Show the details of a particular video [5] Display all videos in the store [6] Check whether a particular video is in the store
|
[2] CUSTOMER PARENT ADT |
|
Data |
Operations |
Customer_ID (preferably int, auto-generated) Name Address |
[1] Add Customer [2] Show the customer details [3] Print list of all customers |
[3] CUSTOMER-RENT CHILD ADT |
|
Customer_ID ( Video_ID (of all rented videos of a customer) |
[1] Rent a Video (Add to the video ids rented) [2] Return a Video (Remove the video id from the list) [3] Print list of all videos rented by each customer
|
The program will require you to maintain 3 text files with specifications below:
Text File |
Requirements |
[1] VIDEO Text File |
[1] Will store the information about the Videos [2] Should contain the following by default: 5 Horror Movies 5 Romance Movies 5 Sci-Fi Movies 5 Action Movies 5 Comedy Movies **Must be authentic and true.** |
[2] CUSTOMER Text File |
[1] Will store the basic information about the Customers [2] Should contain at least 10 customers by default **Must be authentic and true.** |
[3] CUSTOMER-RENT |
[1] Will store all customers that RENT a VIDEO [2] Will store all the Video_IDs of all rented videos [3] Will delete Video_IDs and Customer_ID when videos are returned |
NOTE: IF YOU FIND THE NEED TO ADD OR MAKE ALTERATIONS TO THE SPECIFICATIONS OF THE ADT AND TEXT FILES ABOVE, PLEASE DO SO. JUST MAKE SURE THAT YOU HAVE AN ACCEPTABLE LOGICAL REASON.
INSTRUCTIONS:
- The program will have the following options/menus:
- [1] New Video //Programmer1
- [2] Rent a Video //Lead Programmer
- [3] Return a Video //Lead Programmer
- [4] Show Video Details //Programmer2
- [5] Display all Videos //Programmer1
- [6] Check Video Availability //Programmer2
- [7] Customer Maintenance
- [1] Add New Customer //Programmer1
- [2] Show Customer Details //Programmer2
- [3] List of Videos Rented by a Customer //Lead Programmer
- [8] Exit Program //Programmer1
- Follow the suggested screen dialogs below for each of the option above:
OTHER REQUIREMENTS:
- You must store your videos in a linked list when you retrieved them from the text file. They must also be stored in a linked list data structure during processing. Saving back to the text file will be done when the user chooses [8] Exit Program.
- You must store you customers in a queue when you retrieved them from the text file. They must also be stored in a queue during processing. Saving back to the text file will be done when the user chooses [8] Exit Program.
- Rented videos will be stored in a stack and will be saved in the CUSTOMER-RENT text file when the user chooses [8] Exit Program.
- Use data structures, files, functions, ADTs, STLs and
algorithms in your program. - Put necessary comments to your program.
- Provide error messages whenever necessary.
![[1] NEW VIDEO
Video ID:
Movie Title:
Genre:
Production:
Sci-Fi
Marvel Studios
10
auto-generated
Avengers: Endgame user input
user input
user input
Juser input
Number of Copies:
[4] SHOW VIDEO DETAILS
Alita: Battle Angel
Sci-Fi
Starwars: The Rise
Video ID:
of Skywalker
user input
automatically appears
automatically oppears
automatically oppears
outomatically appears
[2] RENT A VIDEO
Movie Title:
IGenre:
user input
automatically appears
Avengers: Endgame
Sci-Fi
Marvel Studios
10
Customer ID:
Name:
1
Resty Aldana
Gen. Trias, Cavite Jautomatically appears
Production:
Number of Copies:
Address:
[5] DISPLAY ALL VIDEOS
Videos to Rent.
Movie Title
Avengers: Endgame
Production
IMarvel Studios
Video ID
Genre
Video ID:
Movie Title
1
user input
Avengers: Endgame automatically appears
Jautomatically appears
1
Sci-Fi
Press Enter> to see more.
