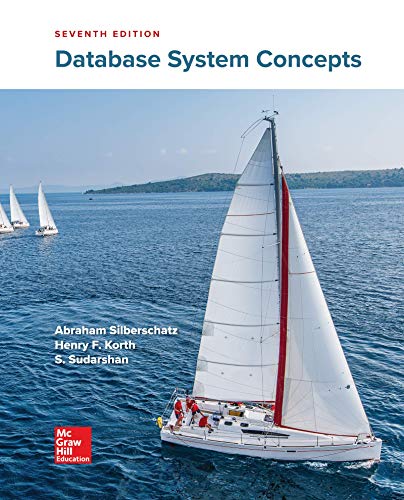
C++
Create class methods (Setters and Getters) that load the data values. The Setter methods will validate the input data based on the criteria below and load the data into the class if valid.
For character arrays, the data must be within the specified data size. (See #3 below)
For zipCode, the number must be between 0 and 99999.
City and State need to be converted and stored in uppercase.
To get started loading the c-strings, review table 10-3 from Week 5.
Getters
The book does not seem to provide a good example of what a (getter) method that returns a char array should look like. Section 9.9 is very close but uses string as examples instead of c-strings.
The best approach is to return a pointer to the c-string- Described as below:
char* getName(); // declaration - also known as prototype
// Customer is my Class name char* Customer::getName() { // defined member function - Return a pointer to the c-string
return name;
}
cout << YOUR_INSTANCE_HERE.getName() << endl;
Setters
As for creating a setter method - you can define unsized char arrays in the method header
bool Customer::setName(char inputName[]) {
// code here..
}
3. Write a program that will allow me to enter data for the class and create and call a method that displays the data for the class.
During the input process, pass the input data to the setter method and confirm that the data entered is valid based on the class method return value. If not valid,prompt for me to re-enter the data until it does pass the class method's validation.(A do-while loop works well for this situation) I only need to enter the data for 1 instance of the class. The best way to do this is for the setter methods to return bool instead of void. If the data is valid, return true. Otherwise return false.
There is no need to loop for multiple instances of the object. Do not use cin statements inside the class method definitions.
Below is the original structure to use to create your class definition.
const int NAME_SIZE = 20;
const int STREET_SIZE = 30;
const int CITY_SIZE = 20;
const int STATE_CODE_SIZE = 3;
struct Customer {
long customerNumber;
char name[NAME_SIZE];
char streetAddress_1[STREET_SIZE];
char streetAddress_2[STREET_SIZE];
char city[CITY_SIZE];
char state[STATE_CODE_SIZE];
int zipCode;};
Hints: The const variables need to be defined outside of the class definition.
All access to the data in the class must use public methods that you define. DO NOT DIRECTLY ACCESS the customer data items.
The class level input methods should return a boolean to confirm the data was loaded and passed any size or data ranges. The customerNumber setter does not need to return a value - It can be a void setter.
Set the customerNumber to 1 via the code - not user input. There is no input loop so only 1 set of customer's data is to be entered.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- in visual c#arrow_forwardProgramming Language: C++ Please use the resources included and provide notes for understanding. Thanks in advance. Code two functions to fill an array with the names of every World Series-winning team from 1903 to 2020, then output each World Series winner with the number of times the team won the championship as well as the years they won them. The input file is attached, along with the main function and screenprint. Please note team names that include two words, such as Red Sox, have an underscore in place of the space. This enables you to use the extraction operator with a single string variable. The following resources are included: Here is main. #include <iostream>#include <fstream>#include<string> using namespace std; // Add function declarations and documentation here void fill(string teams[], int size);void findWinner(string teams[], int size); int main(){ const int SIZE = 118;int lastIndex;string team[SIZE]; fill(team, SIZE);findWinner(team, SIZE); return…arrow_forwardC++ Use 2 D arrays String and float Make simplearrow_forward
- C++ Create a function that will read the following .txt file contents and put them into an array: "apple", "avocado", "apricot","banana", "blackberry", "blueberry", "boysenberry","cherry", "cantaloupe", "cranberry", "coconut", "cucumber", "currant","date", "durian", "dragonfruit","elderberry",arrow_forwardC++ Programming Code two functions to fill an array with the names of every World Series-winning team from 1903 to 2020, then output each World Series winner with the number of times the team won the championship as well as the years they won them. The input file is attached, along with the main function and screenprint. Please note team names that include two words, such as Red Sox, have an underscore in place of the space. This enables you to use the extraction operator with a single string variable. The following resources are included: Here is main. #include <iostream>#include <fstream>#include<string> using namespace std; // Add function declarations and documentation here void fill(string teams[], int size);void findWinner(string teams[], int size); int main(){ const int SIZE = 118;int lastIndex;string team[SIZE]; fill(team, SIZE);findWinner(team, SIZE); return 0;} // Add function definitions here WorldSeriesChampions.txt…arrow_forwardC++ Visual Studio 2019 Complete #7 Customer Accounts and #8 Search Function for Customer Accounts Program. Create the array with only 2 elements. 