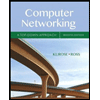
Unit 4 Debugging Exercises
The following 2 debugging assignments begins with some comments (lines that begin with 2 slashes) that describes the program. Examine the pseudocode that follows the introductory comments. Discover the errors and fix the pseudocode in the area labeled, Correct the pseudocode below.
Pseudocode Debugging Exercise 1
// This pseudocode should create a report that contains an apartment complex rental agent's commission.
// The program accepts the ID number and name of the agent who rented the apartment,
// and the number of bedrooms in the apartment.
// The commission is $100 for renting a three-bedroom apartment, $75 for renting a two-bedroom apartment,
// $55 for renting a one-bedroom apartment, and $30 for renting a studio (zero-bedroom) apartment.
// Output is the salesperson’s name and ID number and the commission earned on the rental.
start
Declarations
num salesPersonID
string salesPersonName
num numBedrooms
num COMM_3 = $100.00
num COMM_2 = $75.00
num COMM_1 = $55.00
num COMM_STUDIO = $30.00
num QUIT = 9999
getReady()
while salesPersonID <> QUIT
detailLoop()
endwhile
finish()
stop
getReady()
output "Enter salesperson ID or ", QUIT, " to quit "
output salesperson_ID
return
detailLoop()
output "Enter name "
input salesPersonName
output "Enter number of bedrooms rented "
input numBedrooms
if numBedrooms > 3 then
commissionEarned = COMM_3
else
if numBedrooms < 2 then
commissionEarned = COMM_2
else
if numBedrooms > 1 then
commission = COMM_1
else
commission = COMM_4
endif
endif
endif
output salesPersonID, salesPersName, commissionEarned
output "Enter salesperson ID or ", QUIT, " to quit "
input salesPersonID
return
finish()
output "End of report"
return

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Assignment details: Replace all the 0 (Zero) digits in your ID by 4. Example: If your ID is 38104680, it becomes 38144684 Take the first 6 digits and substitute them in this expression (( A + B) / C) * ((D-E)/F)-2) according to the following table; Letter Replace by Digit Example Digit A 1st 3 B 2nd 8 C 3rd 1 D 4th 4 E 5th 4 F 6th 6 After substitution your expression will be similar to this (( 3 + 8) / 1) * ((4-4)/6)-2). Draw a rooted tree that represents your expression. [3 marks] What is the prefix form of this expression. What is the value of the prefix expression obtained in step 2 above?arrow_forwardComputer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forwardLAB ASSIGNMENTS IMPORTANT: you should complete the PDP thinking process for each program. Turn in items: 1) fullname_regex.py - Ask the user to enter the source text to search, such as the name_source variable here: name_source = input('Enter full name in this format - first middle last'). Then, you can adapt the first two code lines from lesson slide 13, to search this new source. Edit the code to match the new situation and change the regex pattern to identify text that could be a full name. Hints: Initially write your regex pattern to check if the user enters three words separated by a space. Then, strive to make the regex adaptable if the person's full name has more than or less than 3 words. Then, think about allowing (not requiring) common characters like a period, hyphen or '. Slides 11-13 should be helpful. 08 For printing, end with a conditional block that provides an appropriate message if there is a match or not. Match Enter your full name: first middle last Betty Lou Who…arrow_forward
- 16 Type the correct answer in the box. Use numerals instead of words. If necessary, use / for the fraction bar. var num2 = 32; var num1 = 12; var rem=num2 % num1; while(rem> 0) { num2 = num1; num1 = rem; rem =num2 9% num1; document.write(num1): The output of the document.write statement at the end of this block is Reset Next m. All rights reserved. 5896arrow_forwardWeather Statistics": Problem Statement: Write a program that uses a structure to store the following weather data for a particular month: Total Rainfall High Temperature Low Temperature Average Temperature The program should have an array of 12 structures to hold weather data for an entire year. When the program runs, it should ask the user to enter data for each month. (The average temperature should be calculated.) Once the data are entered for all the months, the program should calculate and display the average monthly rainfall, the total rainfall for the year, the highest and lowest temperatures for the year (and the months they occurred in), and the average of all the monthly average temperatures. Input Validation: Only accept temperatures within the range between -100 and +140 degrees Fahrenheit.arrow_forwardSlappy’s Software Sales AssignmentYou have been hired by Slappy’s Software Sales to write a program to figure thetotal cost to customers that order Slappy’s software packages.Discounts are given based on the number of units sold:Number of Units Sold Discount Amount1 – 9 None10 – 19 20%20 – 49 30%50 – 99 40%100 or more 50%Create a form similar to this:The three boxes are labels. Remember to use the Auto Size and Border Styleproperties.The program should read the number of units sold and figure a base cost. Theunits cost $99 each. A discount should then be figured and subtracted from thebase cost. The subtotal, discount amount and total cost should be displayed, ascurrency, in the appropriate labels.Here are some screen shots of the running program:Set the tab order and access keys.Put comments in your program as to what each section is doing.Use constants for the discount rates and base price.arrow_forward
- Given code line, std::string un_address = "405 East 42nd Street, New York, NY, 10017"; Fill in the blanks: 17 std::stoi(un_address) returns std::stoi (un_address.substr( std::stoi (un_address.substr(un_address.find("1"))) J 20)) returns 42 returnsarrow_forwardPhython assignment Exercise 1: Oreo cookies Check out the nutritional values of an Oreo cookie (Koppelingen naar een externe site.). Write code that does the following: calculate how much one Oreo cookie is concerning: calories, sodium, carbohydrate, fat create a user input that asks how much cookies you ate calculate how much calories, etc. you consumed warn the user that if he/she surpasses 2000kcal he/she should stop eating these darn delicious cookies use variables!arrow_forwardPEN and PAPER Debugging the Pseudocode. Hand write the correct pseudocode using pen and paper. for DEBUG03-02.txt: // This pseudocode is intended to display employee net pay values.// All employees have a standard $45 deduction from their checks.// If an employee does not earn enough to cover the deduction,// an error message is displayed.start Declarations string name num hours num rate string DEDUCTION = 45 string EOFNAME = "ZZZ" num gross num net output "Enter first name or ", EOFNAME, " to quit" input name if name not equal to EOFNAME output "Enter hours worked for ", name input hours output "Enter hourly rate for ", name input rate gross = hours * rate net = gross - DEDUCTION while net > 0 then output "Net pay for ", name, " is ", net else output "Deductions not covered. Net is 0." endwhile output "Enter next name or ", EOFNAME, " to quit" input name endif…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
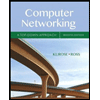
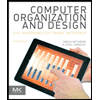
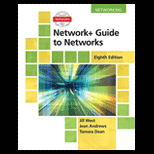
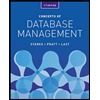
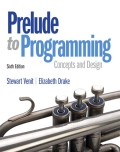
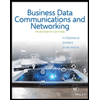