Number of Copies
[6] CHECK VIDEO AVAILABILTIY
Video ID:
Movie Title:
user input
Avengers: Endgame automatically appears
Rent another Video? Y
Video ID:
Movie Title:
2
Captain Marvel
user input
automatically appears
automatically appears
automatically appears
automatically appears
automatically appears
Genre:
Sci-Fi
Production:
Number of Copies: 10
Availability:
Marvel Studios
Number of Copies
2
Captain Marvel
Sci-Fi
Marvel Studios
| Available
automatically appears
Rent another Video? N
[7] CUSTOMER MAINTENANCE
Press <Enter to continue.
[1] Add New Customer
[3] RETURN A VIDEO
[2] Show Customer Details
[3] List of Videos Rented by a Customer
Enter Choice:
Customer ID:
1
Resty Aldana
Gen. Trias, Cavite automaticaily appears
user input
Godzilla
Sci-Fi
Name:
Address:
automatically appears
[7-1] ADD NEW CUSTOMER
Videos Rented.
Video ID: 1
Customer ID:
Name:
Address:
Resty Aldana
Gen. Trias, Cavite
auto-generated
user input
Juser input
Video ID: 2
Alita: Battle Angel
Sci-Fi
20th Century Fox
Videos successfully returned!
Press <Enter to continue.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5c92d0ee-427c-4349-9947-c58c2801c63c%2Fb40525cb-a05f-4ee7-ac83-c7434510dd03%2Fbjgo8q8_processed.jpeg&w=3840&q=75)
![[7-2] SHOW CUSTOMER DETAILS
Customer ID:
Name:
Address:
1
Resty Aldana
Gen. Trias, Cavite
user input
automatically appears
automatically appears
[7-3] LIST ALL VIDEOS RENTED BY A CUSTOMER
Customer ID:
Name:
Address:
1
user input
Resty Aldana
Gen. Trias, Cavite
Jautomatically appears
Jautomatically appears
List of Videos Rented..
Video ID
Movie Title
Avengers: Endgame
Captain Marvel
automatically appears
|automatically appears
2.
Press <Enter> to continue.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5c92d0ee-427c-4349-9947-c58c2801c63c%2Fb40525cb-a05f-4ee7-ac83-c7434510dd03%2Fuv39t2_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

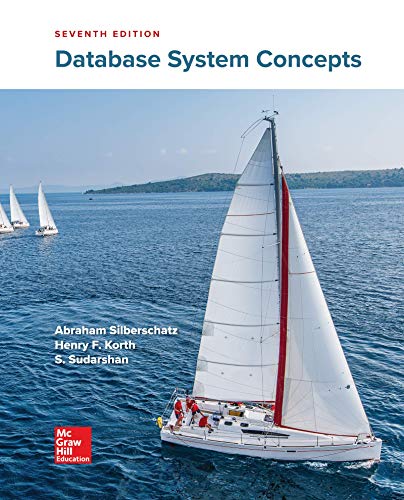
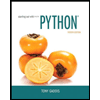
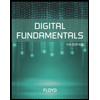
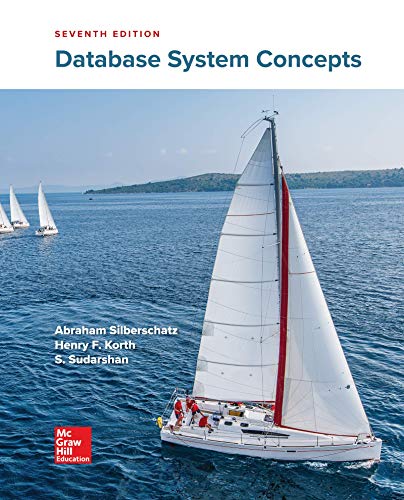
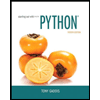
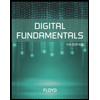
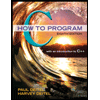
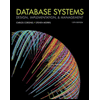
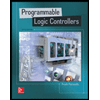