7.Customer Accounts Write a program that uses a structure to store the following data about a customer account: Name Address City, State, and ZIP Telephone Number Account Balance Date of Last Payment The program should use an array of at least 10 structures. It should let the user enter data into the array, change the contents of any element, and display all the data stored in the array. The program should have a menu-driven user interface. Input Validation: When the data for a new account is entered, be sure the user enters data for all the fields. No negative account balances should be entered. 8. Search Function for Customer Accounts Program Add a function to Programming Challenge 7 (Customer Accounts) that allows the user to search the structure array for a particular customer's account. It should accept part of the customer's name as an…arrow_forward
- C++ Write the program that outputs the following menu. The program should allow a user to input numbers into an array of integers. The array size should be 10. The first number should be stored in the zero position of the array. The next number should be stored in the next available location. Each option below should be written using a procedure, except Quit. Remember to comment the procedures with (given, task and return). (MENU) 1) Input number. 2) Sort numbers. 3) Output numbers 4) Quit Option 1) Allows the user to input a number and store it into the array. If the array is full no more numbers should be input. When the user select option 1 and the array is full it should output (Memory Full!). This option will only allow one number to be entered. If the user want to input another number they will need to select option 1 again. Option 2) This option should take the array and sort it in ascending order. Use the selection sort to do this. Option 3)…arrow_forwardASSIGNMENT-4 1. You have to create a memory size calculator in the C language that inputs the number of elements and the datatype of an array and prints the memory size(in Bytes) occupied by the array. User will enter 'i' for integer datatype, 'b’ for Boolean, 'c' for char, 'f for float and print "Invalid data type" for any other input.arrow_forwarduse c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forward
- Summary In this lab, you complete a partially prewritten C++ program that uses an array. The program prompts the user to interactively enter eight batting averages, which the program stores in an array. The program should then find the minimum and maximum batting average stored in the array as well as the average of the eight batting averages. The data file provided for this lab includes the input statement and some variable declarations. Comments are included in the file to help you write the remainder of the program. Instructions Ensure the source code file named BattingAverage.cpp is open in the code editor. Write the C++ statements as indicated by the comments. Execute the program by clicking the Run button. Enter the following batting averages: .299, .157, .242, .203, .198, .333, .270, .190. The minimum batting average should be .157, and the maximum batting average should be .333. The average should be .2365. I entered the question below and that was previously answered…arrow_forwardC++ Array Expander -Just do everything in main and make sure you comment each step Use Pointer Notation for the function and within the function. Use a main function and return the pointer from the ArrayExpander function to mainarrow_forwardFYI: Please write the code in Pseudocode (no programming language please) 1. Write a pseudocode that outputs the contents of parallel arrays. You do NOT have to write the entire program. The first array will hold phone numbers The second array will hold company names You do NOT need to load the arrays. Your code can assume they are already loaded with data. arrays are named phone[] and company[]arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
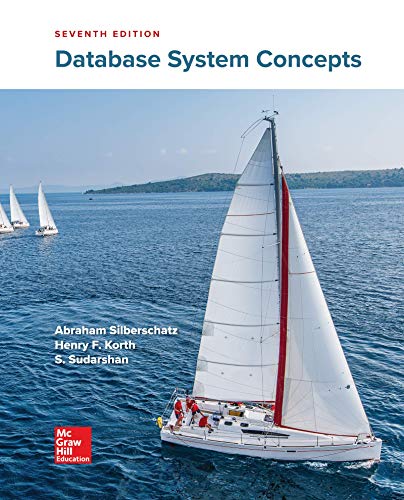
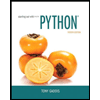
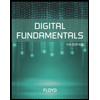
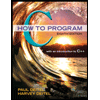
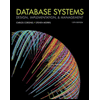
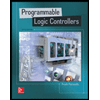